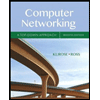
Concept explainers
Write a java class named First_Last_Recursive_Merge_Sort that
implements the recursive
You can use the structure below for your implementation.
public class First_Last_Recursive_Merge_Sort {
//This can be used to test your implementation.
public static void main(String[] args) {
final String[] items = {"Zeke", "Bob", "Ali", "John",
"Jody", "Jamie", "Bill", "Rob", "Zeke", "Clayton"};
display(items, items.length - 1);
mergeSort(items, 0, items.length - 1);
display(items, items.length - 1);
}
private static <T extends Comparable<? super T>>
void mergeSort(T[] a, int first, int last)
{
//<Your implementation of the recursive algorithm for Merge Sort
should go here>
} // end mergeSort
private static <T extends Comparable<? super T>>
void merge(T[] a, T[] tempArray, int first,
int mid, int last)
{
//<Your implementation of the merge algorithm should go here>
} // end merge
//Just a quick method to display the whole array.
public static void display(Object[] array, int n)
{
for (int index = 0; index < n; index++)
System.out.println(array[index]);
System.out.println();
} // end display
}// end First_Last_Recursive_Merge_Sort

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Analyze the following code: import java.util.*; public class Test { public static void main(String[] args) { PriorityQueue queue = } } new PriorityQueue( Arrays.asList(60, 10, 50, 30, 40, 20)); for (int i: queue) System.out.print(i + "); The program displays 60 10 50 30 40 20 The program displays 10 20 30 40 50 60 The program displays 60 50 40 30 20 10 There is no guarantee that the program displays 10 20 30 40 50 60arrow_forwardThe language used here is in Java. Please answer the question in the screenshot. Please use the code below as a base. import java.util.*; class SortingPack { // just in case you need tis method for testing public static void main(String[] args) { // something } // implementation of insertion sort // parameters: int array unsortedArr // return: sorted int array public static int[] insertionSort(int[] unsortedArr) { // to be removed return unsortedArr; } // implementation of quick sort // parameters: int array unsortedArr // return: sorted int array public static int[] quickSort(int[] unsortedArr) { // to be removed return unsortedArr; } // implementation of merge sort // parameters: int array unsortedArr // return: sorted int array public static int[] mergeSort(int[] unsortedArr) { // to be removed return unsortedArr; } // you are welcome to add any supporting methods }arrow_forwardvoid getInput(){for(int i =0; i < studentName.length; i++){System.out.print("Student name: ");studentName[i] = keyboard.nextLine();System.out.print("Studnet ID: ");midTerm1[i] = keyboard.nextInt();}keyboard.close();} Can't put full student name becuase nextLine();arrow_forward
- Please help me fix the errors in the java program belowarrow_forwardPlease do not change any of the method signatures in either class. Implement the methods described below. RadixSort.java RadixSort.java contains two different RadixSort implementations each using a different version of Counting Sort that you will implement. As a reminder the pseudocode for CountingSort discussed in class is as follows. countingSort(arr, n, k) 1. let B[1 : n] and C[0 : k] be new arrays 2. for i = 0 to k 3. C[i] = 0 4. for j = 1 to n 5 . C[arr[j]] = C [arr[j]] + 1 6. for i = 1 to k 7. C[i] = C[i] + C[i – 1] 8. for j = n downto 1 9. B[C[arr[j]]] = arr[j] 10 C[arr[j]] = C[arr[j]] – 1 11. return B private static void countingSort2(int[] arr, int k) Now you implement almost the same method, but instead of iterating from the end of arr, you will instead iterate from the beginning. So line 8 becomes for j=1 to n. You should think about why this alternative does not impact the correctness of CountingSort. You don’t need to write this down, just think about it.…arrow_forwardWite in java. Dont plagirize. Add comments. Keep code neat. Need this by today.arrow_forward
- using java code Problem: Suppose we want to write a program for the class BinaryTree that counts the number of times an object occurs in the tree. We need to use a method with the following header, public int count (O anObject) 1. Name your class objectCounter 2. Define main method, test the program 3. Write a method using one of the iterators of the binary tree. 4. Write another method using a private recursive method of the same name NB: Can you add main method to test the code please for 2. Define main method, test the programarrow_forward44 // create a loop that reads in the next int from the scanner to the intList 45 46 47 // call the ReverseArray function 48 49 50 System.out.print("Your output is: "); 51 // print the output from ReverseArray 52 ww 53 54 55 }arrow_forwardpackage edu.umsl.iterator;import java.util.ArrayList;import java.util.Arrays;import java.util.Collection;import java.util.Iterator;public class Main {public static void main(String[] args) {String[] cities = {"New York", "Atlanta", "Dallas", "Madison"};Collection<String> stringCollection = new ArrayList<>(Arrays.asList(cities));Iterator<String> iterator = stringCollection.iterator();while (iterator.hasNext()) {System.out.println(/* Fill in here */);}}} Rewrite the while loop to print out the collection using an iterator. Group of answer choices iterator.toString() iterator.getClass(java.lang.String) iterator.remove() iterator.next()arrow_forward
- import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardUSING THE FOLLOWING METHOD SWAP CODE: import java.util.*; class ListIndexOutOfBoundsException extends IndexOutOfBoundsException { public ListIndexOutOfBoundsException(String s) { super(s); } } class ListUtil { public static int maxValue(List<Integer> aList) { if(aList == null || aList.size() == 0) { throw new IllegalArgumentException("List cannot be null or empty"); } int max = aList.get(0); for(int i = 1; i < aList.size(); i++) { if(aList.get(i) > max) { max = aList.get(i); } } return max; } public static void swap(List<Integer> aList, int i, int j) throws ListIndexOutOfBoundsException { if(aList == null) { throw new IllegalArgumentException("List cannot be null"); } if(i < 0 || i >= aList.size() || j < 0 || j >= aList.size()) { throw new ListIndexOutOfBoundsException("Index out…arrow_forwardJAVAarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
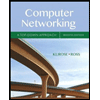
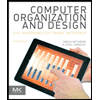
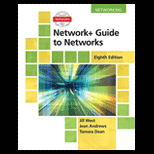
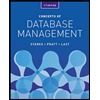
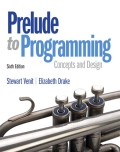
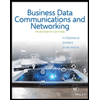