Design and implement a Java program/application that 1. Uses at least one of the following collections: a. Stack b. Queue c. List d. Tree 2. Uses at least one of the following searching methods: a. Linear search b. Binary search 3. Uses at least one of the following sorting methods: a. Bubble sort b. Insertion sort c. Selection sort d. Quick sort e. Merge sort I'm using an insertion sort for this project. I would like help implementing a list collection type and binary search method. //Project with Insertion sort import java.util.Random; public class main{ static void insertionSort(int arr[]){ int n = arr.length; for (int i = 1; i < n; ++i){ int key = arr[i]; int j = i - 1; while (j >= 0 && arr[j] > key){ arr[j + 1] = arr[j]; j = j - 1; } arr[j + 1] = key; } } public static void main(String[] args){ int max = 100; int min = 1; int arr[]=new int[10]; Random randomNum = new Random(); for(int i=0;i
Design and implement a Java program/application that
1. Uses at least one of the following collections:
a. Stack
b. Queue
c. List
d. Tree
2. Uses at least one of the following searching methods:
a. Linear search
b. Binary search
3. Uses at least one of the following sorting methods:
a. Bubble sort
b. Insertion sort
c. Selection sort
d. Quick sort
e. Merge sort
I'm using an insertion sort for this project. I would like help implementing a list collection type and binary search method.
//Project with Insertion sort
import java.util.Random;
public class main{
static void insertionSort(int arr[]){
int n = arr.length;
for (int i = 1; i < n; ++i){
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key){
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
}
}
public static void main(String[] args){
int max = 100;
int min = 1;
int arr[]=new int[10];
Random randomNum = new Random();
for(int i=0;i<arr.length;i++){
arr[i] = min + randomNum.nextInt(max);
}
System.out.println("Array of random number is: ");
for(int i=0;i<arr.length;i++){
System.out.print(arr[i]+ " ");
}
insertionSort(arr);
System.out.println("\nSorted array is: ");
for(int i=0;i<arr.length;i++){
System.out.print(arr[i]+ " ");
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

I'd like to know the
import java.util.Random;
import java.util.*;
public class main{
static void insertionSort(int arr[]){
int n = arr.length;
for (int i = 1; i < n; ++i){
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key){
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
}
}
public static void main(String[] args){
int max = 100;
int min = 1;
int arr[]=new int[10];
int n;
@SuppressWarnings("resource")
Scanner sc=new Scanner(System.in);
Random randomNum = new Random();
for(int i=0;i<arr.length;i++){
arr[i] = min + randomNum.nextInt(max);
}
System.out.println("Array of random number is: ");
for(int i=0;i<arr.length;i++){
System.out.print(arr[i]+ " ");
}
insertionSort(arr);
System.out.println("\nSorted array is: ");
for(int i=0;i<arr.length;i++){
System.out.print(arr[i]+ " ");
}
System.out.println();
System.out.print("\nEnter an element to search: ");
n=sc.nextInt();
List<Integer> list = new ArrayList<Integer>();
for (int i = 0; i < arr.length; i++){
list.add(arr[i]);
}
System.out.println("The element is at position: "+(Collections.binarySearch(list, n)+1));
}
}
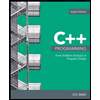
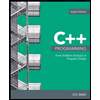