Declare a two-dimensional char array that is 25 by 25 that represents the entire ocean and an If statement that prints “HIT” if a torpedo hits a ship given the coordinates X and Y. Write a C++ program that will read in a file representing a game board with 25 lines where each line has 25 characters corresponding to the description above. An example file might look like: ~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~#####~~~~~~~~~~~~~~ ~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~~~~~~~~~#~~~~~~~~~~ ~~~~~~~~~~~~~~#~~~~~~~~~~ ~~~~~~~~~~~~~~#~~~~~~~~~~ ~~~~~~~~~~~~~~#~~~~~~~~~~ ~~~~~~~~~~~~~~#~~~~~~~~~~ ~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~~~~~~~~#####~~~~~~~ ~~~~~~~~~~~~~~~~~~~~~~~~~ ~######~~~~~~~~~~~~~~~~~~ ~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~~~~~#####~~~~~~~~~~ ~~~~~~~~~~~~~~~~~~~#~~~~~ ~~~~~~~~~~~~~~~~~~~#~~~~~ ~~~~~~~~~~~~~~~~~~~#~~~~~ ~~~~~~~~~~~~~~~~~~~#~~~~~ ~~~######~~~~~~~~~~#~~~~~ ~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~~~~~~~~~~~~~~~~~~~~ ~~~~~~~~~~~~~~~~~~~~~~~~~ It wont appear. Code is below: /*Imagine we are using a two-dimensional array as the basis for creating the game battle- ship. In the game of battleship a ‘~’ character entry in the array represents ocean (i.e., not a ship), a ‘#’ character represents a place in the ocean where part of a ship is present, and a ‘H’ character represents a place in the ocean where part of a ship is present and has been hit by a torpedo. Thus, a ship with all ‘H’ characters means the ship has been sunk. Declare a two-dimensional char array that is 25 by 25 that represents the entire ocean and an If statement that prints “HIT” if a torpedo hits a ship given the coordinates X and Y. Write a C++ program that will read in a file representing a game board with 25 lines where each line has 25 characters corresponding to the description above. You should write a function called Fire that will take an X and Y coordinate and print “HIT” if a ship is hit and “MISS” if a ship is missed. If a ship is HIT you should update the array with an ‘H’ character to indicate the ship was hit. If a ship is hit that has already been hit at that location you should print “HIT AGAIN”. You should write a second function called FleetSunk that will determine if all the ships have been sunk. Your C++ program must then call these functions until all the ships have been sunk at which point the program should display “The fleet was destroyed!”. Your game loop logic (the main logic of a game) should like like the following: do {Fire(x, y, gameBoard);} while(!FleetSunk(gameBoard));*/ #include <iostream>#include <fstream>#include <string.h>#include <windows.h> using namespace std; //function prototypesvoid Fire(char board[][25]);void FleetSunk(char board[][25], int&); int main(){//In cout statement below substitute your name and lab numbercout << "Jaime Ross -- Lab 8" << endl << endl;//Variable declarationschar board[25][25];ifstream infile;infile.open("board.dat");//Program logicif (!infile){cout << "Cannot open file." << endl;}for (int i = 0; i < 25; i++){for (int j = 0; j < 25; j++){char bs;infile >> bs;board[i][j] = bs;}}int fs = 0;do{Fire(board);FleetSunk(board, fs);} while (fs == 0);system("pause");return 0;}void Fire(char board[25][25]){int row = 0, row1 = 0;int col = 0, col1 = 0; cout << "\nEnter row and column you want to hit: ";cin >> row1;cin >> col1;row = row1 - 1;col = col1 - 1;switch (board[row][col]){case '#':if (board[row - 1][col] == 'H'){cout << "HIT AGAIN" << endl;board[row][col] = 'H';}else if (board[row + 1][col] == 'H'){cout << "HIT AGAIN" << endl;board[row][col] = 'H';}else if (board[row][col - 1] == 'H'){cout << "HIT AGAIN" << endl;board[row][col] = 'H';}else if (board[row][col + 1] == 'H'){cout << "HIT AGAIN" << endl;board[row][col] = 'H';}else{cout << "HIT" << endl;board[row][col] = 'H';}break; case '~':cout << "MISS" << endl;break; case 'H':cout << "You already destroyed this ship! :" << endl;break;}}void FleetSunk(char board[25][25], int& fs){//checking if all ships have sunkfor (int i = 0; i < 25; i++){for (int j = 0; j < 25; j++){if (board[i][j] == '#'){fs = 0;return;}}}cout << "The last fleet has been destoryed." << endl;fs = 1;}
Declare a two-dimensional char array that is 25 by 25 that represents the entire ocean and an If statement that prints “HIT” if a torpedo hits a ship given the coordinates X and Y. Write a C++ program that will read in a file representing a game board with 25 lines where each line has 25 characters corresponding to the description above. An example file might look like:
~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~#####~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~#~~~~~~~~~~
~~~~~~~~~~~~~~#~~~~~~~~~~
~~~~~~~~~~~~~~#~~~~~~~~~~
~~~~~~~~~~~~~~#~~~~~~~~~~
~~~~~~~~~~~~~~#~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~#####~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~
~######~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~#####~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~#~~~~~
~~~~~~~~~~~~~~~~~~~#~~~~~
~~~~~~~~~~~~~~~~~~~#~~~~~
~~~~~~~~~~~~~~~~~~~#~~~~~
~~~######~~~~~~~~~~#~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~
~~~~~~~~~~~~~~~~~~~~~~~~~
It wont appear.
