create classes to manage CD and publisher and expand the classes so that you can handle book and DVD. This should be done with the help of inheritance. A general superclass, Media, will be created to be able to reuse common data and functionality between the CD, Book and DVD classes. These 3 classes must thus inherit their properties from the Media class. The Media class is a superclass for different type of media. you will collect instance variables and methods that are common to all type of media. All instance variables in the class are declared as protected, which mean that classes that inherit from Media have a direct access to these variables.The class only needs 2 constructors. One takes 3 argument that set values for all instance variables in the class, and the other takes no arguments but sets certain default values of the instance variables. If you think that more designers are necessary, you can add. In the setLength method, perform a check so that the value submitted is not less than zero. It should also not be possible to create a Media object whose length is less than 0. There is no point in setting the length of a media to -1. If the value sent to the method is less than 0, the value should be set to 0 . If the value sent to the method is greater than 0, we can assign this value to the instance variable. The first subclass to create is DVD. This class contains only an "own" instance variable, ageLimit, which is used to specify the age limit of a video. There must also be set and get methods for this instance variable. all properties are inherited from the Media class. The length of a DVD is stored in the class as the total number of seconds. In the setAgeLimit method, you must make a check so that the submitted value is not less than 0, the same check performed for the setLength method in the Media class. Some methods you need to overshadow in DVD to get the behavior we want. To begin with, the toString method should return a string representation of the current DVD object. print method should print all the values of the object and call the print method in the superclass to print its values. You must type a getLengthAsString () method. The method should return the length of the DVD as a string according to the format: HHh MMm SSs. string of hours (HH), minutes (MM) and seconds (SS) must always contain at least 2 digits. in cases where the hours, minutes or seconds are less than 10, a zero shall be set in front. Ex of strings that can be returned 00h 54m 09s (When length = 3249) 01h 09m 45s (When length = 4185) 100h 00m 00s (When length = 360000) There should be at least 2 different ways to create DVD objects. A constructor who takes 4 arguments and one who takes no arguments at all. Then it's up to you if you want more ways to create objects. It should not be possible to create a DVD whose length or age limit has a negative value (in which case change the value to 0). The 2th subclass you should create is Book which contains an instance variable, author. this class will inherit its properties from Media, but there will be set- and get- methods for author. The toString and print methods should be overshadowed and getLengthAsString should return the length of the book as a string according to the format: xxx page (s) if the number of pages is only 1, the page is printed according to the number of pages. If the number of pages is more than 1, pages are printed afterwards. Ex of strings that can be returned: 0 pages (length = 0) 1 page (length = 1) 321 pages (length = 321) Constructors for creating objects of Book should be present where one has 4 parameters and the other has no parameters at all. If you want more ways to create books, you are free to add designers for it.The final subclass is a CD that you do not need to make any major changes to. Thanks to the Media superclass, you can remove certain instance variables and methods from your original CD class. Some methods you need to overshadow so they fit into the inheritance structure. as for DVD and Book, the print and toString methods. Implementation of getLengthAsString should return the length of the CD as a string according to the format: HH: MM: SS. The string of hours (HH), minutes (MM) and seconds (SS) must always contain at least 2 digits. In cases where the hours, minutes or seconds are less than 10, a zero shall be set in front. Ex of strings that can be returned: 00:54:09 (When length = 3249) 01:09:45 (When length = 4185) 100: 00: 00(When length = 360000) there should be at least 2 different ways to create objects from CD. A constructor that has 4 parameters and one that has none at all. it's up to you if you want more ways to create objects. To check that everything works as intended, write a testclass that creates at least 2 objects each of Book, DVD and CD (at least 6 objects). Call it MediaTest. it should appropriately test all the methods available in the classes you have written. No requirement to use input to give the objects values.
