Java --- Please help me understand further the code below. Thank you!| --- Circle.java public class Circle implements Shape { final float PI = 3.14f; int radius; public Circle(int radius) { this.radius = radius; } @Override public double getPerimeter() { return(2 * PI * radius); } @Override public double getArea() { return(PI * radius*radius); } @Override public void getDetails() { System.out.println("Type: Circle"); System.out.println("Radius: " + radius); System.out.printf("Perimeter: %.1f\n", getPerimeter()); System.out.printf("Area: %.1f\n", getArea()); } } Rectangle.java public class Rectangle implements Shape { int length, width; public Rectangle(int a, int b) { this.length = b; this.width = a; } @Override public double getPerimeter() { return(2 * (length + width)); } @Override public double getArea() { return(length * width); } @Override public void getDetails() { System.out.println("Type: Rectangle"); System.out.println("Lengh: "+ length); System.out.println("Width: "+ width); System.out.printf("Perimeter: %.1f\n", getPerimeter()); System.out.printf("Area: %.1f\n", getArea()); } } Square.java public class Square implements Shape { int side; public Square(int a) { this.side = a; } @Override public double getPerimeter() { return(4 * side); } @Override public double getArea() { return(side * side); } @Override public void getDetails() { System.out.println("Type: Square"); System.out.println("Side: " + side); System.out.printf("Perimeter: %.1f\n", getPerimeter()); System.out.printf("Area: %.1f\n", getArea()); } } Triangle.java public class Triangle implements Shape { int sideA, sideB, sideC; public Triangle(int a, int b, int c) { this.sideA = a; this.sideB = b; this.sideC = c; } @Override public double getPerimeter() { return(sideA + sideB + sideC); } @Override public double getArea() { float s = (sideA+sideB+sideC)/2; return(Math.sqrt(s * (s - sideA) * (s - sideB) * (s - sideC))); } @Override public void getDetails() { System.out.println("Type: Triangle"); System.out.printf("Sides: %d, %d, %d \n", sideA, sideB, sideC); System.out.printf("Perimeter: %.1f\n", getPerimeter()); System.out.printf("Area: %.1f\n", getArea()); } } Shape.java public interface Shape { double getPerimeter(); double getArea(); void getDetails(); }
Java
---
Please help me understand further the code below. Thank you!|
---
Circle.java
public class Circle implements Shape
{
final float PI = 3.14f;
int radius;
public Circle(int radius)
{
this.radius = radius;
}
@Override
public double getPerimeter()
{
return(2 * PI * radius);
}
@Override
public double getArea()
{
return(PI * radius*radius);
}
@Override
public void getDetails()
{
System.out.println("Type: Circle");
System.out.println("Radius: " + radius);
System.out.printf("Perimeter: %.1f\n", getPerimeter());
System.out.printf("Area: %.1f\n", getArea());
}
}
Rectangle.java
public class Rectangle implements Shape
{
int length, width;
public Rectangle(int a, int b)
{
this.length = b;
this.width = a;
}
@Override
public double getPerimeter()
{
return(2 * (length + width));
}
@Override
public double getArea()
{
return(length * width);
}
@Override
public void getDetails()
{
System.out.println("Type: Rectangle");
System.out.println("Lengh: "+ length);
System.out.println("Width: "+ width);
System.out.printf("Perimeter: %.1f\n", getPerimeter());
System.out.printf("Area: %.1f\n", getArea());
}
}
Square.java
public class Square implements Shape
{
int side;
public Square(int a)
{
this.side = a;
}
@Override
public double getPerimeter()
{
return(4 * side);
}
@Override
public double getArea()
{
return(side * side);
}
@Override
public void getDetails()
{
System.out.println("Type: Square");
System.out.println("Side: " + side);
System.out.printf("Perimeter: %.1f\n", getPerimeter());
System.out.printf("Area: %.1f\n", getArea());
}
}
Triangle.java
public class Triangle implements Shape
{
int sideA, sideB, sideC;
public Triangle(int a, int b, int c)
{
this.sideA = a;
this.sideB = b;
this.sideC = c;
}
@Override
public double getPerimeter()
{
return(sideA + sideB + sideC); }
@Override
public double getArea()
{
float s = (sideA+sideB+sideC)/2;
return(Math.sqrt(s * (s - sideA) * (s - sideB) * (s - sideC)));
}
@Override
public void getDetails()
{
System.out.println("Type: Triangle");
System.out.printf("Sides: %d, %d, %d \n", sideA, sideB, sideC);
System.out.printf("Perimeter: %.1f\n", getPerimeter());
System.out.printf("Area: %.1f\n", getArea());
}
}
Shape.java
public interface Shape
{
double getPerimeter();
double getArea();
void getDetails();
}
![D TestShape.java x
1 public class TestShape
{
public static void main(String[] args)
{
2
30
4
5
int a, b, c;
7
if (args.length < 1 || args.length > 3)
System.out.printin("Invalid input");
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
else
{
switch(args.length)
{
case 1:
{
a = Integer.parseInt(args[0]);
Circle circle = new Circle(a);
circle.getDetails();
break;
}
case 2:
{
a = Integer.parseInt(args[0]);
b = Integer.parseInt(args[1]);
// OF BOTH ARGS ARE EQUAL THEN SQUARE
if (a == b)
{
Square square = new Square(a);
square.getDetails();
}
else
{
Rectangle rect = new Rectangle(a, b);
rect.getDetails();
}
break;
}
// IF ARGS LENGTH IS THREE THEN TRIANGLE
case 3:
{
a = Integer.parseInt(args[0]);
b = Integer.parseInt (args[1]);
c = Integer.parseInt (args[2]);
Triangle t = new Triangle(a, b, c);
t.getDetails();
break;
}
}
}
}
55
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F200eab8b-1555-4a19-b68b-e447df014971%2F0c960038-7162-472f-9560-ffe27f15052e%2Fcdoa79_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 9 steps with 9 images

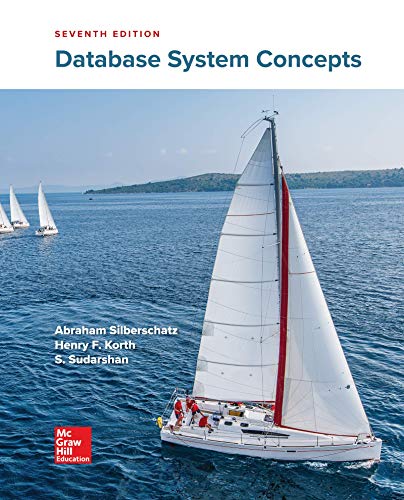
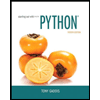
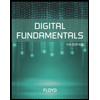
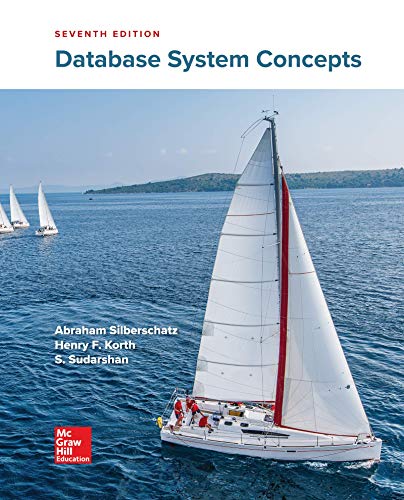
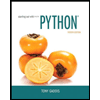
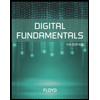
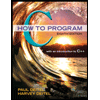
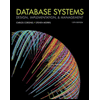
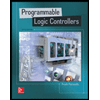