Design a program that asks the user to enter the weight of the package and displays the shipping charges.
Design a program that asks the user to enter the weight of the package and displays the shipping charges.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter7: User-defined Simple Data Types, Namespaces, And The String Type
Section: Chapter Questions
Problem 7PE
Related questions
Question
(PYTHON)
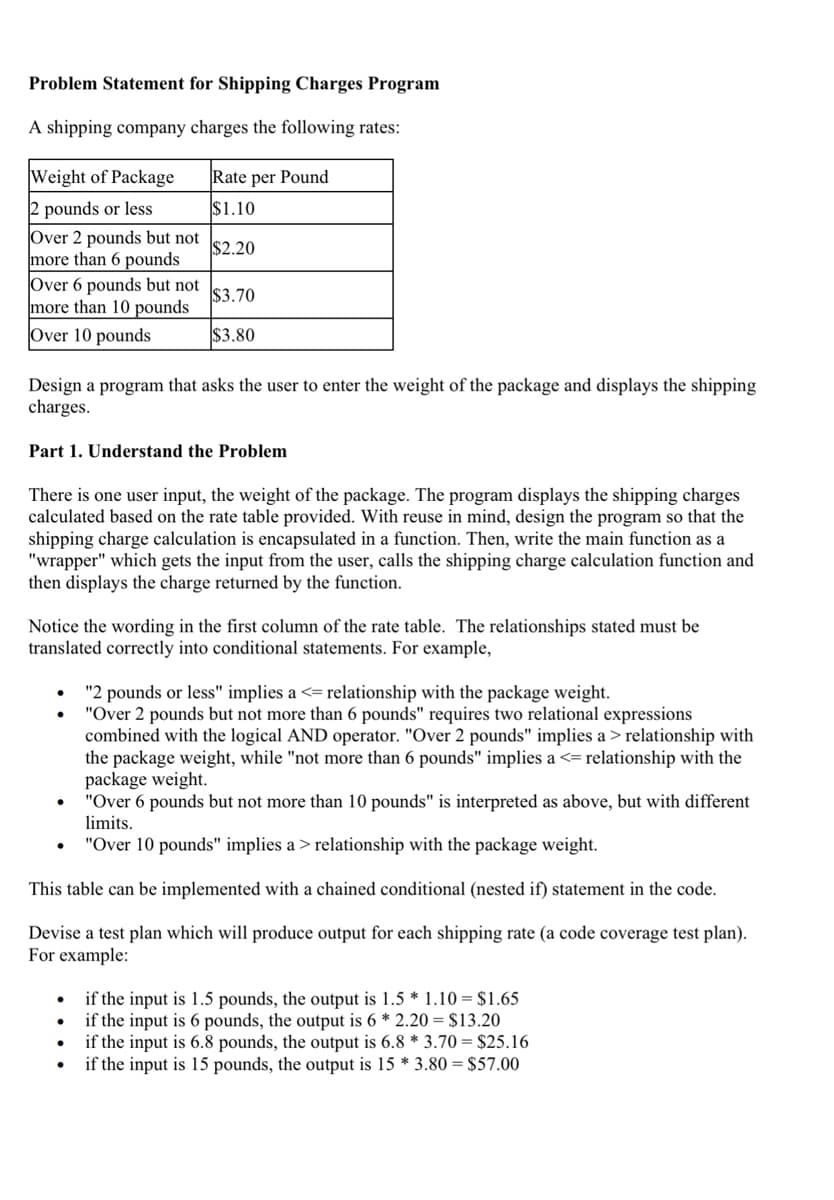
Transcribed Image Text:Problem Statement for Shipping Charges Program
A shipping company charges the following rates:
Weight of Package
Rate per Pound
2 pounds or less
Over 2 pounds but not
more than 6 pounds
Over 6 pounds but not
more than 10 pounds
$1.10
$2.20
$3.70
Over 10 pounds
$3.80
Design a program that asks the user to enter the weight of the package and displays the shipping
charges.
Part 1. Understand the Problem
There is one user input, the weight of the package. The program displays the shipping charges
calculated based on the rate table provided. With reuse in mind, design the program so that the
shipping charge calculation is encapsulated in a function. Then, write the main function as a
"wrapper" which gets the input from the user, calls the shipping charge calculation function and
then displays the charge returned by the function.
Notice the wording in the first column of the rate table. The relationships stated must be
translated correctly into conditional statements. For example,
"2 pounds or less" implies a <= relationship with the package weight.
"Over 2 pounds but not more than 6 pounds" requires two relational expressions
combined with the logical AND operator. "Over 2 pounds" implies a > relationship with
the package weight, while "not more than 6 pounds" implies a <= relationship with the
package weight.
"Over 6 pounds but not more than 10 pounds" is interpreted as above, but with different
limits.
"Over 10 pounds" implies a > relationship with the package weight.
This table can be implemented with a chained conditional (nested if) statement in the code.
Devise a test plan which will produce output for each shipping rate (a code coverage test plan).
