Design a function that accepts a positive integer >= 1 and returns the sum of all the integers from 1 up to the number passed as an argument. For example, if 10 is passed as an argument, the function will return 55. Use recursion to calculate the sum. Write a second function which asks the user for the integer and displays the result of calling the function.
Problem Statement for Recursive Sum of Numbers Program
Here is a simple recursive problem: Design a function that accepts a positive integer >= 1 and returns the sum of all the integers from 1 up to the number passed as an argument. For example, if 10 is passed as an argument, the function will return 55. Use recursion to calculate the sum. Write a second function which asks the user for the integer and displays the result of calling the function.
Part 1. Understand the Problem
To design a recursive function, you need to determine at least one base case (the base case returns a solution) and a general case (the general case calls the function again but passes a smaller version of the data as a parameter). To make this problem recursive think about it like this:
If the integer is 1, the function will return 1.
If the integer is 2, the function will return 1 + 2 = 3.
If the integer is 3, the function will return 1 + 2 + 3 = 6.
. . .
For this problem, we will be sending in the "last" integer as the parameter.The function will have to call itself recursively, passing a parameter one less than the number itself, and continue doing this until the parameter is 1. That is when the recursion can stop because the function will return a 1 without making a recursive call. The sum will”build up" from the bottom. The
If n = 1, sum(n) = 1
If n > 1, sum(n) = n + sum(n-1)
To see how this works, assume n = 6. After the first call to sum(6), the function returns 6 + sum(5). However sum(5) is another function call, so this call must be made and completed before 6 + sum(5) can be calculated. When sum(5) is called, it will return 5 + sum(4) which cannot be evaluated until after sum(4) is called and returns. The call to sum(4) returns 4 + sum(3) which cannot be evaluated until after sum(3) is called and returns. When sum(3) is called it returns 3 + sum(2) and when sum(2) is called it returns 2 + sum(1). Finally, when sum(1)is called it can return a 1 without making another recursive call. The 1 gets returned to sum(2) and now sum(2) can be evaluated and returns 2 + 1 = 3 to sum(3). The function sum(3) can now calculate 3 + 3 and return 6 to sum(4). The function sum(4) can now calculate 4 + 6 and return 10 to sum(5). The function sum(5) can now calculate 5 + 10 andreturn 15 to sum(6) which can now calculate 6 + 15 = 21 which is the sum of 1 + 2 + 3 + 4 + 5 + 6.
(PYTHON)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

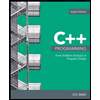
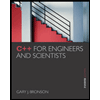
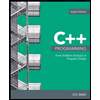
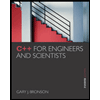