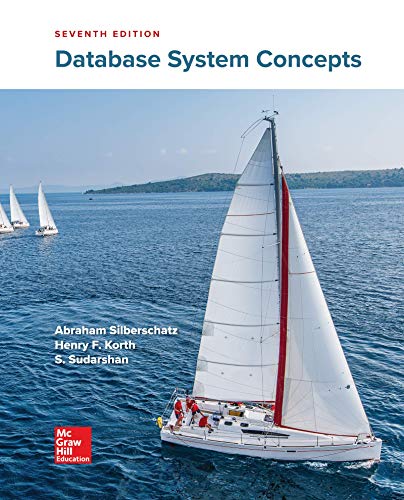
Concept explainers
Code in Java
Define a class named MobilePhone with the attributes brand name, model, color and price. Define 3 constructors - the first one is the default constructor, the second one initializes all data members and the third one gives an initial value to the brand name Apple, model iPhone XR and price to 54250 while allowing color to be initialized by the user. Also, write a display method that prints in this manner:
Brand: Samsung
Model: S10
Color: Black
Price: 53300.0
Write a method updatePrice that updates the price of the mobile phone. The method accepts a price. If the value of the price is negative the price must be deducted with the value. But if positive price must be added with the value. It will not allow update of price if will result to a negative value for price.
For example:
Test | Result |
---|---|
MobilePhone phone = new MobilePhone("Samsung", "S10", "Black", 53300.00); phone.display(); |
Brand: Samsung Model: S10 Color: Black Price: 53300.0 |
MobilePhone phone = new MobilePhone(); phone.updatePrice(1200); phone.display(); |
Brand: null Model: null Color: null Price: 1200.0 |
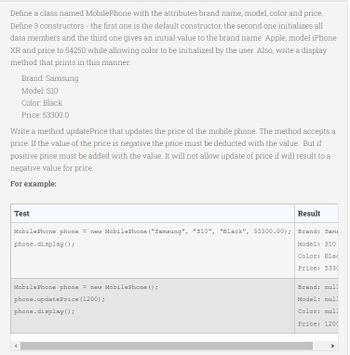

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- Internally your program should have two Java classes: ValidatorNumeric (superclass) ValidatorString (subclass) The subclass ValidatorString extends ValidatorNumeric class, forming a hierarchy of two classes. Add a separate main class with the main() method to test all public methods in both ValidatorNumeric and ValidatorString classes. The main() method should create the necessary objects and run the user interaction as shown above. The ValidatorNumeric class is responsible for capturing user prompt and a range of valid numeric inputs. Its methods display the prompt and accept input from the user. It should provide the following public interface: // Default constructor ValidatorNumeric() // Specific constructor taking params as follows: ValidatorNumeric( String prompt, int min, int max ) // Another specific constructor taking params as follows: ValidatorNumeric( String prompt, double min, double max ) // Method that shows user prompt and gets an int from the user: public…arrow_forwardConstruct a Time class containing integer data members seconds, minutes, and hours. Have the class contain two constructors: The first should be a default constructor having the prototype Time(int, int, int), which uses default values of 0 for each data member. The second constructor should accept a long integer representing a total number of seconds and disassemble the long integer into hours, minutes, and seconds. The final member method should display the class data members.arrow_forwardDefine a class named Employee with the following attributes: name, employee_id, department, and position. The Employee class should also have the following methods: __init__(self, name, employee_id, department, position): a constructor method that initializes an Employee object with name, employee_id, department, and position. get_details(self): a method that prints the details of the employee in the following format: "Name: John, ID: 001, Department: IT, Position: Software Engineer". Create a class named EmployeeManagement which will manage a list of Employee objects. This class should have the following methods: add_employee(self, employee): a method that takes an Employee object and adds it to the list of employees. remove_employee(self, employee_id): a method that takes an employee_id and removes the corresponding Employee object from the list. display_all_employees(self): a method that iterates over the list of employees and calls the get_details() method on each Employee…arrow_forward
