Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used. ** MUST use given Template below: #ifndef avltree_h #define avltree_h #include template class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const { eturn bf; } friendclassAVLTree; }; private: std::unique_ptr root_; int size_; public: AVLTree() : size_(0) {} // TODO: Develop a code to update the balance factor and rebalance Value&operator[](constKey&key) { // Try to find the node with the value we want: std::unique_ptr *cur; for (cur = &root_; cur->get() != nullptr; cur = key < (*cur)->k ? &(*cur)->left_ : &(*cur)->right_) { if (key == (*cur)->k) { return (*cur)->v; } } // If we did not find it, then insert a new node with that key: // (This is the same behaviour as an std::map.) cur->reset(newNode(key)); ++size_; return (*cur)->v; } intsize() { returnsize_; } Node *root() { return root_.get(); } }; #endif
Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used. ** MUST use given Template below: #ifndef avltree_h #define avltree_h #include template class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const { eturn bf; } friendclassAVLTree; }; private: std::unique_ptr root_; int size_; public: AVLTree() : size_(0) {} // TODO: Develop a code to update the balance factor and rebalance Value&operator[](constKey&key) { // Try to find the node with the value we want: std::unique_ptr *cur; for (cur = &root_; cur->get() != nullptr; cur = key < (*cur)->k ? &(*cur)->left_ : &(*cur)->right_) { if (key == (*cur)->k) { return (*cur)->v; } } // If we did not find it, then insert a new node with that key: // (This is the same behaviour as an std::map.) cur->reset(newNode(key)); ++size_; return (*cur)->v; } intsize() { returnsize_; } Node *root() { return root_.get(); } }; #endif
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C++ Data Structure:
Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map.
In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used.
** MUST use given Template below:
#ifndef avltree_h
#define avltree_h
#define avltree_h
#include <memory>
template <typename Key, typename Value=Key>
class AVL_Tree {
public:
classNode {
private:
Key k;
Value v;
int bf; //balace factor
std::unique_ptr<Node> left_, right_;
Node(const Key& key) : k(key), bf(0) {}
Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {}
public:
Node *left() {
return left_.get();
}
Node *right() {
return right_.get();
}
const Key& key() const {
return k;
}
const Value& value() const {
return v;
}
const int balance_factor() const {
eturn bf;
}
friendclassAVLTree<Key, Value>;
};
private:
std::unique_ptr<Node> root_;
int size_;
public:
AVLTree() : size_(0) {}
// TODO: Develop a code to update the balance factor and rebalance
Value&operator[](constKey&key) {
// Try to find the node with the value we want:
std::unique_ptr<Node> *cur;
for (cur = &root_;
cur->get() != nullptr;
cur = key < (*cur)->k ? &(*cur)->left_ : &(*cur)->right_) {
if (key == (*cur)->k) {
return (*cur)->v;
}
}
// If we did not find it, then insert a new node with that key:
// (This is the same behaviour as an std::map.)
cur->reset(newNode(key));
++size_;
return (*cur)->v;
}
intsize() {
returnsize_;
}
Node *root() {
return root_.get();
}
};
#endif
#endif
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
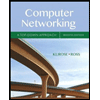
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
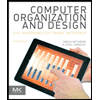
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
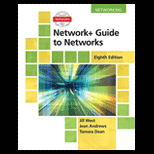
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
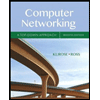
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
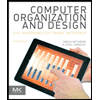
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
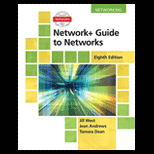
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
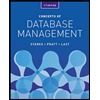
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
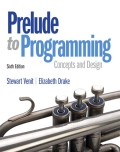
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
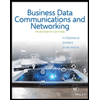
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY