def animals(animal_dict, target): """ Filter animals based on the target category. :param animal_dict: A dictionary mapping animals to their categories. :type animal_dict: dict :param target: The target category to filter animals. :type target: str :return: A list of animals belonging to the target category. :rtype: list animals_dict = {"dogs": "mammals", "snakes": "reptiles", \ "dolphins": "mammals", "sharks": "fish"} >>> target = "mammals" >>> animals(animals_dict, target) ['dogs', 'dolphins'] >>> animals_dict = {True: "mammals"} >>> animals(animals_dict, target) # This will raise an AssertionError Traceback (most recent call last): ... AssertionError: Target should be provided and not None """ assert target is not None, "Target should be provided and not None" assert isinstance(animal_dict, dict) assert all(isinstance(animal, str) for animal in animal_dict.keys()) assert all(isinstance(category, str) for category in animal_dict.values()) filtered_animals = [animal for animal, value in animal_dict.items() if target.lower() == value.lower()] assert isinstance(filtered_animals, list) return filtered_animals # Intentionally do not define the target variable # This will raise an AssertionError animals_dict = {True: "mammals"} animals(animals_dict, target) fix my code to assert >>> animals_dict = {True: "mammals"} >>> animals(animals_dict, target) # This will raise an AssertionError Traceback (most recent call last): ... AssertionError: Target should be provided and not None Requirements: Assert statements, List Comprehension no for loop or any built in fuctions getting this error ERROR! Traceback (most recent call last): File "", line 38, in NameError: name 'target' is not defined
def animals(animal_dict, target):
"""
Filter animals based on the target category.
:param animal_dict: A dictionary mapping animals to their categories.
:type animal_dict: dict
:param target: The target category to filter animals.
:type target: str
:return: A list of animals belonging to the target category.
:rtype: list
animals_dict = {"dogs": "mammals", "snakes": "reptiles", \
"dolphins": "mammals", "sharks": "fish"}
>>> target = "mammals"
>>> animals(animals_dict, target)
['dogs', 'dolphins']
>>> animals_dict = {True: "mammals"}
>>> animals(animals_dict, target) # This will raise an AssertionError
Traceback (most recent call last):
...
AssertionError: Target should be provided and not None
"""
assert target is not None, "Target should be provided and not None"
assert isinstance(animal_dict, dict)
assert all(isinstance(animal, str) for animal in animal_dict.keys())
assert all(isinstance(category, str) for category in animal_dict.values())
filtered_animals = [animal for animal, value in animal_dict.items() if target.lower() == value.lower()]
assert isinstance(filtered_animals, list)
return filtered_animals
# Intentionally do not define the target variable
# This will raise an AssertionError
animals_dict = {True: "mammals"}
animals(animals_dict, target)
fix my code to assert >>> animals_dict = {True: "mammals"}
>>> animals(animals_dict, target) # This will raise an AssertionError
Traceback (most recent call last):
...
AssertionError: Target should be provided and not None
Requirements: Assert statements, List Comprehension no for loop or any built in fuctions getting this error ERROR!
Traceback (most recent call last):
File "<string>", line 38, in <module>
NameError: name 'target' is not defined
>

Step by step
Solved in 4 steps with 1 images

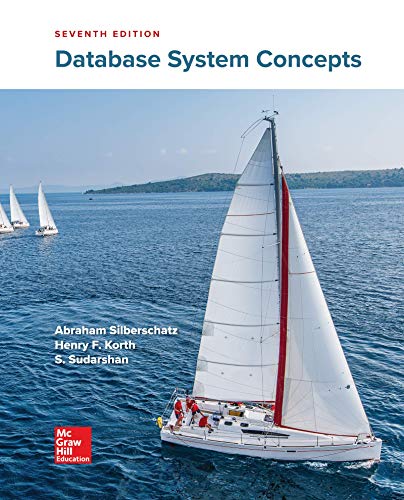
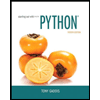
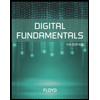
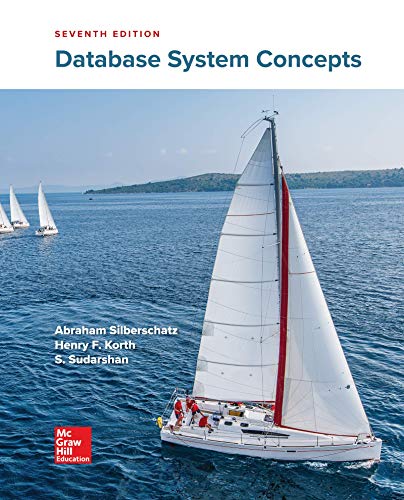
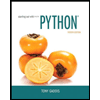
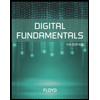
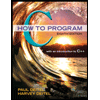
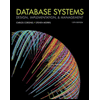
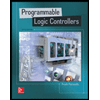