Design a class named Author with the following members: A field for the author’s name (a String) A field for the author’s year of birth (an int) One or more constructors and appropriate accessor and mutator methods A toString method that displays the author’s info as: Author: Mary Shelley (1797) Save the file as Author.java. Next, create an abstract class named Book with the following members: A field for the book title (a String) A field for the book author (a reference to an Author object) A field for the book price (a double) A constructor that requires the title and author Get methods for the title, author, and price An abstract method named setPrice A toString method that displays the book’s title, author’s info, and price as: Frankenstein Author: Mary Shelley (1797) Price: $23.95 Save the file as Book.java. Create Fiction and NonFiction child classes of Book. Each must include a setPrice method that sets the price for all Fiction books to $23.95 and for all NonFiction books to $39.95. Write a constructor for each subclass, and include a call to setPrice() within each. Include an appropriate toString method. Save the files as Fiction.java and NonFiction.java. Finally, write an application named BookArray in which you create an ArrayList that holds 10 Books, some Fiction and some NonFiction. Using a for loop, prompt the user for a title, author, and whether the Book is fiction or nonfiction (F or N). Create the appropriate book type and store it in the array. If the user does not select F or N, reprompt the user. In another for loop, display details about all 10 Books. Save the file as BookArray.java.
Design a class named Author with the following members:
- A field for the author’s name (a String)
- A field for the author’s year of birth (an int)
- One or more constructors and appropriate accessor and mutator methods
- A toString method that displays the author’s info as: Author: Mary Shelley (1797)
Save the file as Author.java.
Next, create an abstract class named Book with the following members:
- A field for the book title (a String)
- A field for the book author (a reference to an Author object)
- A field for the book price (a double)
- A constructor that requires the title and author
- Get methods for the title, author, and price
- An abstract method named setPrice
- A toString method that displays the book’s title, author’s info, and price as: Frankenstein Author: Mary Shelley (1797) Price: $23.95
