Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts Develop the following functionality: Develop a linked list node struct/class You can use it as a subclass like in the book (Class contained inside a class) You can use it as its own separate class Your choice Maintain a private pointer to a node class pointer (head) Constructor Initialize head pointer to null Destructor Make sure to properly delete every node in your linked list push_front(value) Insert the value at the front of the linked list pop_front() Remove the node at the front of the linked list If empty, this is a no operation operator << Display the contents of the linked list just like you would print a character string operator [] Treat like an array Return the value stored in that element of the linked list If element doesn’t exist, return std::numeric_limits::min() empty() Returns a boolean that determines if the linked list is empty or not size() Returns how many elements are currently in the linked list You may not use a variable to maintain the size You must calculate it manually using pointers insert(positionIndex, value) Think vector indexing positionIndex of 0 means insert at the beginning of the linked list positionIndex >= linked list size gets inserted at the back of the linked list erase(positionIndex) Think vector indexing positionIndex of 0 means insert at the beginning of the linked list positionIndex >= linked list size is a no operation find(value) Searches the linked list for the given value Think vector indexing If found, returns the index value it is at If not found, returns -1 Extensively test your linked list class to make sure all above functionality works in all possible cases
Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts Develop the following functionality: Develop a linked list node struct/class You can use it as a subclass like in the book (Class contained inside a class) You can use it as its own separate class Your choice Maintain a private pointer to a node class pointer (head) Constructor Initialize head pointer to null Destructor Make sure to properly delete every node in your linked list push_front(value) Insert the value at the front of the linked list pop_front() Remove the node at the front of the linked list If empty, this is a no operation operator << Display the contents of the linked list just like you would print a character string operator [] Treat like an array Return the value stored in that element of the linked list If element doesn’t exist, return std::numeric_limits::min() empty() Returns a boolean that determines if the linked list is empty or not size() Returns how many elements are currently in the linked list You may not use a variable to maintain the size You must calculate it manually using pointers insert(positionIndex, value) Think vector indexing positionIndex of 0 means insert at the beginning of the linked list positionIndex >= linked list size gets inserted at the back of the linked list erase(positionIndex) Think vector indexing positionIndex of 0 means insert at the beginning of the linked list positionIndex >= linked list size is a no operation find(value) Searches the linked list for the given value Think vector indexing If found, returns the index value it is at If not found, returns -1 Extensively test your linked list class to make sure all above functionality works in all possible cases
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Read this: Complete the code in Visual Studio using C++ Programming Language
- Give me the answer in Visual Studio so I can copy***
- Separate the files such as .cpp, .h, or .main if any!
Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts
Develop the following functionality:
- Develop a linked list node struct/class
- You can use it as a subclass like in the book (Class contained inside a class)
- You can use it as its own separate class
- Your choice
- Maintain a private pointer to a node class pointer (head)
- Constructor
- Initialize head pointer to null
- Destructor
- Make sure to properly delete every node in your linked list
- push_front(value)
- Insert the value at the front of the linked list
- pop_front()
- Remove the node at the front of the linked list
- If empty, this is a no operation
- operator <<
- Display the contents of the linked list just like you would print a character string
- operator []
- Treat like an array
- Return the value stored in that element of the linked list
- If element doesn’t exist, return std::numeric_limits<short>::min()
- empty()
- Returns a boolean that determines if the linked list is empty or not
- size()
- Returns how many elements are currently in the linked list
- You may not use a variable to maintain the size
- You must calculate it manually using pointers
- insert(positionIndex, value)
- Think
vector indexing - positionIndex of 0 means insert at the beginning of the linked list
- positionIndex >= linked list size gets inserted at the back of the linked list
- erase(positionIndex)
- Think vector indexing
- positionIndex of 0 means insert at the beginning of the linked list
- positionIndex >= linked list size is a no operation
- find(value)
- Searches the linked list for the given value
- Think vector indexing
- If found, returns the index value it is at
- If not found, returns -1
Extensively test your linked list class to make sure all above functionality works in all possible cases
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
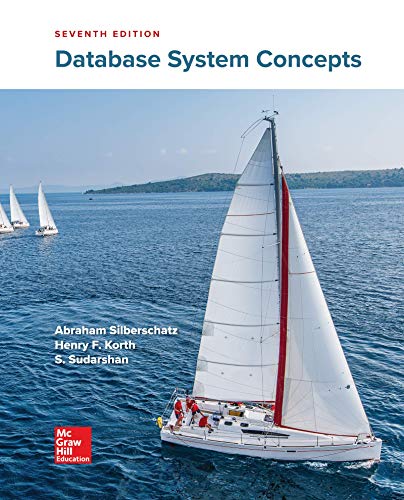
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
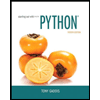
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
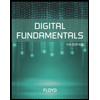
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
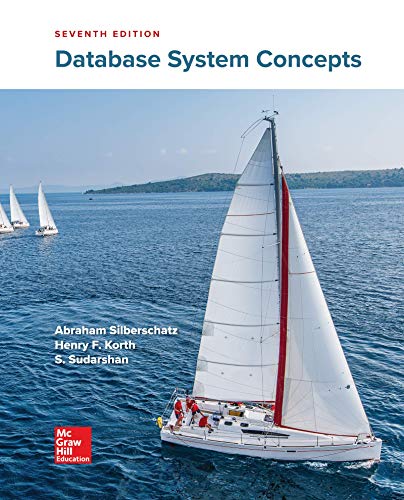
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
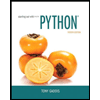
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
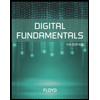
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
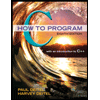
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
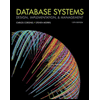
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
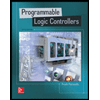
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education