Description Write the code for the function find_sub which takes as parameter a list input_list and finds the sub-list with the maximum sum inside the list. For example, given the list [-3, -2, 5, 2, -7], the sub-list with the largest sum is [5, 2] with the sum 7. The list is to be taken from the user. In the script, input part is handled for you, along with formatting, so you don't need to do anything about it. You must use the variable Ist. It is assigned the list you will work with. The function is also called at the end, you must handle the printing inside the function. The input list will always be one dimensional, it will contain only valid float or integer elements; but an empty list can be given as input, in which case you should print an empty list as output, like in the examples. If there is an equality of maximum sum, print the first occurring sub-list. Check the examples for further clarification. Keep in mind that we will be grading your code not just based on these examples, but other cases as well, so try to write code which can handle all possible cases. You are encouraged to check the code we provided, something called list comprehension is used in it. You are not responsible from it, but it is something used to build lists on the fly. Definition: A sub-list is a list within the input list which contains contiguous elements. For example, given the list [-3, -2, 5, 2, -7], [-3, -2, 5] is a sub-list but [-3, -2, 2] is not because -3 and 2 are not next to each other in the given list.
Description Write the code for the function find_sub which takes as parameter a list input_list and finds the sub-list with the maximum sum inside the list. For example, given the list [-3, -2, 5, 2, -7], the sub-list with the largest sum is [5, 2] with the sum 7. The list is to be taken from the user. In the script, input part is handled for you, along with formatting, so you don't need to do anything about it. You must use the variable Ist. It is assigned the list you will work with. The function is also called at the end, you must handle the printing inside the function. The input list will always be one dimensional, it will contain only valid float or integer elements; but an empty list can be given as input, in which case you should print an empty list as output, like in the examples. If there is an equality of maximum sum, print the first occurring sub-list. Check the examples for further clarification. Keep in mind that we will be grading your code not just based on these examples, but other cases as well, so try to write code which can handle all possible cases. You are encouraged to check the code we provided, something called list comprehension is used in it. You are not responsible from it, but it is something used to build lists on the fly. Definition: A sub-list is a list within the input list which contains contiguous elements. For example, given the list [-3, -2, 5, 2, -7], [-3, -2, 5] is a sub-list but [-3, -2, 2] is not because -3 and 2 are not next to each other in the given list.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 18PE
Related questions
Question
![Description
Write the code for the function find_sub which takes as parameter a list input_list and finds the sub-list with the maximum sum inside the list. For example, given the list [-3, -2, 5, 2, -7], the sub-list with the largest
sum is [5, 2] with the sum 7.
The list is to be taken from the user. In the script, input part is handled for you, along with formatting, so you don't need to do anything about it.
You must use the variable Ist. It is assigned the list you will work with.
The function is also called at the end, you must handle the printing inside the function.
The input list will always be one dimensional, it will contain only valid float or integer elements; but an empty list can be given as input, in which case you should print an empty list as output, like in the examples.
If there is an equality of maximum sum, print the first occurring sub-list.
Check the examples for further clarification. Keep in mind that we will be grading your code not just based on these examples, but other cases as well, so try to write code which can handle all possible cases.
You are encouraged to check the code we provided, something called list comprehension is used in it. You are not responsible from it, but it is something used to build lists on the fly.
Definition: A sub-list is a list within the input list which contains contiguous elements. For example, given the list [-3, -2, 5, 2, -7], [-3, -2, 5] is a sub-list but [-3, -2, 2] is not because -3 and 2 are not next to each other in
the given list.
A sub-list should contain at least one number if the input_list is not empty.
Important: The function should print the maximum sub-list only and exactly as given in the examples.
Hint: You can use multiple for loops and indexes, think about how to iterate over all the combinations of possible sub-lists.
Warning: You are not allowed to use imports, or anything that has not been covered this semester.