Save the file as Book.java.
Create Fiction and NonFiction child classes of Book. Each must include a setPrice method that sets the price for all Fiction books to $23.95 and for all NonFiction books to $39.95. Write a constructor for each subclass, and include a call to setPrice() within each. Include an appropriate toString method. Save the files as Fiction.java and NonFiction.java.
Finally, write an application named BookArray in which you create an ArrayList that holds 10 Books, some Fiction and some NonFiction. Using a for loop, prompt the user for a title, author, and whether the Book is fiction or nonfiction (F or N). Create the appropriate book type and store it in the array. If the user does not select F or N, reprompt the user. In another for loop, display details about all 10 Books. Save the file as BookArray.java.
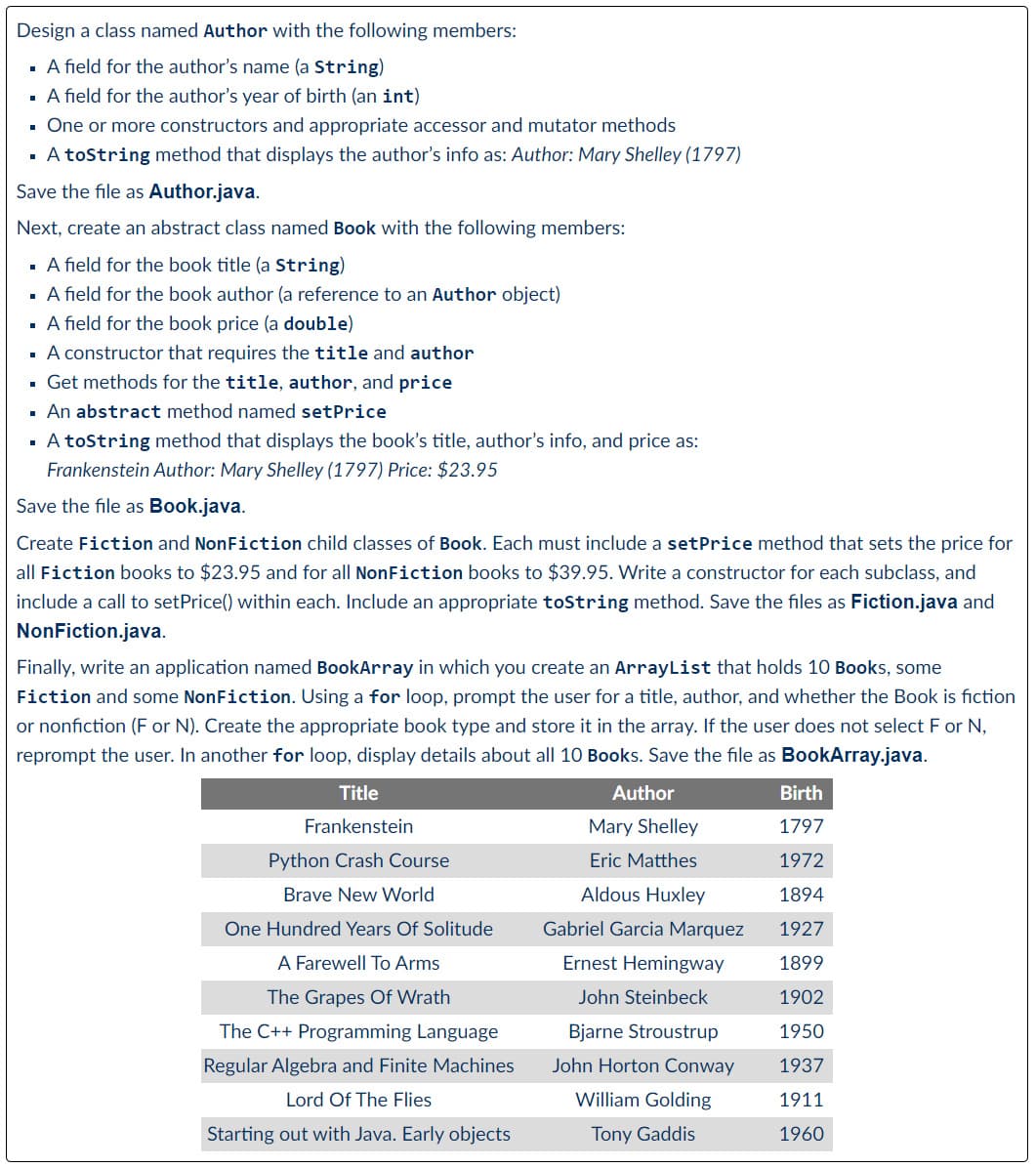
![C:\Users\Java\Desktop\MidTerm>java BookArray
Enter title: Frankenstein
Enter author name: Mary Shelley
Enter year of author's birth: 1797
Is this a [F]iction or [N]onfiction title: F
Enter title: Python Crash Course
Enter author name: Eric Matthes
Enter year of author's birth: 1972
Is this a [F]iction or [N]onfiction title: N
I
Fiction: Frankenstein Author: Mary Shelley (1797) Price: $23.95
NonFiction: Python Crash Course Author: Eric Matthes (1972) Price: $39.95
Fiction: Brave New World Author: Aldous Huxley (1894) Price: $23.95
Fiction: One Hundred Years Of Solitude Author: Gabriel Garcia MMarquez (1927) Price: $23.95
Fiction: A Farewell To Arms Author: Ernest Hemingway (1899) Price: $23.95
Fiction: The Grapes Of Wrath Author: John Steinbeck (1902) Price: $23.95
NonFiction: The C++ Programming Language Author: Bjarne Stroustrup (1950) Price: $39.95
NonFiction: Regular Algebra and Finite Machines Author: John Horton Conway (1937) Price: $39.95
Fiction: Lord Of The Flies Author: William Golding (1911) Price: $23.95
NonFiction: Starting Out with Java. Early objects Author: Tony Gaddis (1960) Price: $39.95](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F286de0ac-61cd-41f9-9a8b-990e2624e200%2Fc0cbcc6d-6b78-4495-bccf-4134c9f1aeaf%2Fh1oehnu_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

Getting a syntax error on the last closing brace of BookArray.java
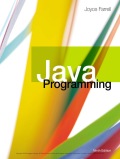
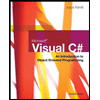
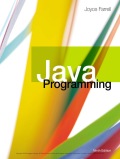
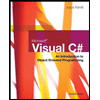