Create hash table and an appropriate hash function for use in storage and retrieval of character data. You will vary the size of the table and count the number of collisions that occurs with each table. Includes the following basic methods: init - mark all positions of the table as empty. hashMe - calculate the hash index based on properties of the data. getNextOpenPosition - in the event of a collision, search table for the next available piece of memory. showTable - prints out occupied positions in table. rehash - when collisions become frequent, allocate a new table with a larger size and then rehash the data from the current table into the new table using a hash function that is modified to take the new table's size into account. The Hashing function is: hashKey = function of data characteristics a) Create a hash table. Use your table to “sort” a list of names. A list of test names are below: joe bob harry mary brian tom jerry bullwinkle pam ellis dale bill barrack george gertrude zack zeus apollo gemini greg larry meriam webster thomas stewart dianna theresa billyjoe carl karl charles karla donna tena kerry howard johnson ulyssess paul peter issaac marvin dudz chuck ellie anny judy matt ross dan robert kim eric junkun ghassan cris raymond avery roy halley mitzee ziggy rocky twirly max huey dewy hongkongfooey clarence lala sammy fred francis b) Your hash function can be one that you develop or you can use the simple 3 letter function outlined below: Let the letters of the alphabet be resolved to the numbers 0 through 25. This can be accomplished by subtracting the letter 'a' from each letter. Equation 1 shows this relationship. Equation 1: letterVal = letter - 'a' The idea of equation 1 can be incorporated into a function of the following form: int getLetVal(char ch) Then a hash value can be generated using an equation similar to equation 2 below: Equation 2: nameHash = getLetVal(name[0])*262 + getLetVal(name[1])*26 + getLetVal(name[0]); c) You will run your program with three different sizes of hash tables: i) 200 ii) 400 iii) 700
Create hash table and an appropriate hash function for use in storage and retrieval of character data. You will vary the size of the table and count the number of collisions that occurs with each table.
Includes the following basic methods:
init - mark all positions of the table as empty.
hashMe - calculate the hash index based on properties of the data.
getNextOpenPosition - in the event of a collision, search table for the next available piece of memory.
showTable - prints out occupied positions in table.
rehash - when collisions become frequent, allocate a new table with a larger size and then rehash the data from the current table into the new table using a hash function that is modified to take the new table's size into account.
The Hashing function is: hashKey = function of data characteristics
a) Create a hash table. Use your table to “sort” a list of names. A list of test names are below:
joe bob harry mary brian tom jerry bullwinkle pam ellis dale bill barrack george gertrude zack zeus apollo gemini greg larry meriam webster thomas stewart dianna theresa billyjoe carl karl charles karla donna tena kerry howard johnson ulyssess paul peter issaac marvin dudz chuck ellie anny judy matt ross dan robert kim eric junkun ghassan cris raymond avery roy halley mitzee ziggy rocky twirly max huey dewy hongkongfooey clarence lala sammy fred francis
b) Your hash function can be one that you develop or you can use the simple 3 letter function outlined below:
Let the letters of the alphabet be resolved to the numbers 0 through 25. This can be accomplished by subtracting the letter 'a' from each letter. Equation 1 shows this relationship.
Equation 1:
letterVal = letter - 'a'
The idea of equation 1 can be incorporated into a function of the following form: int getLetVal(char ch)
Then a hash value can be generated using an equation similar to equation 2 below:
Equation 2:
nameHash = getLetVal(name[0])*262 + getLetVal(name[1])*26 + getLetVal(name[0]);
c) You will run your program with three different sizes of hash tables:
i) 200
ii) 400
iii) 700
d) You will have to adjust your hashing function based on the size of the hash table, meaning you will need to divide the nameHash value by a denominator that maps the names into the table. For example, if using the hash table with size 200, a very rough estimate of the denominator is 100. Why? Because the max value of the hash function using a name that starts with "zzz", like "zzztop" (a very sleepy version of the band from Texas, "ZZTop"), is around 17, 500. That will not fit in a hash table of size 200, but if you divide it by 100, it maps "zzztop" to hash index 175.
e) You will need to have a collision management scheme.
i) Note, record the number of collisions that occur for each different size. If it is done correctly, each time the table gets bigger, the number of collisions should decrease.
f) Print out the contents of the table for each different size of hash table. Look closely at the printout. Which size of table did the best job of arranging the names in alphabetical ordering in the memory.
g) Below is some code to help in program development. Please use this!!!
import java.util.Scanner; import java.io.*;
import java.lang.Math;
public class hashMe { String fname; String hashTable[]; int tabLen; int numSmashes; public hashMe(int hashTableLen) { int j;
/* Initialize variables. */ tabLen = hashTableLen; numSmashes = 0;
/* Initialize hash table. */ hashTable = new String[tabLen];
for(j=0;j
}
getFileName(); readFileContents(); showHashTable();
}
public void readFileContents() { boolean looping; DataInputStream in; String line; int j, len, hashIndex, hInc, spot;
/* Read input from file and process. */ try {
in = new DataInputStream(new FileInputStream(fname)); looping = true; while(looping) {
/* Get a line of input from the file. */ if (null == (line = in.readLine())) {
looping = false;
/* Close and free up system resource. */ in.close();
}
else {
hashIndex = hashFun(line); }
} /* End while. */ } /* End try. */
catch(IOException e) { System.out.println("Error " + e);
} /* End catch. */ }
void showHashTable() {
int j;
System.out.println("Number of Hash Clashes = ");
for(j=0;j
} }
public int hashFun(String name) {
int hashVal;
return hashVal; } public void getFileName() {
Scanner in = new Scanner(System.in);
System.out.println("Enter file name please."); fname = in.nextLine();
System.out.println("You entered "+fname);
}
public static void main(String[] args) {
System.out.println("Hello TV land!");
hashMe h = new hashMe(200);
System.out.println("Bye-bye!");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

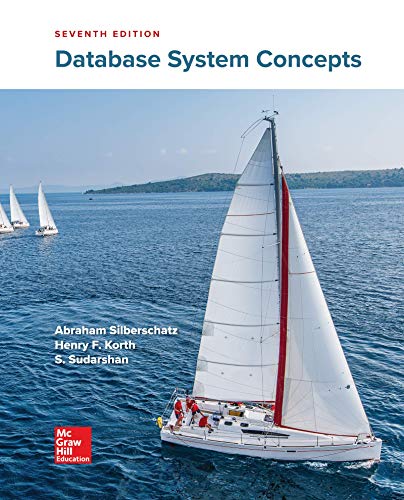
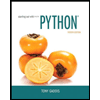
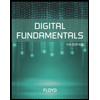
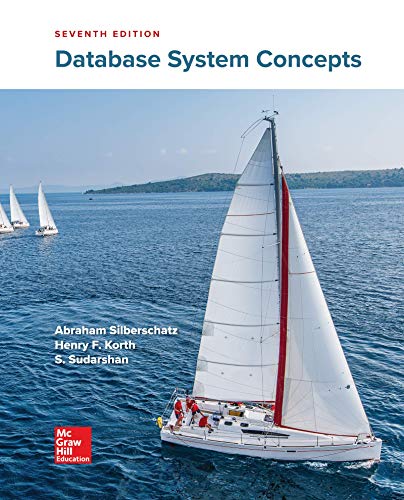
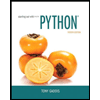
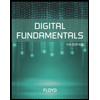
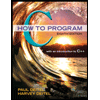
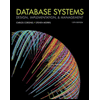
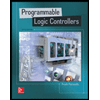