Double Bubble For this exercise you need to create a Bubble class and construct two instances of the Bubble object. You will then take the two Bubble objects and combine them to create a new, larger combined Bubble object. This will be done using functions that take in these Bubble objects as parameters. The Bubble class contains one data member, radius_, and the corresponding accessor and mutator methods for radius_, GetRadius and SetRadius. Create a member function called CalculateVolume that computes for the volume of a bubble (sphere). Use the value 3.1415 for PI. Your main function has some skeleton code that asks the user for the radius of two bubbles. You will use this to create the two Bubble objects. You will create a CombineBubbles function that receives two references (two Bubble objects) and returns a Bubble object. Combining bubbles simply means creating a new Bubble object whose radius is the sum of the two Bubble objects' radii. Take note that the CombineBubbles function is not part of the Bubble` class. Place the Bubble class and the CombineBubbles function declaration in bubble.h. Implement CombineBubbles in bubble.cc. The main function already contains some code, but you need to complete the requirements that is described inside the file. Please see the sample output below to guide the design of your program. Sample Output Please enter the radius of the first bubble: 4.5 Please enter the radius of the second bubble: 2.3 The bubbles have now combined and created a bubble with the volume of: 1317.05 main.cc file #include #include #include "bubble.h" int main() { // 1. Create two bubble objects called `bubble1` and `bubble2` double radius1 = 0.0; double radius2 = 0.0; std::cout << "Please enter the radius of the first bubble: "; std::cin >> radius1; // 2. Set the radius of bubble1 to radius1 std::cout << "Please enter the radius of the second bubble: "; std::cin >> radius2; // 3. Set the radius of bubble2 to radius2 // 4. Create a new bubble object called `combined` // 5. Call the CombineBubbles function to combine the bubble1 // and bubble2 objects, then store the result in the combined variable. double volume = 0.0; // 6. Get the volume of `combined` and set the volume variable above. std::cout << "The bubbles have now combined and created a bubble with the volume " "of: " << std::fixed << std::setprecision(2) << volume << std::endl; return 0; } bubble.cc file #include "bubble.h" // ========================= YOUR CODE HERE ========================= // This implementation file (bubble.cc) is where you should implement // the member functions declared in the header (bubble.h), only // if you didn't implement them inline within bubble.h: // 1. GetRadius // 2. SetRadius // 3. CalculateVolume // // Remember to specify the name of the class with :: in this format: // MyClassName::MyFunction() { // ... // } // to tell the compiler that each function belongs to the Bubble class. // =================================================================== // ========================= YOUR CODE HERE ========================= // Implement the CombineBubbles function you declared in bubble.h // // Since CombineBubbles is NOT in the Bubble class, you should not // specify the name of the class with the :: format. // =================================================================== bubble.h file // ======================= YOUR CODE HERE ======================= // Write the Bubble class here, containing one member variable, // the radius_. Note that radius_ can be a decimal value. // // Then, define the following member functions: // 1. The accessor (getter) function for radius_. // 2. The mutator (setter) function for radius_. // 3. The CalculateVolume member function. // // Note: mark functions that do not modify the member variables // as const, by writing `const` after the parameter list. // =============================================================== class Bubble { }; // ========================= YOUR CODE HERE ========================= // Write the function declaration for the CombineBubbles function. // // 1. Use pass-by-reference to pass in the Bubble objects efficiently. // 2. Consider if the inputs passed in should be modified. If not, // please mark them const.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Double Bubble
For this exercise you need to create a Bubble class and construct two instances of the Bubble object. You will then take the two Bubble objects and combine them to create a new, larger combined Bubble object. This will be done using functions that take in these Bubble objects as parameters.
The Bubble class contains one data member, radius_, and the corresponding accessor and mutator methods for radius_, GetRadius and SetRadius. Create a member function called CalculateVolume that computes for the volume of a bubble (sphere). Use the value 3.1415 for PI.
Your main function has some skeleton code that asks the user for the radius of two bubbles. You will use this to create the two Bubble objects. You will create a CombineBubbles function that receives two references (two Bubble objects) and returns a Bubble object. Combining bubbles simply means creating a new Bubble object whose radius is the sum of the two Bubble objects' radii. Take note that the CombineBubbles function is not part of the Bubble` class.
Place the Bubble class and the CombineBubbles function declaration in bubble.h. Implement CombineBubbles in bubble.cc. The main function already contains some code, but you need to complete the requirements that is described inside the file.
Please see the sample output below to guide the design of your program.
Sample Output
#include <iomanip>
#include <iostream>
#include "bubble.h"
int main() {
// 1. Create two bubble objects called `bubble1` and `bubble2`
double radius1 = 0.0;
double radius2 = 0.0;
std::cout << "Please enter the radius of the first bubble: ";
std::cin >> radius1;
// 2. Set the radius of bubble1 to radius1
std::cout << "Please enter the radius of the second bubble: ";
std::cin >> radius2;
// 3. Set the radius of bubble2 to radius2
// 4. Create a new bubble object called `combined`
// 5. Call the CombineBubbles function to combine the bubble1
// and bubble2 objects, then store the result in the combined variable.
double volume = 0.0;
// 6. Get the volume of `combined` and set the volume variable above.
std::cout
<< "The bubbles have now combined and created a bubble with the volume "
"of: "
<< std::fixed << std::setprecision(2) << volume << std::endl;
return 0;
}
bubble.cc file
#include "bubble.h"
// ========================= YOUR CODE HERE =========================
// This implementation file (bubble.cc) is where you should implement
// the member functions declared in the header (bubble.h), only
// if you didn't implement them inline within bubble.h:
// 1. GetRadius
// 2. SetRadius
// 3. CalculateVolume
//
// Remember to specify the name of the class with :: in this format:
// <return type> MyClassName::MyFunction() {
// ...
// }
// to tell the compiler that each function belongs to the Bubble class.
// ===================================================================
// ========================= YOUR CODE HERE =========================
// Implement the CombineBubbles function you declared in bubble.h
//
// Since CombineBubbles is NOT in the Bubble class, you should not
// specify the name of the class with the :: format.
// ===================================================================
bubble.h file
// ======================= YOUR CODE HERE =======================
// Write the Bubble class here, containing one member variable,
// the radius_. Note that radius_ can be a decimal value.
//
// Then, define the following member functions:
// 1. The accessor (getter) function for radius_.
// 2. The mutator (setter) function for radius_.
// 3. The CalculateVolume member function.
//
// Note: mark functions that do not modify the member variables
// as const, by writing `const` after the parameter list.
// ===============================================================
class Bubble {
};
// ========================= YOUR CODE HERE =========================
// Write the function declaration for the CombineBubbles function.
//
// 1. Use pass-by-reference to pass in the Bubble objects efficiently.
// 2. Consider if the inputs passed in should be modified. If not,
// please mark them const.
// ===================================================================

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 3 images

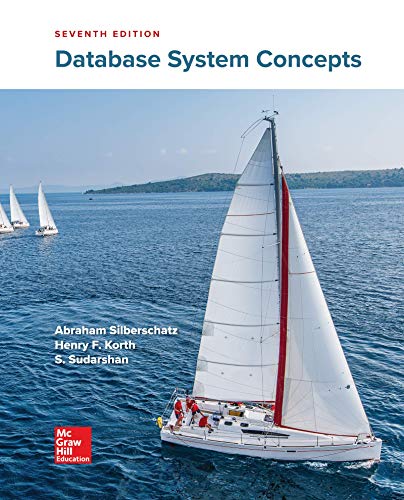
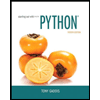
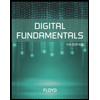
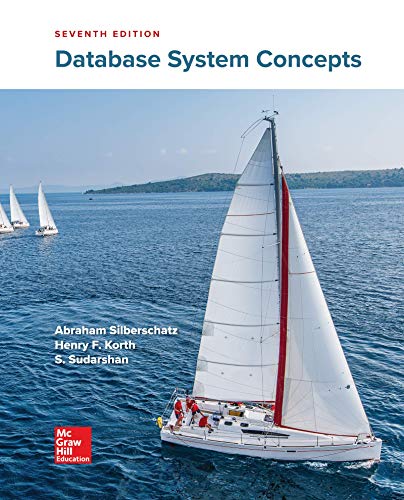
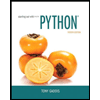
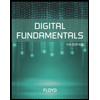
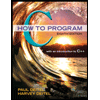
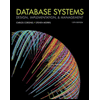
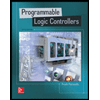