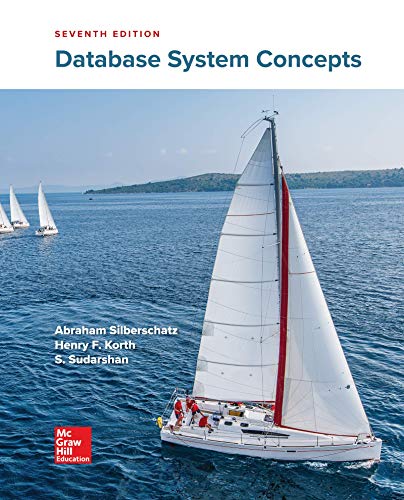
Concept explainers
Create in Java
(1) Create an array of String objects and populate it with the days of the week (7 days)(i.e., Monday - Sunday)
(2) Create an array parallel to the one created in (1) to store the entrées. However, you do not have this information, so
you will need to write a loop that prompts the user to enter the entrée served on that day. This prompt should
include the day for which you are asking for the information (e.g., "What entrée is being served on Monday?")
(3) Create another parallel array that stores the prices. You will also need to collect this information from the user. This
time, your prompt should include the name of the entrée for which you are asking for the price
(4) Prompt the user to choose an entrée from a list that you display numbered 1 through 7 (have them input a number
1 to 7) and search the array and output the day on which the entrée will be served
(5) Search through the array for the highest price entrée and output the name of this entrée for the user including the
text something like “The highest priced entrée is Salmon”.
See attached example of the output
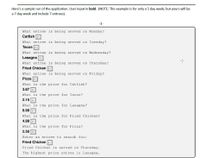

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- TASK 1: Write a Java program that do the following: [10 Marks] 1. Define and create an ArrayList which is called "itemsList" of Integer objects. [1 mark] 2. Define and create an array which is called "numList" with five integer vales. [1 mark] 3. Copy the five values from the array "numList" o ArrayList using a for loop. [2 marks] 4. Display the contents of the ArrayList with a message using the for loop with the get method. marks] 5. Display the size of ArrayList with a message. [1.5 mark] 6. Find the sum of ArrayList. [3 marks] [1.5arrow_forward1. Array splitting in Java Create an integer array with 10 integer numbers, initialize the array arbitrarily but make sure there are both positive and negative integers. Then generate two arrays out of the original array, one array with all natural numbers (positive numbers or zeros) and another one with all negative numbers. Print out the number of elements and the detailed elements in each array.arrow_forwardProblem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forward
- in java Integer numVals is read from input and integer array userCounts is declared with size numVals. Then, numVals integers are read from input and stored into userCounts. If the first element is less than the last element, then assign Boolean firstSmaller with true. Otherwise, assign firstSmaller with false. Ex: If the input is: 5 40 22 41 84 77 then the output is: First element is less than last element 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 import java.util.Scanner; public class UserTracker { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intnumVals; int[] userCounts; inti; booleanfirstSmaller; numVals=scnr.nextInt(); userCounts=newint[numVals]; for (i=0; i<userCounts.length; ++i) { userCounts[i] =scnr.nextInt(); } /* Your code goes here */ if (firstSmaller) { System.out.println("First element is less than last element"); } else { System.out.println("First element is not less…arrow_forwardIn this project you will generate a poker hand containing five cards randomly selected from a deck of cards. The names of the cards are stored in a text string will be converted into an array. The array will be randomly sorted to "shuffle" the deck. Each time the user clicks a Deal button, the last five cards of the array will be removed, reducing the size of the deck size. When the size of the deck drops to zero, a new randomly sorted deck will be generated. A preview of the completed project with a randomly generated hand is shown in Figure 7-50.arrow_forwardThis is needed in Javaarrow_forward
- In Java Assignment 5B : Maze Game! 2D Arrays can be used to store and represent informationabout video game levels or boards. In this exercise, you will use this knowledge tocreate an interactive game where players attempt to move through a maze. You willstart by creating a pre-defined 2D array with the following values:{"_","X","_","X","X"}{"_","X","_","X","W"}{"_","_","_","X","_"}{"X","X","_","_","_"}{"_","_","_","X","X"}You will then set the player (represented by “O”) at index 0, 0 of the array, the top-leftcorner of the maze. You will use a loop to repeatedly prompt the user to enter adirection (“Left”, “Right”, “Up”, or “Down”). Based on these directions, you will try tomove the player.• If the location is valid (represented by “_”), you will move the player there• If the location is out of bounds (e.g. index 0, -1) or the command is invalid, youwill inform the player and prompt them to enter another direction• If the location is a wall (represented by “X”), you will tell the…arrow_forwardstrobogrammatic number is a number that looks the same when rotated 180 degrees (looked at upside down). Find all strobogrammatic numbers that are of length = n. For example, Given n = 2, return ["11","69","88","96"]. def gen_strobogrammatic(n): Given n, generate all strobogrammatic numbers of length n. :type n: int :rtype: List[str] return helper(n, n) def helper(n, length): if n == 0: return [™"] if n == 1: return ["1", "0", "8"] middles = helper(n-2, length) result = [] for middle in middles: if n != length: result.append("0" + middle + "0") result.append("8" + middle + "8") result.append("1" + middle + "1") result.append("9" + middle + "6") result.append("6" + middle + "9") return result def strobogrammatic_in_range(low, high): :type low: str :type high: str :rtype: int res = count = 0 low_len = len(low) high_len = len(high) for i in range(low_len, high_len + 1): res.extend(helper2(i, i)) for perm in res: if len(perm) == low_len and int(perm) int(high): continue count += 1 return…arrow_forwardplease write in javaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
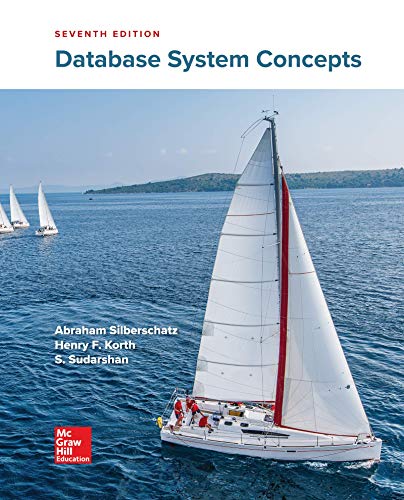
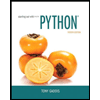
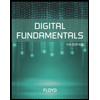
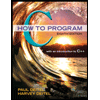
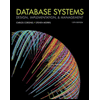
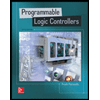