What is meant by exception and exception handling?
An exception is a situation or anomaly that occurs during the program execution which prevents the execution from continuing further. An exception is an unexpected behavior that can cause the program to stop abruptly.
Exception handling refers to mechanisms used to handle the exception when it occurs so that the program terminates gracefully. A try block is used to catch these exceptions, whereas a catch block might handle these exceptions which prevents the program or application from crashing.
Syntax
try
{
// try block – track the exception
}
catch (exception e)
{
//catch block – handles the exception
}
finally
{
// finally block – execute always
}
When an exception occurs?
An exception occurs for various reasons; most of the exceptions are due to programming errors, user errors, or physical resources which are failed. The following are some situations where exceptions will occur:
- When there is necessity to open a file and that file is not found.
- When the user enters an invalid information/data.
- Whenever the network connection is lost at the middle of communication.
- When there is a lack of memory space in JVM (Java Virtual Mechine).
- When accessing the null object member.
Based on these scenarios, the exception is divided into 3 different categories and they are:
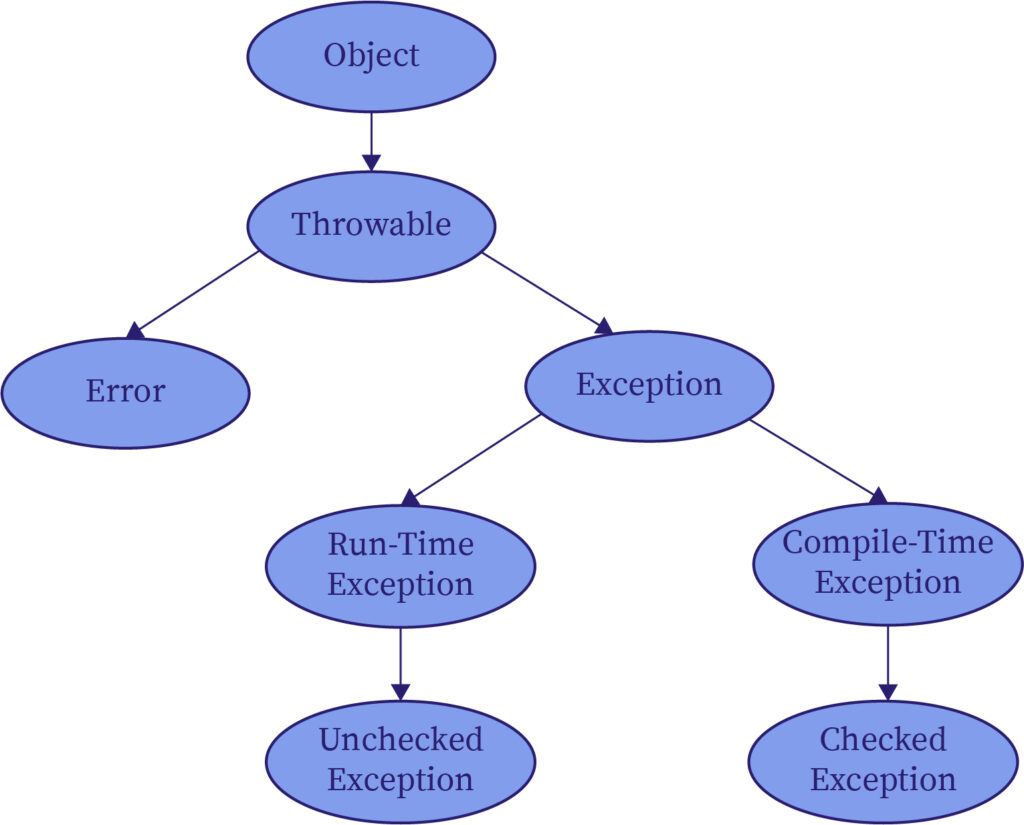
Checked exception
The checked exception is checked/notified during the compile-time and it is known as a compile-time exception. An exception will directly inherit the throwable exception except for the runtime and error class Exception.
Unchecked exception
The unchecked exception occurs during the execution time and is known as a runtime exception. The class which inherits the RuntimeException is used to deal with unchecked exception.
Errors
Errors occur when the system does not behave as expected and it belongs to runtime exception category.
Exception hierarchy
Exception classes are the subtypes of java.lang.Exception class. Exception class is the subclass of Throwable class. Error is another subclass apart from the Exception class which is also derived from the Throwable class. The two subclasses of exception class are:
- IOException class.
- RuntimeException class.
Types of exception
In Java programming, the exceptions are divided into two types namely:
User-defined exception
The other name for the user-defined exception is a custom exception. The user can create the Exception class and the exceptions are thrown using the keyword throw. This can be done by just extending the Exception class. These exceptions are known as compile-time or checked exceptions.
Built-in exception
These exceptions are caught, by using the Java libraries. It is also known as runtime or unchecked exceptions. The following are the built-in exception types and they are:
- ArithmeticException: The arithmeticException occurs when there is a mismatch during arithmetic computation.
- ClassNotFoundException: The ClassNotFoundException occurs when there is no proper definition for the class.
- IOException: The IOException occurs when either the output or input gets terminated and the operation is failed.
- ArrayIndexOutOfBoundsException: The ArrayIndexOutOfBoundsException occurs when the index is accessed wrongly, when index range is unreachable, and when the index cannot be accessed.
