dd(60) will return 5 as it is the fifth position in the list. int remove(int num) This will remove the first instance of the element and return the position of the removed element. In the above example, having remove(40) will return 3 as 40 was the third element in the linked list before having removed.
TOPIC: Singly Linked List
Implement the following functions using C++
- int add(int num)
This will add the element num into the linked list and return the position of the newly-added element. In the above example, having add(60) will return 5 as it is the fifth position in the list.
- int remove(int num)
This will remove the first instance of the element and return the position of the removed element. In the above example, having remove(40) will return 3 as 40 was the third element in the linked list before having removed.
- int get(int pos)
This method will get the element at the specified position. This will return -1 if the specified position is invalid.
- bool addAt(int num, int pos)
This method will add the integer num to the posth position of the list and returns true if the value of pos is valid.
Performing addAt(20, 2) in the example list will add 20 at the 2nd position and the linked list will now look like this: 10 -> 20 -> 30 -> 40 -> 50.
When the value of pos is invalid i.e. greater than the size + 1 or less than one, return false.
Now, you also have to improve the following functions:
- int removeAt(int pos)
Removes the number in the posth position of the list and returns the element removed.
Performing removeAt(3) in the example list will remove the 3rd element of the linked list and the updated list will be: 10 -> 30 -> 50
When the value of pos is greater than the size or less than one, return -1.
- int removeAll(int num)
Removes all instances of num in the linked list and returns the number of instances removed.
In this list 10 -> 10 -> 20 -> 30 -> 10, performing removeAll(10) will remove all three 10's and the list will look like this: 20 -> 30. Then, it will return the number of instances removed, in this case, 3.
- int contains(int num)
This will return the position of the first instance of the element num in the list. If num is not found, return 0. In the example, having the method contains(30) will return 2 as it is located in the second position.
- int count(int num)
This will return the count of the instances of the element num in the list. In the linked list in removeAll method, having the method count(10) will return 3 as there are three 10's in the linked list.
Additionally, you are to implement these methods:
- bool move(int pos1, int pos2)
This method will move the element at position pos1 to the position specified as pos2 and will return true if the move is successful. This will return false if either the specified positions is invalid. In the example stated, operating the method move(1, 3) will move the 1st element and shall now become the 3rd element. The linked list shall now look like: 30 -> 40 -> 10 -> 50
- int removeTail()
This method will remove the last element in your linked list and return the said element. If the list is empty, return 0. You can use the existing methods.
- void isEmpty()
This method will return true if the linked list is empty, otherwise return false.
- void clear()
This method will empty your linked list. Effectively, this should and already has been called in your destructor (i.e., the ~LinkedList() method) so that it will deallocate the nodes created first before deallocating the linked list itself.

Step by step
Solved in 2 steps

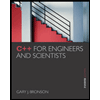
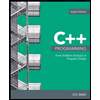
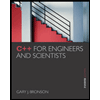
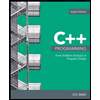