Examples:
INPUT
OUTPUT
[0.282, -0.262, -0.477, 0.462, 0.400, 0.422, -0.279, 0.074, -0.251, 0.371, -0.548, -0.463, 0.103, 0.154, 0.095, 0.029, 0.067, 0.493, -0.232, 0.421] [0.462, 0.4, 0.422, -0.279, 0.074, -0.251, 0.371, -0.548, -0.463, 0.103, 0.154, 0.095, 0.029, 0.067, 0.493, -0.232, 0.421]
[-1.6, -2.73, 4.19, -1.2, 5.12, -3]
[4.19, -1.2, 5.12]
[-5.23]
[-5.23]
[-3, 2.1]
[2.1]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe530ec5b-9ac6-4690-b2cc-e6d60d27c8b8%2F4cd47b22-0860-4612-ab5d-32dbd1d291b7%2Fj9a5tu_processed.png&w=3840&q=75)
Transcribed Image Text:Description
Write the code for the function find_sub which takes as parameter a list input_list and finds the sub-list with the maximum sum inside the list. For example, given the list [-3, -2, 5, 2, -7], the sub-list with the largest
sum is [5, 2] with the sum 7.
The list is to be taken from the user. In the script, input part is handled for you, along with formatting, so you don't need to do anything about it.
You must use the variable Ist. It is assigned the list you will work with.
The function is also called at the end, you must handle the printing inside the function.
The input list will always be one dimensional, it will contain only valid float or integer elements; but an empty list can be given as input, in which case you should print an empty list as output, like in the examples.
If there is an equality of maximum sum, print the first occurring sub-list.
Check the examples for further clarification. Keep in mind that we will be grading your code not just based on these examples, but other cases as well, so try to write code which can handle all possible cases.
You are encouraged to check the code we provided, something called list comprehension is used in it. You are not responsible from it, but it is something used to build lists on the fly.
Definition: A sub-list is a list within the input list which contains contiguous elements. For example, given the list [-3, -2, 5, 2, -7], [-3, -2, 5] is a sub-list but [-3, -2, 2] is not because -3 and 2 are not next to each other in
the given list.
A sub-list should contain at least one number if the input_list is not empty.
Important: The function should print the maximum sub-list only and exactly as given in the examples.
Hint: You can use multiple for loops and indexes, think about how to iterate over all the combinations of possible sub-lists.
Warning: You are not allowed to use imports, or anything that has not been covered this semester.
Examples:
INPUT
OUTPUT
[0.282, -0.262, -0.477, 0.462, 0.400, 0.422, -0.279, 0.074, -0.251, 0.371, -0.548, -0.463, 0.103, 0.154, 0.095, 0.029, 0.067, 0.493, -0.232, 0.421] [0.462, 0.4, 0.422, -0.279, 0.074, -0.251, 0.371, -0.548, -0.463, 0.103, 0.154, 0.095, 0.029, 0.067, 0.493, -0.232, 0.421]
[-1.6, -2.73, 4.19, -1.2, 5.12, -3]
[4.19, -1.2, 5.12]
[-5.23]
[-5.23]
[-3, 2.1]
[2.1]
![Edef read_list(param):
p = param.replace(',',
p = p[1:-1]
p.split()
')
li =
while('' in li):
li.remove('')
li =
[int(elem) if '
not in elem else float(elem) for elem in li]
return li
lst = read_list(input())
def find_sub(input_list):
# DO_NOT_EDIT_ANYTHING_ABOVE_THIS_LINE
A# DO_NOT_EDIT_ANYTHING_BELOW_THIS_LINE
return
find_sub(lst)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe530ec5b-9ac6-4690-b2cc-e6d60d27c8b8%2F4cd47b22-0860-4612-ab5d-32dbd1d291b7%2Fv44os39_processed.png&w=3840&q=75)
Transcribed Image Text:Edef read_list(param):
p = param.replace(',',
p = p[1:-1]
p.split()
')
li =
while('' in li):
li.remove('')
li =
[int(elem) if '
not in elem else float(elem) for elem in li]
return li
lst = read_list(input())
def find_sub(input_list):
# DO_NOT_EDIT_ANYTHING_ABOVE_THIS_LINE
A# DO_NOT_EDIT_ANYTHING_BELOW_THIS_LINE
return
find_sub(lst)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
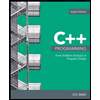
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
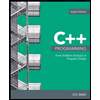
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning