Using java, write an easier version of a linked list with only a couple of the normal linked list functions and the ability to generate and utilize a list of ints. The data type of the connection to the following node can be just Node, and the data element can be just an int. You will need a reference like (Java) or either a reference to the first node, as well as one to the last node. (Answer the following questions) 1) Create a method or function that accepts an integer, constructs a node with that integer as its data value, and then includes the node to the end of the list. If the new node is the first one, this function will also need to update the reference to the first node. This function will need to update the reference to the final node. Consider how to insert the new node following the previous last node, and keep in mind that the next reference for the list's last node should be null. 2) Create a different method or function that iteratively explores the list, printing the int data values as it goes. It should start at the first node, follow the links to the final node, and so forth.
Using java, write an easier version of a linked list with only a couple of the normal linked list functions and the ability to generate and utilize a list of ints. The data type of the connection to the following node can be just Node, and the data element can be just an int. You will need a reference like (Java) or either a reference to the first node, as well as one to the last node.
(Answer the following questions)
1) Create a method or function that accepts an integer, constructs a node with that integer as its data value, and then includes the node to the end of the list. If the new node is the first one, this function will also need to update the reference to the first node. This function will need to update the reference to the final node. Consider how to insert the new node following the previous last node, and keep in mind that the next reference for the list's last node should be null.
2) Create a different method or function that iteratively explores the list, printing the int data values as it goes. It should start at the first node, follow the links to the final node, and so forth.

Step by step
Solved in 4 steps with 1 images

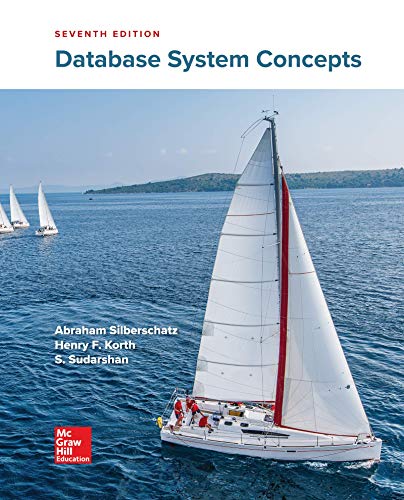
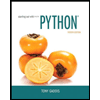
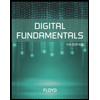
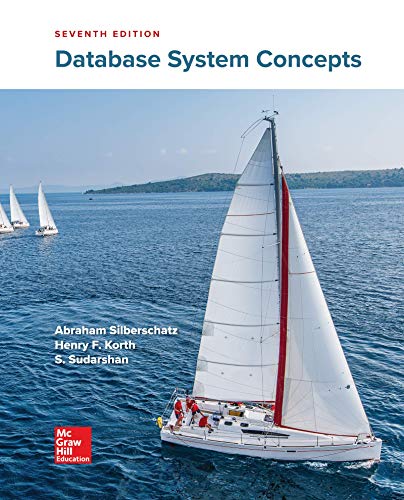
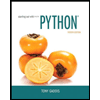
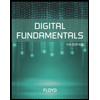
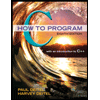
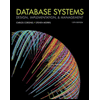
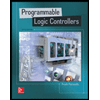