- 1. Write a class named Coin. The Coin class should have the following field: • A String named sideUp. The sideUp field will hold either "heads" or "tails" indicating the side of the coin that is facing up. The Coin class should have the following methods: • A no-arg constructor that randomly determines the side of the coin that is facing up ("heads" or "tails") and initializes the sideUp field accordingly. • A void method named toss that simulates the tossing of the coin. When the toss method is called, it randomly determines the side of the coin that is facing up ("heads" or "tails") and sets the sideUp field accordingly. • A method named getSide Up that returns the value of the side Up field. Write a program that demonstrates the Coin class. The program should create an instance of the class and display the side that is initially facing up. Then, use a loop to toss the coin 20 times. Each time the coin is tossed, display the side that is facing up. The program should keep count of…arrow_forwardJava Program This assignment requires one project with two classes. Class Employee Class Employee- I will attach the code for this: //Import the required packages. import java.text.DecimalFormat; import java.text.NumberFormat; //Define the employee class. class Employee { //Define the data members. private String id, lastName, firstName; private int salary; //Create the constructor. public Employee(String id, String lastName, String firstName, int salary) { this.id = id; this.lastName = lastName; this.firstName = firstName; this.salary = salary; } //Define the getter methods. public String getId() { return id; } public String getLastName() { return lastName; } public String getFirstName() { return firstName; } public int getSalary() { return salary; } //Define the method to return the employee details. @Override public String toString() { //Use number format and decimal format //to…arrow_forwardjavacoding please thank you!arrow_forward
- 1. Design a new Triangle class that extends the abstract GeometricObject class. Write a test program that prompts the user to enter three sides of the triangle, a color, and a Boolean value to indicate whether the triangle is filled. The program should create a Triangle object with these sides and set the color and filled properties using the input. The program should display the area, perimeter, color, and true or false to indicate whether it is filled or not.arrow_forwardJava Program This assignment requires one project with two classes. Class Employee Class Employee- I will attach the code for this: //Import the required packages. import java.text.DecimalFormat; import java.text.NumberFormat; //Define the employee class. class Employee { //Define the data members. private String id, lastName, firstName; private int salary; //Create the constructor. public Employee(String id, String lastName, String firstName, int salary) { this.id = id; this.lastName = lastName; this.firstName = firstName; this.salary = salary; } //Define the getter methods. public String getId() { return id; } public String getLastName() { return lastName; } public String getFirstName() { return firstName; } public int getSalary() { return salary; } //Define the method to return the employee details. @Override public String toString() { //Use number format and decimal format //to…arrow_forwardPlease type the code for the following problems in Pythonarrow_forward
- Create an Account class that a bank might use to represent customers’ bank accounts. Include a data member to represent the account balance. Provide a constructor that receives an initial balance and uses it to initialize the data member. The constructor should validate the initial balance to ensure that it is greater than or equal to 0. If not, set the balance to 0. Provide three member functions:- credit($amount) should add amount to the current balance- debit($amount) should ensure that amount does not exceed the current balance and then reduce the current balance by amount. The balance should not change if amount exceeds the balance.- getBalance() should return the current balanceNote: Create a set of test data and then write a program to test the class.arrow_forwardDesign a class named octopus that holds attributes for an octopus named weight and color. Include methods to set and get each of these attributes. Create the class diagram and write the class pseudocode that defines the class. Create a small program that declares two octopus objects and set the octopus's name weight and color attributes the program must also output each octopus's name weight and color after they are set. Please write out the pseudocode. I have a hard time with this.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
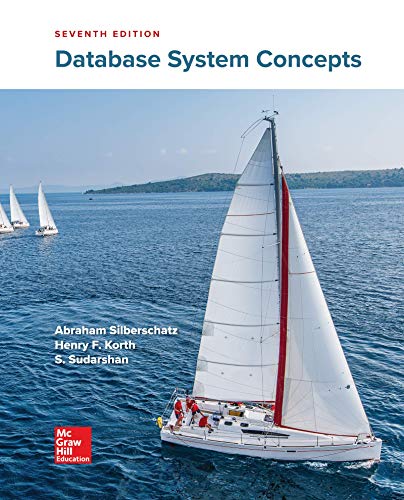
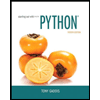
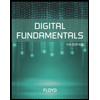
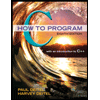
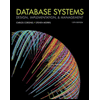
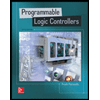