Create a flowchart and modify the code. INSTRUCTION: Create a new class called CalculatorWithMod. This class should be a sub class of the base class Calculator. This class should also have an additional method for calculating the modulo. The modulo method should only be seen at the sub class, and not the base class! Include exception handling for instances when dividing by 0 or calculating the modulo with 0. You would need to use throw, try, and catch. The modulo (or "modulus" or "mod") is the remainder after dividing one number by another. Example: 20 mod 3 equals 2 Because 20/3 = 6 with a remainder of 2 CODE TO COPY: #include using namespace std; class Calculator{ public: Calculator(){ printf("Welcome to my Calculator\n"); } int addition(int a, int b); int subtraction(int a, int b); int multiplication(int a, int b); float division(int a, int b); };
Create a flowchart and modify the code.
INSTRUCTION:
Create a new class called CalculatorWithMod. This class should be a sub class of the base class Calculator. This class should also have an additional method for calculating the modulo. The modulo method should only be seen at the sub class, and not the base class! Include exception handling for instances when dividing by 0 or calculating the modulo with 0. You would need to use throw, try, and catch. The modulo (or "modulus" or "mod") is the remainder after dividing one number by another.
Example: 20 mod 3 equals 2
Because 20/3 = 6 with a remainder of 2
CODE TO COPY:
#include <iostream>
using namespace std;
class Calculator{
public:
Calculator(){
printf("Welcome to my Calculator\n");
}
int addition(int a, int b);
int subtraction(int a, int b);
int multiplication(int a, int b);
float division(int a, int b);
};
int Calculator::addition(int a, int b){
return (a+b);
}
int Calculator::subtraction(int a, int b){
return (a-b);
}
int Calculator::multiplication(int a, int b){
return (a*b);
}
float Calculator::division(int a, int b){
return (a*1.0/b);
}
int main(){
Calculator myCalculator; //change the class name to CalculatorWithMod when you finish your modifications
int x, y;
printf("Input 1st integer: ");
scanf("%d",&x);
printf("Input 2nd integer: ");
scanf("%d",&y);
printf("The sum is %d \n", myCalculator.addition(x,y));
printf("The difference is %d \n", myCalculator.subtraction(x,y));
printf("The product is %d \n", myCalculator.multiplication(x,y));
printf("The quotient is %d \n", myCalculator.division(x,y));
return 0;
}
I have attached the sample output



Step by step
Solved in 4 steps with 4 images

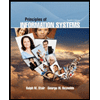
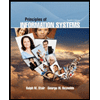