For example:
if the input is 1.5 pounds, the output is 1.5 * 1.10 = $1.65
if the input is 6 pounds, the output is 6 * 2.20 = $13.20
if the input is 6.8 pounds, the output is 6.8 * 3.70 = $25.16
if the input is 15 pounds, the output is 15 * 3.80 = $57.00
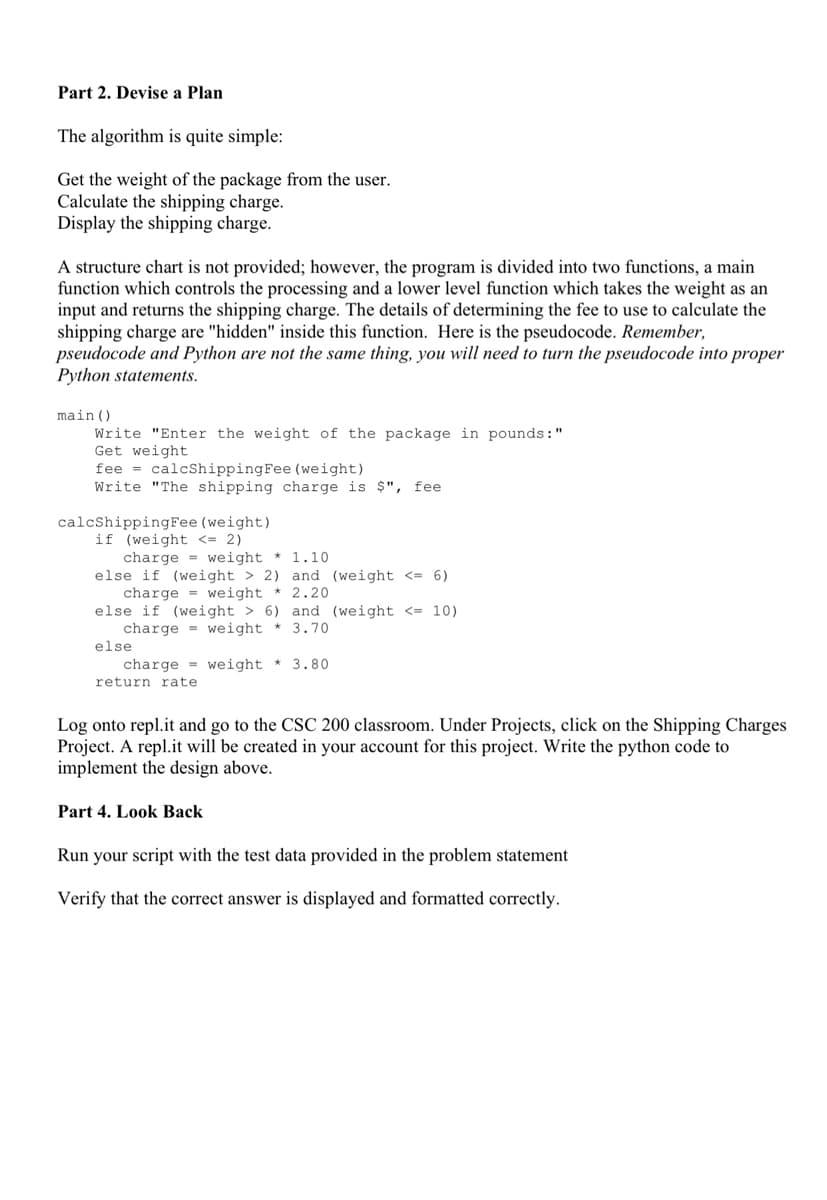
Transcribed Image Text:Part 2. Devise a Plan
The algorithm is quite simple:
Get the weight of the package from the user.
Calculate the shipping charge.
Display the shipping charge.
A structure chart is not provided; however, the program is divided into two functions, a main
function which controls the processing and a lower level function which takes the weight as an
input and returns the shipping charge. The details of determining the fee to use to calculate the
shipping charge are "hidden" inside this function. Here is the pseudocode. Remember,
pseudocode and Python are not the same thing, you will need to turn the pseudocode into proper
Python statements.
main ()
Write "Enter the weight of the package in pounds:"
Get weight
fee = calcShippingFee (weight)
Write "The shipping charge is $", fee
calcShippingFee (weight)
if (weight <= 2)
charge = weight * 1.10
else if (weight > 2) and (weight <= 6)
charge = weight * 2.20
else if (weight > 6) and (weight <= 10)
charge
weight * 3.70
else
charge = weight * 3.80
return rate
Log onto repl.it and go to the CSC 200 classroom. Under Projects, click on the Shipping Charges
Project. A repl.it will be created in your account for this project. Write the python code to
implement the design above.
Part 4. Look Back
Run your script with the test data provided in the problem statement
Verify that the correct answer is displayed and formatted correctly.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
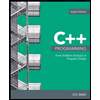
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
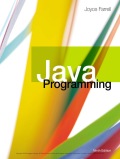
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
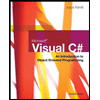
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
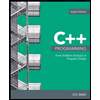
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
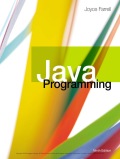
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
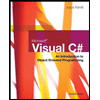
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,