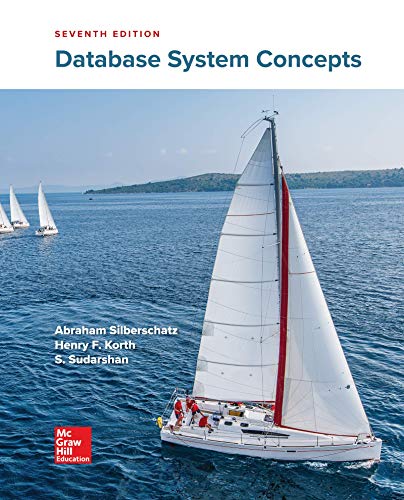
import java.awt.*;
public class TestRectangle {
public static void main(String[] args) {
Rectangle r1 = new Rectangle(5, 4, 10, 17);
Rectangle r2 = new Rectangle(10, 10, 20, 3);
Rectangle r3 = new Rectangle(0, 1, 12, 15);
Rectangle r4 = new Rectangle(10, 10, 20, 3);
System.out.println("r1 = " + r1);
System.out.println("r2 = " + r2);
System.out.println("r3 = " + r3);
System.out.println("r2 equals r1? " + r2.equals(r1));
System.out.println("r2 equals r2? " + r2.equals(r2));
System.out.println("r2 equals r3? " + r2.equals(r3));
System.out.println("r2 equals r4? " + r2.equals(r4));
System.out.println("r1 contains (6, 8)? = " + r1.contains(6, 8));
System.out.println("r2 contains (6, 8)? = " + r2.contains(6, 8));
System.out.println("r3 contains (6, 8)? = " + r3.contains(6, 8));
System.out.println("r2 contains (30, 13)? = " + r2.contains(30, 13));
r1.intersect(r3);
r2.intersect(r4);
System.out.println("r1 intersect r3 = " + r1);
System.out.println("r2 intersect r4 = " + r2);
r1.intersect(r2);
System.out.println("r1 intersect r2 = " + r1);
}
}
notes:please puts code in a order so when I put it in java it runs and gives output.
![2. Define a class named Rectangle. A Rectangle object stores an (x, y) coordinate of its top/left corner, a
width, and a height.
Each Rectangle object should have the following public methods:
Rectangle (x, y, width, height)
Constructs a new rectangle whose top-left corner is specified by the given coordinates and with the given
width and height.
getX()
Returns this rectangle's leftmost x-coordinate.
getY()
Returns this rectangle's top y-coordinate.
getWidth ()
Returns this rectangle's width.
getHeight ()
Returns this rectangle's height.
tostring ()
Returns a String representation of this rectangle, such as "Rectangle[x=1,y=2,width=3,height=4]".
contains (x, y)
Returns whether the given Point's coordinates lie inside the bounds of this rectangle.
intersect (rect)
Turns this rectangle into the intersection of itself and the given other rectangle rect;
that is, the largest rectangular region completely contained within both this rectangle
and the given other rectangle. (In the picture at right, the intersection of the two
rectagles is the shaded black region.) You may assume that the two rectangles do
overlap and therefore have a nonempty intersection.
equals (o)
Returns whether the given other object o is a rectangle with the same x/y coordinates, width, and height as
this rectangle. You may assume that o refers to a Rectangle object.
Test your program by running it with the TestRectangle class, which is found on the Labs section of the
course web site.](https://content.bartleby.com/qna-images/question/600106ee-dc0f-4694-93fc-e1dd417feaa5/fdd96847-9fac-4334-b7e4-0a876041cdf2/upoaesr_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Q1. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { balance = balance + amount ; } // modified toString() method public String toString() { return "Account: " + actNum + "\tName: " + nameOnAct + "\tBalance: " + amount ; } } Q2. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { // scope of amount starts here balance = balance + amount ; // scope of amount ends here } public void processCheck( int amount ) { // scope of amount starts here int charge; incrementUse(); if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // scope of amount ends here }…arrow_forwardpublic class KnowledgeCheckTrek { public static final String TNG = "The Next Generation"; public static final String DS9 = "Deep Space Nine"; public static final String VOYAGER = "Voyager"; public static String trek(String character) { if (character != null && (character.equalsIgnoreCase("Picard") || character.equalsIgnoreCase("Data"))) { // IF ONE return TNG; } else if (character != null && character.contains("7")) { // IF TWO return VOYAGER; } else if ((character.contains("Quark") || character.contains("Odo"))) { // IF THREE return DS9; } return null; } public static void main(String[] args) { System.out.println(trek("Captain Picard")); System.out.println(trek("7 of Nine")); System.out.println(trek("Odo")); System.out.println(trek("Quark")); System.out.println(trek("Data").equalsIgnoreCase(TNG));…arrow_forwardHow do I fix the Java code errors? Code: //import java.util.Arrays;//Movie.java package movie; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; public Movie() { movieName = "Flick"; numMinutes = 0; isKidFriendly = false; numCastMembers = 0; castMembers = new String[10]; } public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; this.numCastMembers = castMembers.length; this.castMembers = castMembers; } public String getMovieName() { return this.movieName; } public int getNumMinutes() { return this.numMinutes; } public boolean getIsKidFriendly() { return this.isKidFriendly; } public boolean isKidFriendly() {…arrow_forward