create classes to manage CD and publisher and expand the classes so that you can handle book and DVD. This should be done with the help of inheritance. A general superclass, Media, will be created to be able to reuse common data and functionality between the CD, Book and DVD classes. These 3 classes must thus inherit their properties from the Media class. The Media class is a superclass for different type of media. you will collect instance variables and methods that are common to all type of media. All instance variables in the class are declared as protected, which mean that classes that inherit from Media have a direct access to these variables.The class only needs 2 constructors. One takes 3 argument that set values for all instance variables in the class, and the other takes no arguments but sets certain default values of the instance variables. If you think that more designers are necessary, you can add. In the setLength method, perform a check so that the value submitted is not less than zero. It should also not be possible to create a Media object whose length is less than 0. There is no point in setting the length of a media to -1. If the value sent to the method is less than 0, the value should be set to 0 . If the value sent to the method is greater than 0, we can assign this value to the instance variable. The first subclass to create is DVD. This class contains only an "own" instance variable, ageLimit, which is used to specify the age limit of a video. There must also be set and get methods for this instance variable. all properties are inherited from the Media class. The length of a DVD is stored in the class as the total number of seconds. In the setAgeLimit method, you must make a check so that the submitted value is not less than 0, the same check performed for the setLength method in the Media class. Some methods you need to overshadow in DVD to get the behavior we want. To begin with, the toString method should return a string representation of the current DVD object. print method should print all the values of the object and call the print method in the superclass to print its values. You must type a getLengthAsString () method. The method should return the length of the DVD as a string according to the format: HHh MMm SSs. string of hours (HH), minutes (MM) and seconds (SS) must always contain at least 2 digits. in cases where the hours, minutes or seconds are less than 10, a zero shall be set in front. Ex of strings that can be returned
00h 54m 09s (When length = 3249)
01h 09m 45s (When length = 4185)
100h 00m 00s (When length = 360000)
There should be at least 2 different ways to create DVD objects. A constructor who takes 4 arguments and one who takes no arguments at all. Then it's up to you if you want more ways to create objects. It should not be possible to create a DVD whose length or age limit has a negative value (in which case change the value to 0). The 2th subclass you should create is Book which contains an instance variable, author. this class will inherit its properties from Media, but there will be set- and get- methods for author. The toString and print methods should be overshadowed and getLengthAsString should return the length of the book as a string according to the format: xxx page (s)
if the number of pages is only 1, the page is printed according to the number of pages. If the number of pages is more than 1, pages are printed afterwards. Ex of strings that can be returned: 0 pages (length = 0)
1 page (length = 1)
321 pages (length = 321)
Constructors for creating objects of Book should be present where one has 4 parameters and the other has no parameters at all. If you want more ways to create books, you are free to add designers for it.The final subclass is a CD that you do not need to make any major changes to. Thanks to the Media superclass, you can remove certain instance variables and methods from your original CD class. Some methods you need to overshadow so they fit into the inheritance structure. as for DVD and Book, the print and toString methods. Implementation of getLengthAsString should return the length of the CD as a string according to the format: HH: MM: SS. The string of hours (HH), minutes (MM) and seconds (SS) must always contain at least 2 digits. In cases where the hours, minutes or seconds are less than 10, a zero shall be set in front. Ex of strings that can be returned:
00:54:09 (When length = 3249)
01:09:45 (When length = 4185)
100: 00: 00(When length = 360000)
there should be at least 2 different ways to create objects from CD. A constructor that has 4 parameters and one that has none at all. it's up to you if you want more ways to create objects.
To check that everything works as intended, write a testclass that creates at least 2 objects each of Book, DVD and CD (at least 6 objects). Call it MediaTest. it should appropriately test all the methods available in the classes you have written. No requirement to use input to give the objects values.
