Create an object of MessageDigest class using the java.security.MessageDigest library. Initialize the object with your selection for an appropriate algorithm cipher. Use the digest() method of the class to generate a hash value of byte type from the unique data string (your first and last name). Convert the hash value to hex using the bytesToHex function. Create a RESTFul route using the @RequestMapping method to generate and return the required information, which includes the hash value, to the web browser. Here, is the code to edit package com.snhu.sslserver; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication public class ServerApplication { public static void main(String[] args) { SpringApplication.run(ServerApplication.class, args); } } @RestController class ServerController{ //FIXME: Add hash function to return the checksum value for the data string that should contain your name. @RequestMapping("/hash") public String myHash(){ String data = "Hello Mike Bux!"; return "data:"+data; } }
- Create an object of MessageDigest class using the java.security.MessageDigest library.
- Initialize the object with your selection for an appropriate
algorithm cipher. - Use the digest() method of the class to generate a hash value of byte type from the unique data string (your first and last name).
- Convert the hash value to hex using the bytesToHex function.
- Create a RESTFul route using the @RequestMapping method to generate and return the required information, which includes the hash value, to the web browser.
Here, is the code to edit
package com.snhu.sslserver;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class ServerApplication {
public static void main(String[] args) {
SpringApplication.run(ServerApplication.class, args);
}
}
@RestController
class ServerController{
//FIXME: Add hash function to return the checksum value for the data string that should contain your name.
@RequestMapping("/hash")
public String myHash(){
String data = "Hello Mike Bux!";
return "<p>data:"+data;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

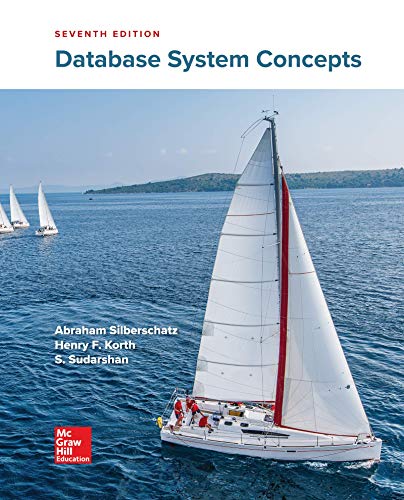
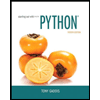
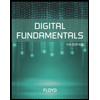
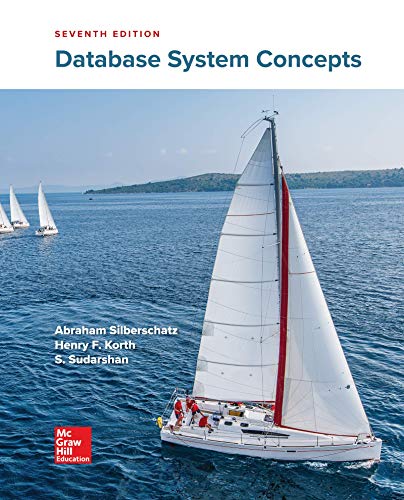
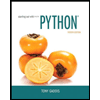
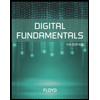
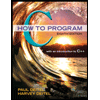
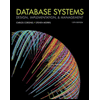
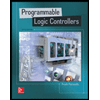