- 1. Insert the missing part of the code below to output the string. public class MyClass { public static void main(String[] args) { ("Hello, I am a Computer Engineer");arrow_forward// SuperMarket.java - This program creates a report that lists weekly hours worked // by employees of a supermarket. The report lists total hours for // each day of one week. // Input: Interactive // Output: Report. import java.util.Scanner; public class SuperMarket { public static void main(String args[]) { // Declare variables. final String HEAD1 = "WEEKLY HOURS WORKED"; final String DAY_FOOTER = " Day Total "; // Leading spaces are intentional. final String SENTINEL = "done"; // Named constant for sentinel value. double hoursWorked = 0; // Current record hours. String hoursWorkedString = ""; // String version of hours String dayOfWeek; // Current record day of week. double hoursTotal = 0; // Hours total for a day. String prevDay = ""; // Previous day of week. boolean done = false; // loop control Scanner input = new…arrow_forwardx = 3 + numbers[3]++; System.out.println(x); QUESTION 2: Class Rectangle has two data members, length (type of float) and width (type of float). The class Rectangle also has the following: //mutator methods void setlength (float len) } //B. length = len; H accessor methods float getLength() I return length; The following statement creates an array of Rectangle with size 2: Rectangle[] arrayRectangle= new Rectangle[2]; Is it correct if executing the following statements? What is the output? If it is not correct, write the correct one arrayRectangle. setLength (12.59); System.out.printin[rectangle Set.getLength()); arrayRectangle[1].setLength(4.15); System.out.println(arrayRectangle[1].getLength()): QUESTION 3: Use the following int array int[] numbers = { 42, 28, 36, 72, 17, 25, 81, 65, 23, 58} -Open file data.txt to write. to write the values of the array numbers to the file with the y numbers to get the valuesarrow_forward
- complete the missing code. public class Exercise09_04Extra { public static void main(String[] args) { SimpleTime time = new SimpleTime(); time.hour = 2; time.minute = 3; time.second = 4; System.out.println("Hour: " + time.hour + " Minute: " + time.minute + " Second: " + time.second); } } class SimpleTime { // Complete the code to declare the data fields hour, // minute, and second of the int type }arrow_forwardimport java.util.Scanner;class BloodData { private static String bloodType; private static String rhFactor; public BloodData() { bloodType="O"; rhFactor="+"; } public BloodData(String bt, String rh) { bloodType = bt; rhFactor = rh; } public void display() { System.out.println(bloodType+rhFactor+" is added to the blood bank."); //prints message }} public class Main { public static void main(String[] args) { Scanner sc=new Scanner(System.in); //create Scanner instance System.out.print("Enter blood type of patient: "); String input1=sc.nextLine(); //accept input from user System.out.print("Enter the Rhesus factor (+ or -): "); String input2=sc.nextLine(); //accept input from user BloodData bd; //create instance if("".equals(input1) || "".equals(input2)) //if any of inputs is blank bd=new BloodData(); //allocates memory using default constructor else //if valid inputs bd=new BloodData(input1,input2); //allocates memory using parameterized constructor…arrow_forwardJAva Input. class increment { public static void main(String args[]) { double var1 = 1 + 5; double var2 = var1 / 4; int var3 = 1 + 5; int var4 = var3 / 4; System.out.print(var2 + " " + var4); } } Find output.arrow_forward
- PROBLEM STATEMENT: Return the longest String! public class LongestString{public static String solution(String firstWord, String secondWord){// ↓↓↓↓ your code goes here ↓↓↓↓return null;}} Can you help me with this Java Questionarrow_forwardQ: What's a better way to write this? (C#) public string SomeMethod(string OriginalZip) { string zip = "Zip Not Available"; zip = GetZip(zip, OriginalZip); return zip; } private static string GetZip(string zip, string OriginalZip) { int zipLength = (OriginalZip.HasValue) ? OriginalZip.Value.ToString().Length: 0; int NumOf Leading Zeroes = (zipLength > O && zipLength < 5) ? 5 - zipLength: 0; if (OriginalZip.HasValue) { i++) } zip = OriginalZip.Value.ToString(); for (int i = 0; i < NumOf Leading Zeroes; { } zip = "0" + zip; } return zip;arrow_forwardpublic class Ex08FanTest { public static void main (String[] args){ Ex08Fan fan1= new Ex08Fan(); Ex08Fan fan2= new Ex08Fan(); fan1.setOn(true); fan1.setSpeed(3); fan1.setRadius(10); fan1.setColor("yellow"); fan2.setOn(true); fan2.setSpeed(2); fan2.setRadius(5); fan2.setColor("blue"); fan2.setOn(false); System.out.println("Fan 1:\n" + fan1.toString()); System.out.println("\nFan 2:\n" + fan2.toString()); }}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
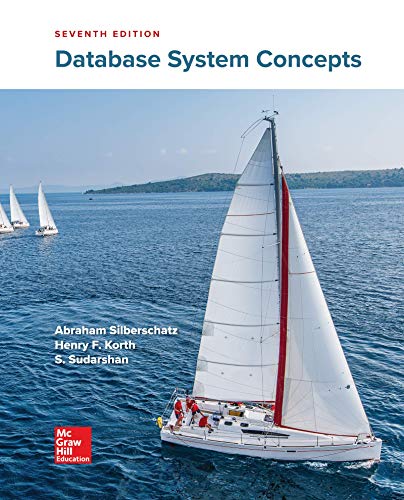
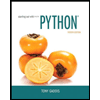
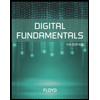
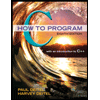
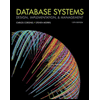
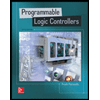