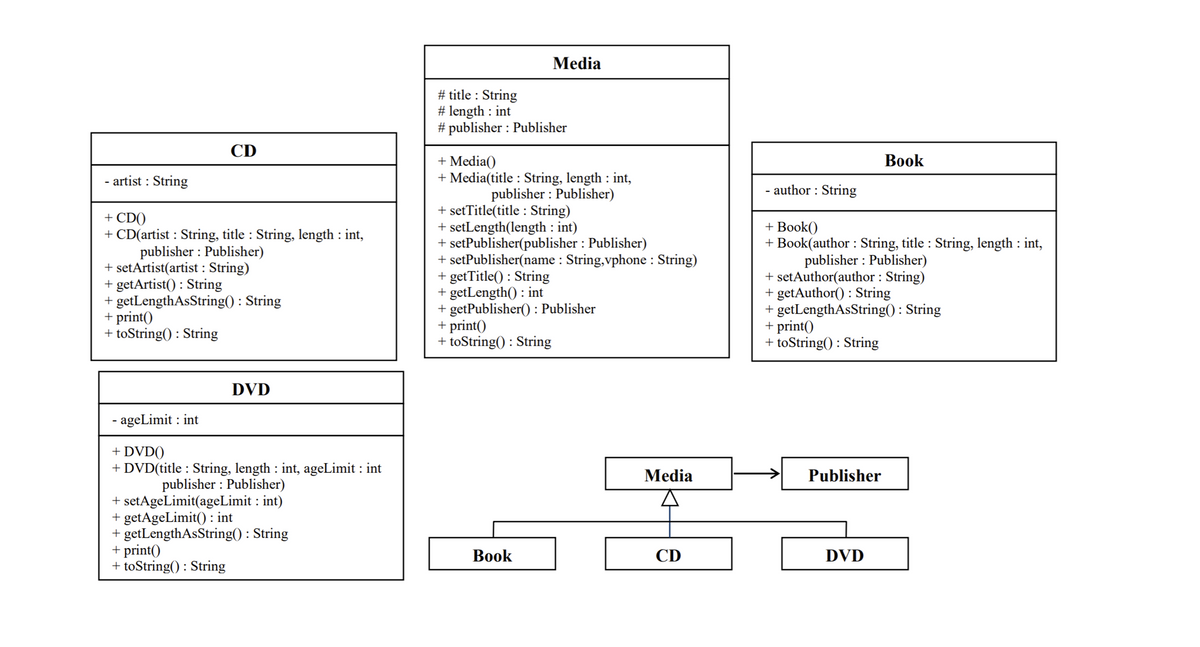
![Publisher
CD
PublisherTest1
- title : String
- artist : String
- length : int
- publisher : Publisher
CDTest
- name : String
- phone : String
- nrOfPublishers : int
use
use
1..1
3..*
+ main(args : String[ ])
1.1
+ main(args : String[ ])
3.*
+ CD()
+ CD(title : String, artist : String, lenth : int,
publisher : Publisher)
+ setLength(length : int)
+ setTitle(title : String)
+ setArtist(artist : String)
+ setPublisher(publisher : Publisher)
setPublisher(name : String, phone : String)
+ getLength() : int
getTitle() : String
getArtist() : String
+ getPublisher() : Publisher
+ print()
+ toString() : String
+ Publisher()
+ Publisher(name : String, phone : String)
+ setName(name : String)
+ setPhone(phone : String)
+ getName() : String
+ getPhone() : String
+ print()
1.*
PublisherTest2
Publisher
Has
use
1.1
0..*
1..1
+ main(args : String[ ])
+ toString() : String
+ getnrOfPublishers() : int
Requirement
In order for the assignment to be considered approved, the above-mentioned details and this are required:
• protected should be used for instance variables in the Media class
• No public instance variables may otherwise be in any class, only methods may be public.
• Classes and methods do not need to be commented on with documentation comments if you do not want to.
• In the subclass print method, the length of the current media should be printed with the string that the getlengthAsString method returns. For CDs and DVDS, printing should be done in
two digits for hours, minutes and seconds (examples 00, 02, 10, 23 etc).
• In the subclass print method, a call to the superclass print must be made.
• In the subclass constructor, a suitable constructor in the superclass must be called.
• All screen prints should be well-structured and easy to understand.
• For the methods şetlength, and setAgelimit () it should not be possible to set a negative number.
Tip
• Use modulus (%) together with division to divide the length (number of seconds) into hours, minutes and seconds.
• Or try what the following statements do:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe8260224-635c-426e-a960-28f2cf28de1f%2F06134765-b08d-4fd4-aea4-de8157bad724%2Fphu688_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

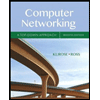
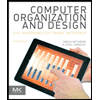
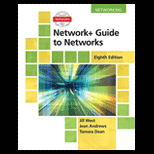
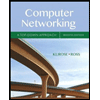
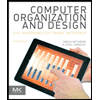
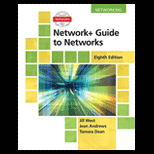
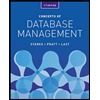
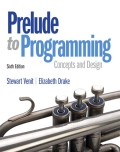
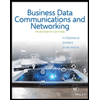