- FileNotFoundException: The FileNotFoundException occurs when the file path is not properly mentioned or when the files does not open.
- NullPointerException: The NullPointerException occurs when the member of the object refers or points to null values.
- NoSuchFieldException: The NoSuchFieldException occurs when there is no variable or field present.
- NoSuchMethodException: The NoSuchMethodException occurs while accessing the method which is missing or not defined properly.
- StringIndexOutOfBoundsException: The StringIndexOutOfBoundsException occurs when the range of index is in negative or it is greater than the defined range at the string class.
- RuntimeException: The RuntimeException occurs during the runtime.
Example: Program for ArithmeticException
Public class ArithmeticException1
{
public static void main(String[] args)
{
try { //try block
int a = 0;
int b = 20;
int c = 0;
int d = (a-b)/c;
System.out.println("n Result: " + d );
}
catch(ArithmeticException arith_ex)
{ //catch block
System.out.println ("n The first number which is multiplied by the second number cannot be stored at third number ");
}
}
}
Exception handling in detail
Exception handling is a procedure that handles the runtime errors and allows the user to take necessary action in order to avoid these runtime errors. The exception handling operations are done during the program runtime. The exception handling can be done at the programmer’s side with the help of the keywords of exception handling.
Flow of control during exception handling
The following are the control flow of the exception handling:
- The exceptions are handled by the catch block. The catch block can throw an exception and also rethrow the exception that is caught.
- When an exception occurs at try block, the corresponding catch block gets executed and the statements that are present at the try block after the statement that had thrown exception will not get executed. But, the finally block will get executed.
- The finally block will get executed, even though the try block has a return statement. After executing the finally block the control will move to try block and then to the return statement.
Exception handler
The core feature which makes Java robust is the exception handler framework. When an exception occurs, it constructs an exception object with the help of JVM or a method that executes the code. The object holds the data about the exception. Thus, the exception handler is a method of handling the exception object.
Exception handling keywords
Exception handling allows the user to take necessary actions in order to avoid runtime errors. Java has five keywords that support exception handling; these keywords are reserved words that cannot be changed. With the help of these keywords, the user can easily control the exception that occurs during the runtime and the keywords are:
Try
The try block is created with the help of the try keyword followed by open and close braces. Try block has a bunch of statements with certain exception rules to throw whenever an exception occurs. If some exception is occurred during the program execution and matches one of the try blocks; then the try gets an exception and transfers the control to the corresponding catch block and the catch block might handle those errors. Try block cannot be used as a standalone block and it should have either a catch block or finally block.
Catch
The catch block is created with the help of the catch keyword followed by open and close braces. The catch block will catch the exception that is transferred from the try block and just displays the information to the programmer to overcome an exception. Catch block cannot be used as a standalone block and it should be preceded by try block; however, finally block is optional.
Finally
The finally block is created with the help of finally keyword followed by open and close braces. This block is an optional one; whereas it may/may not be used in the try-catch block. As per the syntax of exception handling this block is at the end of each try-catch block. Whether an exception occurs or not this block gets executed; as it contains all the important codes that need to be executed.
Throw
To throw an exception the throw keyword is used. To throw a custom exception, the user needs to a create custom exception and then it is thrown using the keyword throw.
Throws
The exception is declared with the help of the throws keyword. This keyword shows that there are possibilities for exceptions in the method.
Benefits of exception handling
- The flow of program is maintained with the help of exception handling.
- Group and differentiate the error types.
- Separates the error-handling code from other code.
- Exception act as a termination statement.
- Exception handling makes the error, user-friendly.
Context and Applications
This topic is important for postgraduate and undergraduate courses, particularly for, Bachelors in Computer Science Engineering, and Associate of Science in Computer Science.
Practice problems
Question 1: Which one of the following is not a keyword of exception handling?
a) try
b) throw
c) finally
d) thrown
Answer: Option d is correct.
Explanation: An exception is an unexpected behavior that helps the program to break the application and exception handling uses five keywords such as – try, finally, catch, throws, and throw.
Question 2: Exception handling can group and differentiate the error type.
a) True
b) False
c) Maybe true
d) None of the above
Answer: Option a is correct.
Explanation: Generally, the exception happens for various reasons and they occur due to physical resources, programming errors, or even user errors. thus, exception handling helps to group and differentiate the error type.
Question 3: In code sequence, an exception arises during __________ time.
a)Compile time or run time
b) Compile time
c) Runtime
d) None of the above
Answer: Option a is correct.
Explanation: An exception is a situation that happens during the program flow to prevent the program from continuing. They can occur during compile-time or runtime.
Question 4: Which of the following keyword is used to check for exceptions?
a) try
b) throw
c) finally
d) catch
Answer: Option a is correct.
Explanation: Try block is a block with certain exception rules to throw when an exception occurs. The keyword try is used to create a try block and try block checks all the exceptions.
Question 5: From the below options, select the three, which can be thrown by using the throw statement.
- Throwable
- RuntimeException
- Error
- Object
- Event
a) 1, 3, 5
b) 1, 2, 3
c) 1, 2, 4
d) 2, 4, 5
Answer: Option b is correct.
Explanation: Throwable acts as an error’s superclass and exception at Java. During normal operations, RuntimeException act as an exception superclass. An error will be the subclass of all throwable.
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Exception Handling Keywords Homework Questions from Fellow Students
Browse our recently answered Exception Handling Keywords homework questions.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.