Extra 6-1 Develop the Temperature Converter In this exercise, you’ll use radio buttons to determine whether the conversion is from Fahrenheit to Celsius or vice versa. You’ll also modify the DOM so the labels change when a radio button is clicked, and the page displays an error message when the user enters invalid data. 1. Open the application in this folder: exercises_extrach06convert_temps 2. Note that the JavaScript file has some starting JavaScript code, including the $() function, three helper functions, three event handler functions, and a DOMContentLoaded event handler that attaches the three event handlers. 3. Review how the toCelsius() and toFarhenheit() event handler functions call the toggleDisplay() helper function and pass it strings to display. Also note that the toggleDisplay() helper function and the convertTemp() event handler function are incomplete. 4. Code the toggleDisplay() function so it changes the text in the labels for the text boxes to the values in the parameters that it receives. It should also clear the disabled text box where the computed temperature is displayed. 5. Code the convertTemp() function without any data validation. It should use the helper functions to calculate the temperature based on which radio button is checked. The value returned by the helper functions should be rounded to zero decimal places. 6. Add data validation to the convertTemp() function. If the entry is note a valid number, a validation message should be displayed in the element with the id of “message”. 7. Add any finishing touches to the application whenever that’s appropriate. This can be things like moving the focus to the first text box and selecting the text, clearing a previous error message, or clearing a previously computed temperature value.
Extra 6-1 Develop the Temperature Converter
In this exercise, you’ll use radio buttons to determine whether the conversion is from Fahrenheit to Celsius or vice versa. You’ll also modify the DOM so the labels change when a radio button is clicked, and the page displays an error message when the user enters invalid data.
1. Open the application in this folder:
exercises_extrach06convert_temps
2. Note that the JavaScript file has some starting JavaScript code, including the $() function, three helper functions, three event handler functions, and a DOMContentLoaded event handler that attaches the three event handlers.
3. Review how the toCelsius() and toFarhenheit() event handler functions call the toggleDisplay() helper function and pass it strings to display. Also note that the toggleDisplay() helper function and the convertTemp() event handler function are incomplete.
4. Code the toggleDisplay() function so it changes the text in the labels for the text boxes to the values in the parameters that it receives. It should also clear the disabled text box where the computed temperature is displayed.
5. Code the convertTemp() function without any data validation. It should use the helper functions to calculate the temperature based on which radio button is checked. The value returned by the helper functions should be rounded to zero decimal places.
6. Add data validation to the convertTemp() function. If the entry is note a valid number, a validation message should be displayed in the element with the id of “message”.
7. Add any finishing touches to the application whenever that’s appropriate. This can be things like moving the focus to the first text box and selecting the text, clearing a previous error message, or clearing a previously computed temperature value.
![<> index.html X #convert_temp.css. JS convert_temp.js.
exercises_extra > ch06 > convert_temps> <> index.html > ...
1 k!DOCTYPE html>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
25700
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
34
36
<title>Convert Temperatures</title>
<link rel="stylesheet" href="convert_temp.css">
</head>
<body>
1
2
3
4
<main>
5
6
</body>
</html>
<h1>Convert temperatures</h1>
<div>
}
9 vh1{
</div>
<div>
}
<> index.html
<input type="radio" name="conversion_type" id="to_celsius" checked>Fahrenheit to Celsius
</div>
<div>
<input type="radio" name="conversion_type" id="to_fahrenheit">Celsius to Fahrenheit
<> index.html
# convert_temp.css
exercises_extra > ch06 > convert_temps > # convert_temp.css > #convert
1 ✓ body {
</div>
<div>
<label id="degree_label_1">Enter F degrees: </label>
<input type="text" id="degrees_entered">
</main>
<script src="convert_temp.js"></script>
</div>
<div>
<label id="degree_label_2">Degrees Celsius:</label>
<input type="text" id="degrees_computed" disabled>
};
10
11 }
12 v div {
13
14 }
15 ✓ label {
16
17
18
19 }
20 ✓ input {
21
22
23 }
24 ✓ span {
25
});
<label></label>
</div>
<input type="button" id="convert" value="Convert" />
26 }
27 ✓ #convert
28
29
30
31
32
33
font-family: Arial, Helvetica, sans-serif;
background-color: I white;
margin: 0 auto;
width: 500px;
border: 3px solid blue;
padding: 0 2em 1em;
color: blue;
margin-bottom: 1em;
/******
display: inline-block;
width: 11em;
text-align: right;
margin-left: 1em;
margin-right: 0.5em;
color: red;
H
/*div that's the 3rd child of its parent element (main) */
div:nth-child(3) {
margin-bottom: 1.5em;
# convert_temp.css
JS convert_temp.js.