Code is below:
/*
Imagine we are using a two-dimensional array as the basis for creating the game battle- ship. In the game of battleship a ‘~’ character entry in the array represents ocean (i.e., not a ship), a ‘#’ character represents a place in the ocean where part of a ship is present, and a ‘H’ character represents a place in the ocean where part of a ship is present and has been hit by a torpedo. Thus, a ship with all ‘H’ characters means the ship has been sunk. Declare a two-dimensional char array that is 25 by 25 that represents the entire ocean and an If statement that prints “HIT” if a torpedo hits a ship given the coordinates X and Y. Write a C++ program that will read in a file representing a game board with 25 lines where each line has 25 characters corresponding to the description above.
You should write a function called Fire that will take an X and Y coordinate and print “HIT” if a ship is hit and “MISS” if a ship is missed. If a ship is HIT you should update the array with an ‘H’ character to indicate the ship was hit. If a ship is hit that has already been hit at that location you should print “HIT AGAIN”. You should write a second function called FleetSunk that will determine if all the ships have been sunk. Your C++ program must then call these functions until all the ships have been sunk at which point the program should display “The fleet was destroyed!”.
Your game loop logic (the main logic of a game) should like like the following:
do {
Fire(x, y, gameBoard);
} while(!FleetSunk(gameBoard));
*/
#include <iostream>
#include <fstream>
#include <string.h>
#include <windows.h>
using namespace std;
//function prototypes
void Fire(char board[][25]);
void FleetSunk(char board[][25], int&);
int main()
{
//In cout statement below substitute your name and lab number
cout << "Jaime Ross -- Lab 8" << endl << endl;
//Variable declarations
char board[25][25];
ifstream infile;
infile.open("board.dat");
//Program logic
if (!infile)
{
cout << "Cannot open file." << endl;
}
for (int i = 0; i < 25; i++)
{
for (int j = 0; j < 25; j++)
{
char bs;
infile >> bs;
board[i][j] = bs;
}
}
int fs = 0;
do
{
Fire(board);
FleetSunk(board, fs);
} while (fs == 0);
system("pause");
return 0;
}
void Fire(char board[25][25])
{
int row = 0, row1 = 0;
int col = 0, col1 = 0;
cout << "\nEnter row and column you want to hit: ";
cin >> row1;
cin >> col1;
row = row1 - 1;
col = col1 - 1;
switch (board[row][col])
{
case '#':
if (board[row - 1][col] == 'H')
{
cout << "HIT AGAIN" << endl;
board[row][col] = 'H';
}
else if (board[row + 1][col] == 'H')
{
cout << "HIT AGAIN" << endl;
board[row][col] = 'H';
}
else if (board[row][col - 1] == 'H')
{
cout << "HIT AGAIN" << endl;
board[row][col] = 'H';
}
else if (board[row][col + 1] == 'H')
{
cout << "HIT AGAIN" << endl;
board[row][col] = 'H';
}
else
{
cout << "HIT" << endl;
board[row][col] = 'H';
}
break;
case '~':
cout << "MISS" << endl;
break;
case 'H':
cout << "You already destroyed this ship! :" << endl;
break;
}
}
void FleetSunk(char board[25][25], int& fs)
{
//checking if all ships have sunk
for (int i = 0; i < 25; i++)
{
for (int j = 0; j < 25; j++)
{
if (board[i][j] == '#')
{
fs = 0;
return;
}
}
}
cout << "The last fleet has been destoryed." << endl;
fs = 1;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

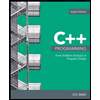
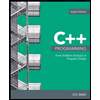