Deeper Class Design - the Square Class In this section, your job will be to write a new class that will adhere to the methods and data outlined in the “members” section below (said another way, your classes will implement a specific set of functions (called an interface) that we’ll describe contractually, ahead of time). These classes will all be shapes, with actions (methods) that are appropriate to shape objects, such as “getArea()”, or “draw()”. First we build a Square class that contains the following data and methods listed below, and then later, we will copy it to quickly make a Circle class. Note: when considering each data item below, think about what type of data would best represent the concept we’re trying to model in our software. For example, all shapes will have a coordinate pair indicating their location in a Cartesian coordinate system. This x and y pair may be modeled as ints or floats, and you might choose between the two depending on what the client application will do (i.e., how it would use these shapes and at what precision).
Deeper Class Design - the Square Class
In this section, your job will be to write a new class that will adhere to the methods and data outlined in the “members” section below (said another way, your classes will implement a specific set of functions (called an interface) that we’ll describe contractually, ahead of time). These classes will all be shapes, with actions (methods) that are appropriate to shape objects, such as “getArea()”, or “draw()”. First we build a Square class that contains the following data and methods listed below, and then later, we will copy it to quickly make a Circle class.
Note: when considering each data item below, think about what type of data would best represent the concept we’re trying to model in our software. For example, all shapes will have a coordinate pair indicating their location in a Cartesian coordinate system. This x and y pair may be modeled as ints or floats, and you might choose between the two depending on what the client application will do (i.e., how it would use these shapes and at what precision).
Data Members (the state of our object; these are private class-level variables):
- Define an x position variable.
- What’s a good primitive type for this?
- Define y position variable.
- Make this private and the same type used for x.
- Define a variable to represent the sideLength amount.
- Use a double for this.
- Add a String to represent this shape for console output (for now, just “[ ]” ).
- A Color (optional) (use java.awt.Color)
Public methods (the object’s actions; often called its interface):
- A Square constructor that takes no arguments
- public Square() {
- A Square constructor that takes an initial x,y pair
- public Square(int nx, ...) {
- Note that we’re overloading this constructor.
- public Square(int nx, ...) {
- A Square constructor that takes an x,y pair and a starting side length.
- Another overloaded constructor.
- Build a draw() method that outputs to the console the characters used to represent this shape (“[]”)
- public void draw() {
- Next, let’s build our accessor methods:
- int getX()
- This returns the value stored in x.
- int getY()
- Accessor method that returns the value of y.
- double getSideLength()
- Another accessor method for getting the value of the Square’s side length
- double getArea()
- returns the calculated area for this Square
- int getX()
- Now, our mutator methods:
- void setX(int nX) { //changes x to nX
- void setY(int nY) { //changes y to nY
- void setSideLength(double sl) { //change sidelength’s value to sl
- Build a reporting method called toString() that returns the characters associated with that shape.
- @Override
public String toString() { //sample : “[]”
- @Override
- Build an equals function to determine if two Squares are the same
- public boolean equals(Square that) {
- Returns true if the x,y pairs match & the sideLengths match.
- (hint: this.x == that.x && ...)
- public boolean equals(Square that) {

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

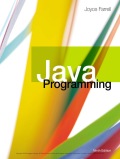
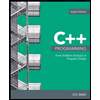
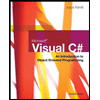
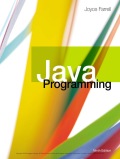
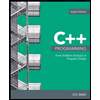
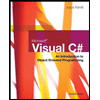