exercises_extra > ch06 > convert_temps > JS convert_temp.js > [e] toggleDisplay
"use strict";
const $ = selector => document.querySelector (selector);
width: 10em;
helper functions
7 const calculateCelsius
temp => (temp-32) * 5/9;
8 const calculateFahrenheit = temp => temp * 9/5 + 32;
9
10
11
12
13
14
15
16
17
18
19
20
21 const toCelsius
() => toggleDisplay("Enter F degrees:", "Degrees Celsius:");
22 const toFahrenheit = () => toggleDisplay("Enter C degrees:", "Degrees Fahrenheit:");
23
24 document.addEventListener("DOMContent Loaded", () => {
// add event handlers
25
26
27
28
29
30
31
32
const toggleDisplay = (label1Text, label2Text) =>
周
const convertTemp = () => {
JS convert_temp.js
=
event handler functions
=
// move focus
$("#convert").addEventListener("click", convertTemp);
$("#to_celsius").addEventListener("click", toCelsius);
$("#to_fahrenheit").addEventListener("click", toFahrenheit);
$("#degrees_entered").focus();](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5cb9f425-fe9e-4cb9-a79d-57c845d48575%2Fb1fc6e34-c0d5-4fb5-a3e0-4098a40f57c4%2F2kzlsd_processed.jpeg&w=3840&q=75)

Algorithm:
- Define the HTML page as index.html and CSS file as style.css and javascript file as convert_temp.js.
- Define a strict mode for JavaScript. Define a function that takes an id and returns the element with that id from the DOM.
- Define a function to clear the input and output text boxes.
- Define a function to update the labels to prompt the user for temperature input in either Fahrenheit or Celsius.
- Define a function to convert the temperature from Fahrenheit to Celsius or Celsius to Fahrenheit, depending on the radio button selected by the user.
- Check if the input temperature value is a valid number.
- If the value is valid, convert the temperature based on the radio button selected and output the result in the output text box.
- When the window loads, assign event listeners to the convert button and the radio buttons for Fahrenheit and Celsius options.
- Set the focus on the input text box to make it easier for the user to enter temperature values.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

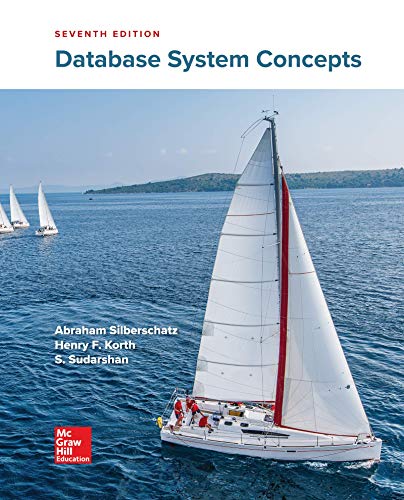
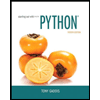
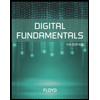
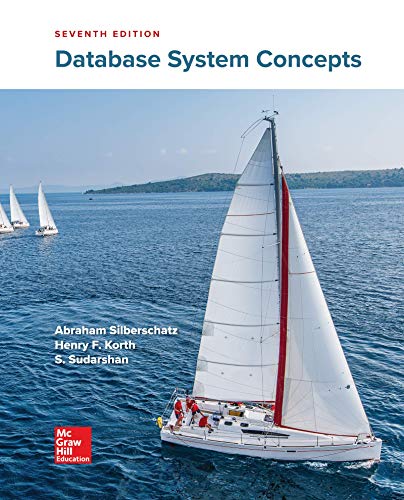
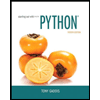
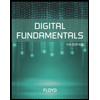
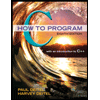
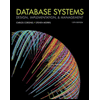
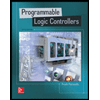