Create an Eclipse Java project that reads in the names and postal (Zip) code data for individuals from the attached "employee-2.txt Download employee-2.txt" file (note: you need to add the text file with the exact file name to the root "project folder" of your Eclipse Java project, not the "src" folder). In the attached text file, edit the last row manually to add your own First Name, Last Name and Zip code. Your project should read in the employee data provided in the text file and store the data into a LinkedList data structure, for which, you need to write a Java class named "Employee.java" that defines a first name (type String), a last name (type String), a postal code (type int or String), an Employee "constructor" and a toString() method. You may optionally define "setters" and "getters" methods in the "Employee.java" class. You should write a separate driver class named "EmployeeListDriver.java" to implement the main() method, the main() method is where you should create the LinkedList and all related operations. Your project should have the following two Java files in the "src" folder, and the given text file inside the root (project) folder. Employee.java EmployeeListDriver.java In the attached text file, each line contains two strings followed by an integer value, each separated by a tab character. Each line of data in the attached text file should be read in to "construct" an Employee object as defined in your Java class "Employee.java", each constructed Employee object should then be added to the LinkedList. After all the data from the text file have been read in and stored in LinkedList object, re-access the LinkedList<> to print all the Employee object's First Name, Last Name and Zip Code in an appropriate format on the screen.
Create an Eclipse Java project that reads in the names and postal (Zip) code data for individuals from the attached "employee-2.txt Download employee-2.txt" file (note: you need to add the text file with the exact file name to the root "project folder" of your Eclipse Java project, not the "src" folder). In the attached text file, edit the last row manually to add your own First Name, Last Name and Zip code.
Your project should read in the employee data provided in the text file and store the data into a LinkedList<Employee> data structure, for which, you need to write a Java class named "Employee.java" that defines a first name (type String), a last name (type String), a postal code (type int or String), an Employee "constructor" and a toString() method. You may optionally define "setters" and "getters" methods in the "Employee.java" class.
You should write a separate driver class named "EmployeeListDriver.java" to implement the main() method, the main() method is where you should create the LinkedList<Employee> and all related operations.
Your project should have the following two Java files in the "src" folder, and the given text file inside the root (project) folder.
- Employee.java
- EmployeeListDriver.java
In the attached text file, each line contains two strings followed by an integer value, each separated by a tab character. Each line of data in the attached text file should be read in to "construct" an Employee object as defined in your Java class "Employee.java", each constructed Employee object should then be added to the LinkedList<Employee>. After all the data from the text file have been read in and stored in LinkedList<Employee> object, re-access the LinkedList<> to print all the Employee object's First Name, Last Name and Zip Code in an appropriate format on the screen.
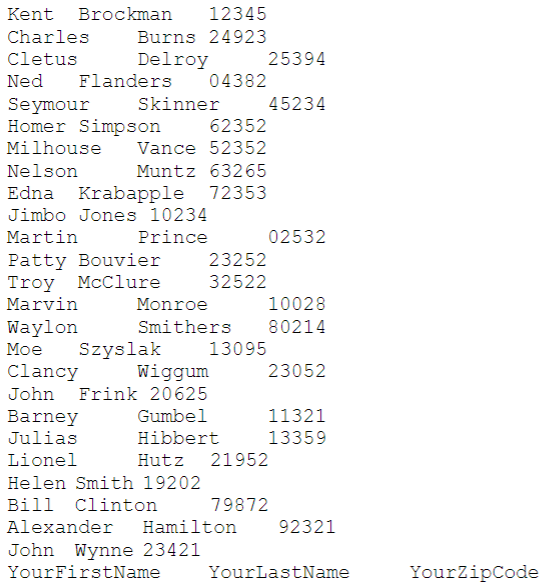
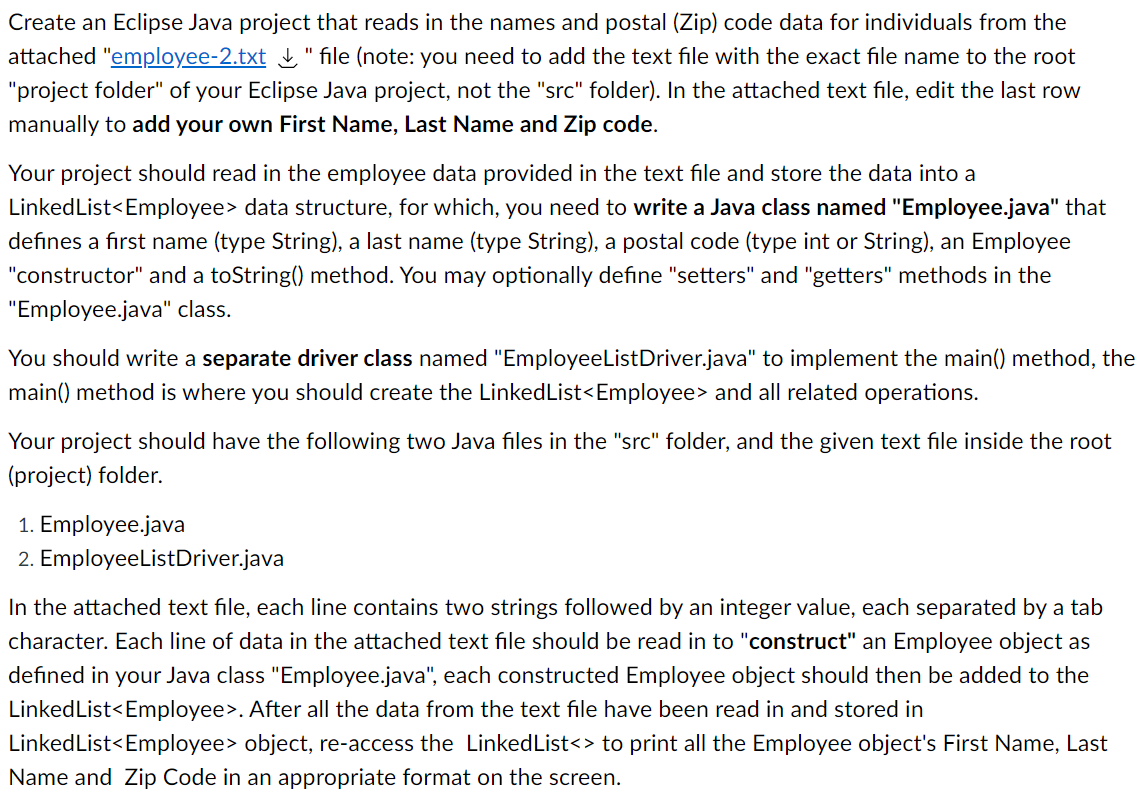

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

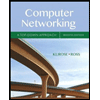
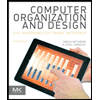
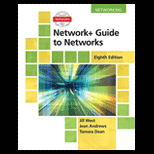
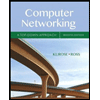
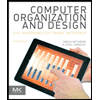
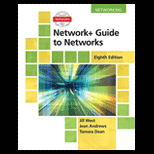
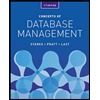
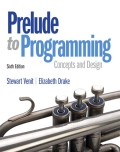
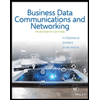