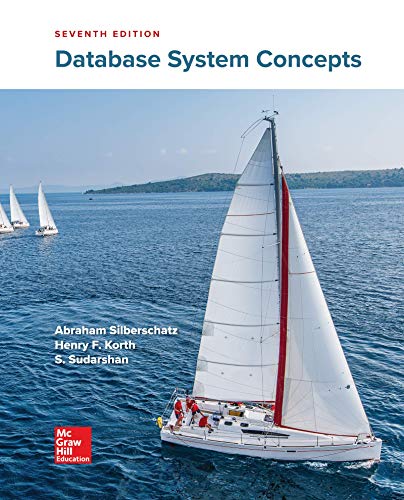
Please provide JAVA source code for following assignment. Please attach proper comments and read the full requirements.
The local Driver’s License Office has asked you to write a
-
B
-
D
-
A
-
A
-
C
-
A
-
B
-
A
-
C
-
D
-
B
-
C
-
D
-
A
-
D
-
C
-
C
-
D
-
D
-
A
A student must correctly answer 15 of the 20 questions to pass the exam.
Write a class named Drivertest that holds the correct answers to the test in an array field. The class should also have an array field that holds the student’s answers. The class should have the following methods:
-
passed. Returns true if the student passed the test, or false if the student failed
-
totalCorrect. Returns the total number of correctly answered questions
-
totalIncorrect. Returns the total number of incorrectly answered questions
-
questionsMissed. An int array containing the question numbers of the questions that the student missed
Demonstrate the class in a complete program that asks the user to enter a student’s answers, and then displays the results returned from the Drivertest class’s methods.
Input Validation: Only accept the letters A, B, C, or D as answers.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- A method’s __________ consists of the method’s name and the data type and argument kind (by value, ref, or out) of the method’s parameters, from left to right. a. appearance b. signature c. identifier d. footprintarrow_forwardYour program should ask for the input from the user. The input ends with 999 to indicate that there are no more classes to be scheduled. Please enter class details. 3203 100 1500 1103 100 1500 2201 300 800 2203 100 1500 3401 200 800 1201 100 1500 999 The output should be the following: 3401 starts at 800 ends at 1000 2201 starts at 1000 ends at 1300 3203 starts at 1500 ends at 1600 2203 starts at 1600 ends at 1700 1201 starts at 1700 ends at 1800 1103 starts at 1800 ends at 1900 To help you get started, you are given with a code snippet to write your code. #include <iostream> using namespace std; struct Course { int ccode; // course code int duration; // class duration in military hours, so 2 hours is 200 an so on int lecture_arrive_time; //prefered start time 800 for 8am and 1200 for 12pm }; void print_sched(Course c[],int); void class_sort(Course c[],int); int main() { int input=0,i=0; Course classes[100]; cout <<…arrow_forwardIn this assignment you will demonstrate your knowledge of debugging by fixing the errors you find in the program below. Fix the code, and paste it in this document, along with the list of the problems you fixed.This example allows the user to display the string for the day of the week. For example, if the user passed the integer 1, the method will return the string Sunday. If the user passed the integer 2, the method will return Monday. This code has both syntax errors and logic errors. Hint: There are two logic errors to find and fix (in addition to the syntax errors).Inport daysAndDates.DaysOfWeek;public class TestDaysOfWeek {public static void main(String[] args) {System.out.println("Days Of week: ");for (int i = 0;i < 8;i++) {System.out.println("Number: " + i + "\tDay Of Week: " + DaysOfWeek.DayOfWeekStr(i) )}}}package daysAndDatespublic class DaysOfWeek {public static String DayOfWeekStr(int NumberOfDay) {String dayStr = ""switch (NumberOfDay) {case 1:dayStr =…arrow_forward
- Which of the following statement is correct? Answer Choices: f. struct can be used as a base class for another class. enum can be used as a base class for another class Only a class can act as a base class for another class. h. None of the above. g.arrow_forwardQUESTION 13 2 Code only the header for a method that returns a message about the status of a tank of gas. The method monitors fuel. The method is parameterless. The name of the method is in the 2nd sentence, except the verb is non-plural. //Method header. Call the method by assigning it to a variable called msg. First declare the variable initialized to the proper data type. //Variable declaration. //Method call.arrow_forward#this is a python program #topic: OOP Design the Country class so that the code gives the expected output. [You are not allowed to change the code below] # Write your Class Code here country = Country() print('Name:',country.name) print('Continent:',country.continent) print('Capital:',country.capital) print('Fifa Ranking:',country.fifa_ranking) print('===================') country.name = “Belgium” country.continent = “Europe” country.capital = “Brussels” country.fifa_ranking = 1 print('Name:',country.name) print('Continent:',country.continent) print('Capital:',country.capital) print('Fifa Ranking:',country.fifa_ranking) Output: Name: Bangladesh Continent: Asia Capital: Dhaka Fifa Ranking: 187 =================== Name: Belgium Continent: Europe Capital: Brussels Fifa Ranking: 1arrow_forward
- IN C# PLEASE Create class Cube. The class has attributes length and width and depth, each of which defaults to 1. It has read-only properties that calculate the Perimeter and the Area for a side of the cube, and another that calculates the cubed feet for the cube. (You will have to research how to do that calculation online) It has properties for length and width and depth. The set accessors should verify that length and width and depth are all floating-point numbers greater than 0.0 and less than 20.0. Write an app to test class Cube by creating 3 cubes of different sizes.arrow_forwardFor c++. Need help designing class, no namespsace. FoodWastageRecord NOTE: You need to design this class. It represents each food wastage entry recorded by the user through the form on the webpage (frontend). If you notice the form on the webpage, you’ll see that each FoodWastageRecord will have the following as the data members aka member variables. Date (as string) Meal (as string) Food name (as string) Quantity in ounces (as double) Wastage reason (as string) Disposal mechanism (as string) Cost (as double) Each member variable comes with its accessor/mutator functions. --------------------------------------------------------------------------------- FoodWastageReport NOTE: You need to design this class. It represents the report generated on the basis of the records entered by the user. This class will be constructed with all the records entered by the user as a parameter. It will then apply the logic to go over all the records and compute the following: Names of most commonly…arrow_forwardExercise 5 - Number to Word Make a new class in the Lab2 project called Num2wordthat prompts the user enter a number between 0 and 9 and then displays the corresponding word (i.e. "zero"for 0, "one"for 1, etc.). Use a switchstatement to do this. Include a default case that lets the user know they entered a wrong number. The switch statement has the following syntax: switch (variable) { case value1: // Code that should execute when variable==value1 break; // this prevents it from automatically going to the next case case value2: // Code that should execute when variable==value2 break; // this prevents it from automatically going to the next case default: // Code that should execute when variable has a value that didn't match the above }arrow_forward
- Monthly Payment Program (in the attached photo), update it with the following in java code: If applicable, remove hard coding for the principal, annual interest rate and number of years. Replace these with Input Dialog Boxes requesting data be entered. The calculation should remain the same. Replace the output area with a showMessage box. Allow the user to request a detailed display of the payment schedule. This will include the ending balance and interest paid per month in a line-by-line display. Add a loop to the program allowing the user to continue entering new data as many times as they like (all input and output should be displayed in dialog boxes).arrow_forwardobject oriented programming need the full code as soon as possible.arrow_forwardProject: Two Formulas in Separate Class Write two formulas your choice in separate file and you call it in some manner from your from main program. In Java the pic for examplearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
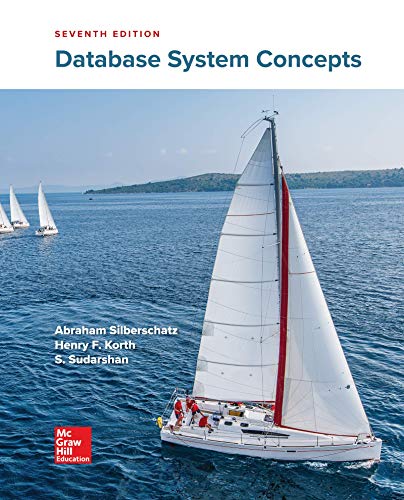
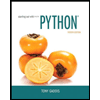
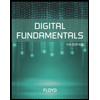
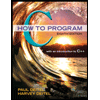
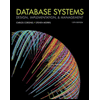
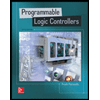