Create a struct to store the node label and its cost: struct Node { char label; int cost; }; SCS214: Data Structures Assignment-4 2- Implement a class MinHeap that has the following declaration: 3- Create a class WeightedGraph, which stores a graph using an adjacency matrix with the following declaration: class WeightedGraph { int** g; int nVertices; public: int getNVertices();//returns the number of vertices int getWeight(char,char);//returns weight of the edge connecting the given vertices int* returnNeighbors(int v);// returns the indices of the neighbors of the vertex v as an int array int numNeighbors(int v);//returns the number of neighbors of the vertex v void loadGraphFromFile(ifstream&);//allocates the adjacency matrix & initializes edge weights from the specified file void dijkstra(char startVertex, char* prev, Node distances[] );//find the shortest path from the start vertex to all other vertices, by filling the prev array and the distances array }; class MinHeap { Node* heap; //an array of nodes int _size; //size of array public: Node extractMin(); //returns & removes the node with minimum cost void buildMinHeap(Node[],int);// allocates array then builds a min-heap from an array of struct Node with the given size void minHeapify(int i, int n);//restores the min-heap property for the “heap” array using the given index and size n void decreaseKey(char label,int newCost);//decreases the node that has the given label to newCost int parent(int i);//returns the index of the parent of i int getSize();//returns size of the heap bool inHeap(char);//checks if the node with the given label is in the heap }; SCS214: Data Structures Assignment-4 int main() { WeightedGraph wg; ifstream ifile("graph.txt"); wg.loadGraphFromFile(ifile); char* p; p = new char[wg.getNVertices()]; Node* n; n=new Node[wg.getNVertices()]; wg.dijkstra('g',p,n); cout<
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
1- Create a struct to store the node label and its cost:
struct Node
{
char label;
int cost;
};
SCS214: Data Structures
Assignment-4
2- Implement a class MinHeap that has the following declaration:
3- Create a class WeightedGraph, which stores a graph using an adjacency matrix
with the following declaration:
class WeightedGraph
{
int** g;
int nVertices;
public:
int getNVertices();//returns the number of vertices
int getWeight(char,char);//returns weight of the edge connecting the given
vertices
int* returnNeighbors(int v);// returns the indices of the neighbors of the vertex
v as an int array
int numNeighbors(int v);//returns the number of neighbors of the vertex v
void loadGraphFromFile(ifstream&);//allocates the adjacency matrix & initializes
edge weights from the specified file
void dijkstra(char startVertex, char* prev, Node distances[] );//find the shortest
path from the start vertex to all other vertices, by filling the prev array and the
distances array
};
class MinHeap
{
Node* heap; //an array of nodes
int _size; //size of array
public:
Node extractMin(); //returns & removes the node with minimum cost
void buildMinHeap(Node[],int);// allocates array then builds a min-heap from an
array of struct Node with the given size
void minHeapify(int i, int n);//restores the min-heap property for the “heap”
array using the given index and size n
void decreaseKey(char label,int newCost);//decreases the node that has the given
label to newCost
int parent(int i);//returns the index of the parent of i
int getSize();//returns size of the heap
bool inHeap(char);//checks if the node with the given label is in the heap
};
SCS214: Data Structures
Assignment-4
int main()
{
WeightedGraph wg;
ifstream ifile("graph.txt");
wg.loadGraphFromFile(ifile);
char* p;
p = new char[wg.getNVertices()];
Node* n;
n=new Node[wg.getNVertices()];
wg.dijkstra('g',p,n);
cout<<endl<<"Node\tCost\tPrevious";
for(int i=0;i<wg.getNVertices();i++)
{
cout<<endl<<n[i].label<<"\t"<<n[i].cost<<"\t"<<p[i];
}
ifile.close();
return 0;
}
4- Your main function might look like this:
5- The file graph.txt will have the following format, where the
first line represents number of vertices and the second line
represents the number of edges. For each of the following
lines, each line has the label of the first vertex, then the label
of the second vertex, then the edge weight. Note that the
sample graph in this file is a directed graph.
6- For the sample graph in graph.txt, the output should be:
8
15
g a 9
g e 14
g f 15
a b 24
b d 2
b h 19
c b 6
c h 6
d c 11
d h 16
e b 18
e d 30
e f 5
f d 20
f h 44

Step by step
Solved in 2 steps

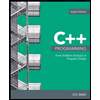
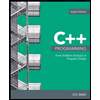