CS Java Program (NEED HELP WITH THE ACTUAL CODING PART): For this project, you will create an application for a zoo. You will need to create a class that implements the iAnimal interface (provided below), and use one other class for an additional animal that implements the iAnimal interface as well (provided below). Modify the animal classes that you will use for this project so they implement Java’s comparable or comparator interface. You will also need a Main Class that will provide a user interface for this application. This can be a console-based interface. Tasks can be performed in one or more methods as needed. The Main Class should have one or more methods that handle these tasks: Create a new instance (object) of each of the animal classes and pass the user input as arguments to the object's mutator methods to set the private instance data for the appropriate animal class. Create an ArrayList of type iAnimal and add the new instance created in task 1 to the ArrayList. Before adding the instance to the ArrayList you must: Call the compareTo method for the new animal's instance to compare that new instance to each of the instances already in the ArrayList (use a loop or recursion for this). If the ArrayList is empty, or if none of the instances are identical to the new instance, insert the new instance into the ArrayList. If the new instance is identical to any of the instances already in the ArrayList, display an error message and do not insert the new instance. When testing, add multiple instances of your animal's class and multiple instances of the class you borrowed from the discussion to the ArrayList as described above. Because you are working with more than one class, you can decide how to determine which class to use based on user input, but the goal is to create an instance of the class that matches the type of animal entered by the user. Use a loop to iterate through the ArrayList and display all of the data for each instance in the ArrayList using accessor or ToString methods. Classes for iAnimal Interface: public class Panda implements iAnimal { private String animalType = “Panda"; private int idTag; private int minTemperature = 30; private int maxTemperature = 80; public String getAnimalType() { return animalType; } public int getIdTag() { return idTag; } public void setIdTag(int anIdTag) { idTag = anIdTag; } public int getMinTemperature() { return minTemperature; } public int getMaxTemperature() { return maxTemperature; } } public class Tiger implements iAnimal { private String animalType = “Tiger"; private int anIdTag; private int minTemp = 44; private int maxTemp = 77; public String getAnimalType() { return "Hamster"; } public int getIdTag() { return anIdTag; } public void setIdTag(int anIdTag) { this.anIdTag = anIdTag; } public int getMinTemperature() { return minTemp; } public int getMaxTemperature() { return maxTemp; }
CS Java Program (NEED HELP WITH THE ACTUAL CODING PART):
For this project, you will create an application for a zoo.
You will need to create a class that implements the iAnimal interface (provided below), and use one other class for an additional animal that implements the iAnimal interface as well (provided below). Modify the animal classes that you will use for this project so they implement Java’s comparable or comparator interface.
You will also need a Main Class that will provide a user interface for this application. This can be a console-based interface. Tasks can be performed in one or more methods as needed.
The Main Class should have one or more methods that handle these tasks:
- Create a new instance (object) of each of the animal classes and pass the user input as arguments to the object's mutator methods to set the private instance data for the appropriate animal class.
- Create an ArrayList of type iAnimal and add the new instance created in task 1 to the ArrayList. Before adding the instance to the ArrayList you must:
- Call the compareTo method for the new animal's instance to compare that new instance to each of the instances already in the ArrayList (use a loop or recursion for this).
- If the ArrayList is empty, or if none of the instances are identical to the new instance, insert the new instance into the ArrayList.
- If the new instance is identical to any of the instances already in the ArrayList, display an error message and do not insert the new instance.
- When testing, add multiple instances of your animal's class and multiple instances of the class you borrowed from the discussion to the ArrayList as described above. Because you are working with more than one class, you can decide how to determine which class to use based on user input, but the goal is to create an instance of the class that matches the type of animal entered by the user.
- Use a loop to iterate through the ArrayList and display all of the data for each instance in the ArrayList using accessor or ToString methods.
Classes for iAnimal Interface:
public class Panda implements iAnimal {
private String animalType = “Panda";
private int idTag;
private int minTemperature = 30;
private int maxTemperature = 80;
public String getAnimalType() {
return animalType;
}
public int getIdTag() {
return idTag;
}
public void setIdTag(int anIdTag) {
idTag = anIdTag;
}
public int getMinTemperature() {
return minTemperature;
}
public int getMaxTemperature() {
return maxTemperature;
}
}
public class Tiger implements iAnimal {
private String animalType = “Tiger";
private int anIdTag;
private int minTemp = 44;
private int maxTemp = 77;
public String getAnimalType() {
return "Hamster";
}
public int getIdTag() {
return anIdTag;
}
public void setIdTag(int anIdTag) {
this.anIdTag = anIdTag;
}
public int getMinTemperature() {
return minTemp;
}
public int getMaxTemperature() {
return maxTemp;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

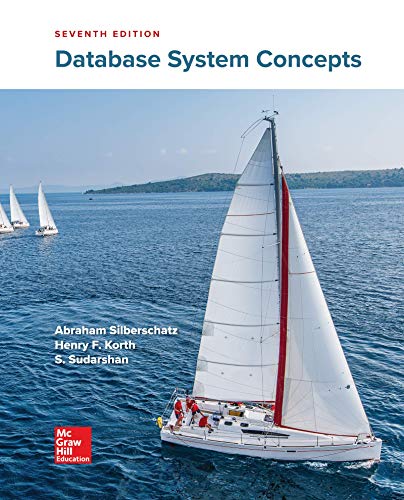
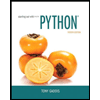
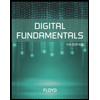
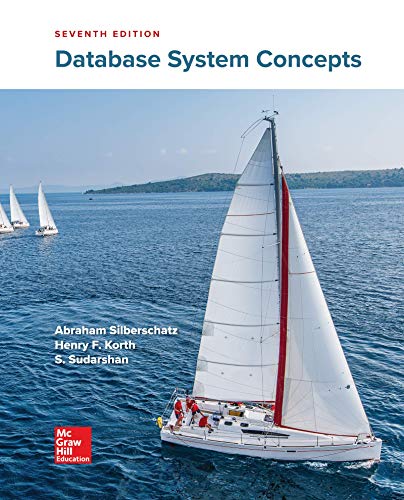
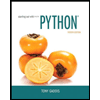
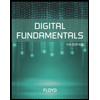
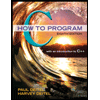
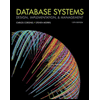
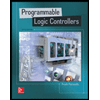