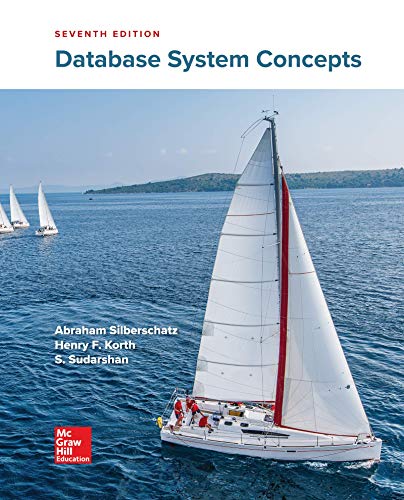
Python
def _insertionsort(self, order):
### Problem 1:
# Implements the insertion sort
#######################################################################
# Remove the pass and write your code
#######################################################################
pass
#######################################################################
# End code
#######################################################################
def _mergesort(self, order):
### Problem 2:
# Implements the merge sort algorithm to sort atos
#######################################################################
# Remove the pass and write your code
#######################################################################
pass
#######################################################################
# End code
#######################################################################
def _countsort(self, order):
### Problem 3:
# Implements the count sort algorithm to sort atos
#######################################################################
# Remove the pass and write your code
#######################################################################
pass
#######################################################################
# End code
#######################################################################
def _quicksort(self, order):
### Problem 4:
# Implements the quick sort algorithm to sort atos
#######################################################################
# Remove the pass and write your code
#######################################################################
pass
#######################################################################
# End code
#######################################################################
def _radixsort(self, order):
### Problem 5:
# Implements the radix sort algorithm to sort atos
#######################################################################
# Remove the pass and write your code
#######################################################################
pass
#######################################################################
# End code
#######################################################################
def _heapsort(self, order):
### Problem 6:
# Implements the heap sort algorithm to sort atos
#######################################################################
# Remove the pass and write your code
#######################################################################
pass
#######################################################################
# End code
#######################################################################

Here is an implementation of the insertion sort algorithm in Python to sort a list of items in ascending or descending order based on the order parameter:
CODE in Python:
def _insertionsort(self, order):
# Implements the insertion sort algorithm to sort a list of items in ascending or descending order
n = len(self.items)
for i in range(1, n):
key = self.items[i]
j = i - 1
if order == 'asc':
while j >= 0 and key < self.items[j]:
self.items[j + 1] = self.items[j]
j -= 1
self.items[j + 1] = key
elif order == 'desc':
while j >= 0 and key > self.items[j]:
self.items[j + 1] = self.items[j]
j -= 1
self.items[j + 1] = key
Here's an implementation of the merge sort algorithm in Python:
CODE in Python:
def _mergesort(self, order):
if len(self.atos) > 1:
mid = len(self.atos) // 2
left_half = self.atos[:mid]
right_half = self.atos[mid:]
self.atos = []
left_half = self._mergesort(left_half)
right_half = self._mergesort(right_half)
while len(left_half) > 0 and len(right_half) > 0:
if order(left_half[0], right_half[0]):
self.atos.append(left_half[0])
left_half = left_half[1:]
else:
self.atos.append(right_half[0])
right_half = right_half[1:]
self.atos += left_half
self.atos += right_half
return self.atos
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- JAVA The following code for InsertionSort is given to us by the textbook. Trace the code stepby step using the array[55, 22, 77, 99, 66, 33, 11]on a piece of paper or using a Word document. If the code has errors, correct it and make itwork.public static void insertionSort(double[] list) {for (int i = 1; i < list.length; i++) {/** insert list[i] into a sorted sublist list[0..i-1] so thatlist[0..i] is sorted. */double currentElement = list[i];int k;for (k = i - 1; k >= 0 && list[k] > currentElement; k--) {list[k + 1] = list[k];}// Insert the current element into list[k+1]list[k + 1] = currentElement;}}arrow_forwardIn Java, Apply replacement selection sort to the following list assuming array size M = 3; 27 47 35 7 67 21 32 18 24 20 12 8 Show the content of each sorted file.arrow_forwardI have implemented a stack using arrays. The array is 5 elements long and is called examstack. The contents are: examstack[0] = 36 examstack[1] = 49 examstack[2] = 7 examstack[3] = 67 examstack[4] = 9 If I did a Pop what would be returned? 9 or 36 09 07 O 67 O 9 or 67 36 or 49 O 36 49arrow_forward
- IN JAVA Write recursive code and iterative code for binary search.arrow_forwardusing python in google colabarrow_forwardCreate three problem instances of size n is around 10, representing the best-case, worst-case and average-case for Radix Sort respectively. Show how the Radix Sort works on the best-case, and explain why each of them represents the best-case, worst-case and average-case respectively. psudocode for Radixsort attachedarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
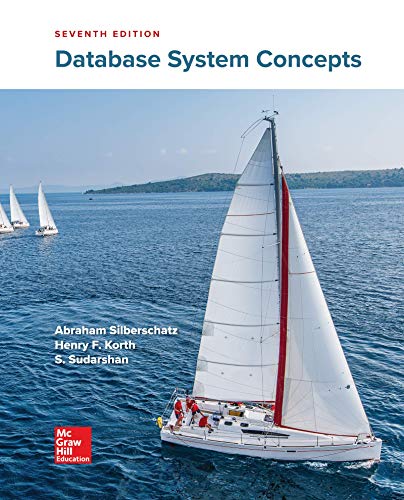
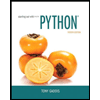
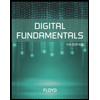
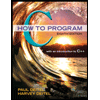
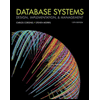
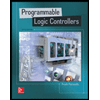