Using PyCharm, create a new Project lab12. Under lab12 create a new Directory exercise,then create a Python file called name.py in which to writea class named Name. This class holds the entire name of a person, that is, first, middle and last names and a suffix, and keeps count of how many of these names are being used (this is explained below). 1. The class has the following fields: count, first, middle, last and suffix. 2. The class has a constructor that receives one string parameter named name whose default value is an empty string (that is, calling the constructor with no parameters sets the value of name to an empty string). The constructor initializes all fields based on the value of name, as explained next: – The value of count depends on the number of words in name. For example, given the name Robert John Downey Jr then count is 4. – If name has one word then it has a first name. – If name has two words then it has a first and last names in that order. – If name has three words then it has a first, middle and last names in that order. – If name has four words then it has a first, middle, last names and suffix in that order. Assume that name will never have more than 4 names; that is, it will have one of the following formats exactly: – first – first last – first middle last – first middle last suffix Hint: Use split( ) to get an array of words. 3. The class has getter and setter methods for fields first, middle, last and suffix. Each setter should adjust (as needed) the value of count each time a name is given or made empty. That is, if the name is empty and is set to a non-empty value then count is incremented. If the name is not empty and is set to an empty value then count is decremented. Note that count is not modified if the name has a value and is set to another value, or if it is empty and is set to an empty value (which is kind of pointless, really, but can happen). For example, if the middle name is empty and we call method set_middle("Bob") then the middle name changes to "Bob" and count is incremented. No other fields should change. The methods to implement are: – set_first(self, value ): sets the value of first. – get_first(self): returns the value of first. – set_middle(self, value ): sets the value of middle. – get_middle(self): returns the value of middle. – set_last(self, value ): sets the value of last. – get_last(self): returns the value of last. – set_suffix(self, value ): sets the value of suffix. – get_suffix(self): returns the value of suffix. 4. The class has the following additional methods – get_count(self): returns the value of count. – get_name(self): returns the concatenation of first + " " + middle + " " + last + " " + suffix with only one space between names as appropriate. For example, if middle name is empty then the method returns first + " " + last + " " + suffix with one space in between. – get_initials(self): returns the concatenation of the first letter in first + middle + last if each respective name exists. For example, if there is no middle name, then it returns the initials of the first and last name
Using PyCharm, create a new Project lab12. Under lab12 create a new Directory exercise,then create a Python file called name.py in which to writea class named Name. This class holds the entire name of a person, that is, first, middle and last names and a suffix, and keeps count of how many of these names are being used (this is explained below).
1. The class has the following fields: count, first, middle, last and suffix.
2. The class has a constructor that receives one string parameter named name whose default value is an empty string (that is, calling the constructor with no parameters sets the value of name to an empty string).
The constructor initializes all fields based on the value of name, as explained next:
– The value of count depends on the number of words in name. For example,
given the name Robert John Downey Jr then count is 4.
– If name has one word then it has a first name.
– If name has two words then it has a first and last names in that order.
– If name has three words then it has a first, middle and last names in that order.
– If name has four words then it has a first, middle, last names and suffix in that order.
Assume that name will never have more than 4 names; that is, it will have one of the
following formats exactly:
– first
– first last
– first middle last
– first middle last suffix
Hint: Use split( ) to get an array of words.
3. The class has getter and setter methods for fields first, middle, last and suffix. Each setter should adjust (as needed) the value of count each time a name is given or made empty. That is, if the name is empty and is set to a non-empty value then count is incremented. If the name is not empty and is set to an empty value then count is decremented. Note that count is not modified if the name has a value and is set to another value, or if it is empty and is set to an empty value (which is kind of pointless, really, but can happen). For example, if the middle name is empty and we call method
set_middle("Bob") then the middle name changes to "Bob" and count is incremented. No other fields should change.
The methods to implement are:
– set_first(self, value ): sets the value of first.
– get_first(self): returns the value of first.
– set_middle(self, value ): sets the value of middle.
– get_middle(self): returns the value of middle.
– set_last(self, value ): sets the value of last.
– get_last(self): returns the value of last.
– set_suffix(self, value ): sets the value of suffix.
– get_suffix(self): returns the value of suffix.
4. The class has the following additional methods
– get_count(self): returns the value of count.
– get_name(self): returns the concatenation of first + " " + middle + " " + last + " " + suffix with only one space between names as appropriate. For example, if middle name is empty then the method returns first + " " + last + " " + suffix with one space in between.
– get_initials(self): returns the concatenation of the first letter in first +
middle + last if each respective name exists. For example, if there is no middle
name, then it returns the initials of the first and last name

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

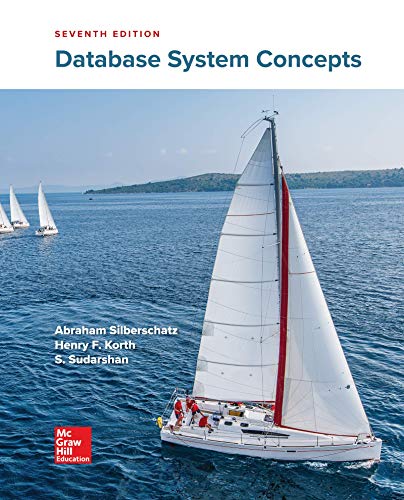
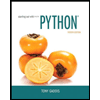
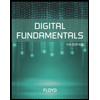
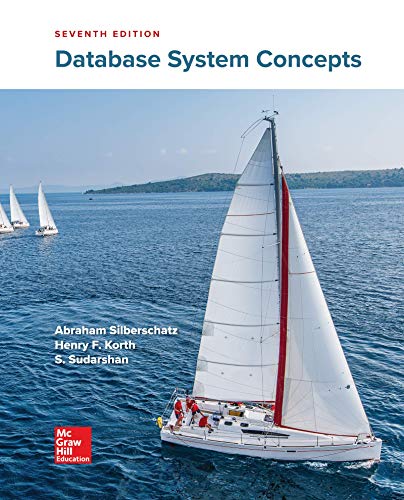
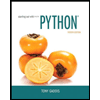
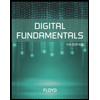
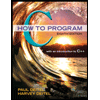
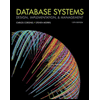
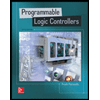