Description: Create a class named ComparableDog that extends Animal and implements Comparable interface. Implement the compareTo method to compare the Dogs on the basis of age. Write a test class to find the bigger age of two instances of ComparableDog Objects, and complement the ComparableDog class with the missing part. 1) The test program, the ComparableDog class, and its super class Animal are given as follows.
Description:
Create a class named ComparableDog that extends Animal and implements Comparable interface.
Implement the compareTo method to compare the Dogs on the basis of age.
Write a test class to find the bigger age of two instances of ComparableDog Objects, and complement the ComparableDog class with the missing part.
1) The test program, the ComparableDog class, and its super class Animal are given as follows.
// Main method
public static void main(String[] args) {
// Create two comparable Dogs
ComparableDog dog1 = new ComparableDog(3);
ComparableDog dog2 = new ComparableDog(4);
System.out.println("Dog1:" + dog1);
System.out.println("Dog2:" + dog2);
if(dog1.compareTo(dog2) == 1)
System.out.println("Dog1 is older than Dog2");
else if(dog1.compareTo(dog2) == -1)
System.out.println("Dog1 is younger than Dog2");
else
System.out.println("Dog1 and Dog2 have the same age");
}
class ComparableDog extends Animal implements Comparable<ComparableDog> {
private int age;
public ComparableDog() {
}
/* Print the Dog info */
public void printDog() {
System.out.println("The Dog is created " + getDateCreated() +
" the age is " + age);
}
public int getAge(){
return age;
}
public int compareTo(ComparableDog d)
{
}
public String toString() {
}
}
abstract class Animal {
private String color = "white";
private java.util.Date dateCreated;
protected Animal() {
dateCreated = new java.util.Date();
}
/** Construct an animal object with color value */
protected Animal(String color, boolean filled) {
dateCreated = new java.util.Date();
this.color = color;
}
/** Return color */
public String getColor() {
return color;
}
/** Set a new color */
public void setColor(String color) {
this.color = color;
}
/** Get dateCreated */
public java.util.Date getDateCreated() {
return dateCreated;
}
/** Return a string representation of this object */
public String toString() {
return "created on " + dateCreated + "\ncolor: " + color;
}
public abstract int getAge();
}
Output:
Dog1: color: white, age: 3
Dog2: color: white, age: 4
Dog1 is younger than Dog2

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

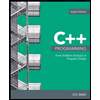
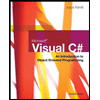
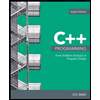
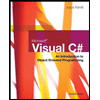