Design and implement a data class. The class will store data that has been read as user input from the keyboard (see Getting Input below), and provide necessary operations. As the data stored relates to monetary change, the class should be named Change. The class requires at least 2 instance variables for the name of a person and the coin change amount to be given to that person. You may also wish to use 4 instance variables to represent amounts for each of the 4 coin denominations (see Client Class below). There should be no need for more than these instance variables. However, if you wish to use more instance variables, you must provide legitimate justification for their usage in the internal and external documentation. Your class will need to have at least a default constructor, and a constructor with two parameters: one parameter being a name and the other a coin amount. Your class should also provide appropriate get and set methods for client usage. Other methods may be provided as needed. However, make sure they are necessary for good class design; In particular, your class should NOT include Input and Output methods. The only way to get data out of a data class object to the client program is to use an appropriate get method. The data class methods must not write data out. Data should be entered into a data class object via a constructor or an appropriate set method. When designing your Change class, use an UML class diagram to help understand what the class design needs. Getting Input: Input for the client program will come from keyboard (entered by the user). The input should consist of: the name of a person, and a coin value (as an integer). The program should validate the input coin value to ensure that it is in the range 5 to 95, and is evenly divisible by 5. Names are one-word strings. You should ask the user to enter the required information using a loop with a question after each loop iteration to check if the user wants to end the input of data. It is recommended for the user to input at least 12 such data – this can be conveyed to the user using a message before entering the loop. NOTE: provide a method in the client class that hardcodes data into at least 12 Change objects and stores these objects into the array provided by your program. In this case, the user would not need to manually key in the data to test the program when testing the program. Provide a call to this method (commented out) in the main function. Client Class: The client program should read the input data from the user (or the method with hardcoded inputs) and use the Change class to store the data entered. This data should be stored in a Change class object. You will need a data structure to store the Change class objects according to the number of persons entered - utilize an array of Change objects. It should be noted that it is possible to have the same name entered numerous times, but the coin values for such repetitions could be different. When the name is the same, it would mean the same individual, and your program should add up the coin amounts to obtain a total amount for that individual; this should be performed before computing the change to be given. Note that in this scenario, the total amount for an individual may end up being over 100, 200, 300, or more cents. Make sure you have hardcoded test cases of the above. Processing would involve determining repeated names and accumulating the total for those repeated names. You must ensure that there are no objects with repeated names in the array. Then methods would need to be called to calculate the required output corresponding to the coin amounts stored in the array of objects. Output change values must consist of the following denominations: 50, 20, 10 and 5 cents. Once the data input has been completed, your program should then display a menu screen as illustrated below. The program will continue to show the menu and execute the menu options until "Exit" is selected by entering the value 5 at the menu prompt. 1. Enter a name and display change to be given for each denomination 2. Find the name with the smallest amount and display change to be given for each denomination 3. Find the name with the largest amount and display change to be given for each denomination 4. Calculate and display the total number of coins for each denomination, and the sum of these totals 5. Exit The client program requires a structure chart, a high-level algorithm and low-level algorithm (i.e. suitable de-compositions of each step in the high-level algorithm).
Design and implement a data class. The class will store data that has been read as
user input from the keyboard (see Getting Input below), and provide necessary operations. As the data stored relates to monetary change, the class should be named Change. The class requires at least 2 instance variables for the name of a person and the coin change amount to be given to that person. You may also wish to use 4 instance variables to represent amounts for each of the 4 coin denominations (see Client Class below). There should be no need for more than these instance variables. However, if you wish to use more instance variables, you must provide legitimate justification for their usage in the internal and external documentation. Your class will need to have at least a default constructor, and a constructor with two parameters: one parameter being a name and the other a coin amount. Your class should also provide appropriate
get and set methods for client usage. Other methods may be provided as needed. However, make sure they are necessary for good class design; In particular, your class should NOT include Input and Output methods. The only way to get data out of a data class object to the client program is to use an appropriate get method. The data class methods must not write data out. Data should be entered into a data class object via a constructor or an appropriate set method. When designing your Change class, use an UML class diagram to help understand what the class design needs.
Getting Input:
Input for the client program will come from keyboard (entered by the user). The input should consist of: the name of a person, and a coin value (as an integer). The program should validate the input coin value to ensure that it is in the range 5 to 95, and is evenly divisible by 5. Names are one-word strings. You should ask the user to enter the required information using a loop with a question after each loop iteration to check if the user wants to end the input of data. It is recommended for the user to input at least 12 such data – this can be conveyed to the user using a message before entering the loop.
NOTE: provide a method in the client class that hardcodes data into at least 12 Change objects and stores these objects into the array provided by your program. In this case, the user would not need to manually key in the data to test the program when testing the program. Provide a call to this method (commented out) in the main function.
Client Class:
The client program should read the input data from the user (or the method with hardcoded inputs) and use the Change class to store the data entered. This data should be stored in a Change class object. You will need a data structure to store the Change class objects according to the number of persons entered - utilize an array of Change objects.
It should be noted that it is possible to have the same name entered numerous times, but the coin values for such repetitions could be different. When the name is the same, it would mean the same individual, and your program should add up the coin amounts to obtain a total amount for that individual; this should be performed before computing the change to be given. Note that in this scenario, the total amount for an individual may end up being over 100, 200, 300, or more cents. Make sure you have hardcoded test cases of the above.
Processing would involve determining repeated names and accumulating the total for those repeated names. You must ensure that there are no objects with repeated names in the array. Then methods would need to be called to calculate the required output corresponding to the coin amounts stored in the array of objects. Output change values must consist of the following denominations: 50, 20, 10 and 5 cents. Once the data input has been completed, your program should then display a menu screen as
illustrated below. The program will continue to show the menu and execute the menu options until "Exit" is selected by entering the value 5 at the menu prompt.
1. Enter a name and display change to be given for each denomination
2. Find the name with the smallest amount and display change to be
given for each denomination
3. Find the name with the largest amount and display change to be
given for each denomination
4. Calculate and display the total number of coins for each
denomination, and the sum of these totals
5. Exit
The client program requires a structure chart, a high-level

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

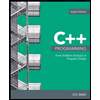
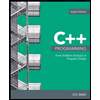