Extra 7-2 Add a stopwatch to the Clock application In this exercise, you’ll add a stopwatch feature to a digital clock application. The stopwatch will display elapsed minutes, seconds, and milliseconds. 1. Open the application in this folder: exercises_extra\ch07\clock_stopwatch\ 2. In the JavaScript file, note the $(), displayCurrentTime(), padSingleDigit(), and DOMContentLoaded event handler functions from the Clock application. In addition, note the global variables and starting code for the tickStopwatch(), startStopwatch(), stopStopwatch(), and resetStopwatch() functions. 3. In the tickStopwatch() function, add code that adds 10 milliseconds to the elapsedMilliseconds variable and then adjusts the elapsedMinutes and elapsedSeconds variables accordingly. Then, add code that displays the result in the appropriate span tags in the page. 4. In the startStopwatch() function, add code that starts the stopwatch. Be sure to cancel the default action of the link too. 5. In the stopStopwatch() and resetStopwatch() functions, add code that stops the stopwatch. Also, in the resetStopwatch() function, reset the elapsed time and the page display. Be sure to cancel the default action of the links too. 6. In the DOMContentLoaded event handler, attach the stopwatch event handlers to the appropriate links. index.html Clock Digital clock with stopwatch Clock : : Stop Watch Start Stop Reset 00: 00: 000 clock.js "use strict"; const $ = selector => document.querySelector(selector); const padSingleDigit = num => num.toString().padStart(2, "0"); const displayCurrentTime = () => { const now = new Date(); const hours = now.getHours(); let ampm = "AM"; // set default value // correct hours and AM/PM value for display if (hours > 12) { // convert from military time hours = hours - 12; ampm = "PM"; } else { // adjust 12 noon and 12 midnight switch (hours) { case 12: // noon ampm = "PM"; break; case 0: // midnight hours = 12; ampm = "AM"; } } $("#hours").textContent = hours; $("#minutes").textContent = padSingleDigit(now.getMinutes()); $("#seconds").textContent = padSingleDigit(now.getSeconds()); $("#ampm").textContent = ampm; }; //global stop watch timer variable and elapsed time object let stopwatchTimer = null; let elapsedMinutes = 0; let elapsedSeconds = 0; let elapsedMilliseconds = 0; const tickStopwatch = () => { // increment milliseconds by 10 milliseconds // if milliseconds total 1000, increment seconds by one and reset milliseconds to zero // if seconds total 60, increment minutes by one and reset seconds to zero //display new stopwatch time }; // event handler functions const startStopwatch = evt => { // prevent default action of link // do first tick of stop watch and then set interval timer to tick // stop watch every 10 milliseconds. Store timer object in stopwatchTimer // variable so next two functions can stop timer. }; const stopStopwatch = evt => { // prevent default action of link // stop timer }; const resetStopwatch = evt => { // prevent default action of link // stop timer // reset elapsed variables and clear stopwatch display }; document.addEventListener("DOMContentLoaded", () => { // set initial clock display and then set interval timer to display // new time every second. Don't store timer object because it // won't be needed - clock will just run. displayCurrentTime(); setInterval(displayCurrentTime, 1000); // set up stopwatch event handlers }); clock.css body { font-family: Arial, Helvetica, sans-serif; background-color: white; margin: 0 auto; width: 450px; border: 3px solid blue; padding: 0 2em 1em; } h1 { color: blue; } div { margin-bottom: 1em; } label { display: inline-block; width: 11em; text-align: right; } input { margin-left: 1em; margin-bottom: .5em; } fieldset { margin-bottom: 1em; } fieldset a, #seconds { margin-right: 0.5em; } library_event.js "use strict"; var evt = { attach: function(node, eventName, func) { }, detach: function(node, eventName, func) { }, preventDefault: function(e) { } };
Extra 7-2 Add a stopwatch to the Clock application
In this exercise, you’ll add a stopwatch feature to a digital clock application. The stopwatch will display elapsed minutes, seconds, and milliseconds.
1. Open the application in this folder:
exercises_extra\ch07\clock_stopwatch\
2. In the JavaScript file, note the $(), displayCurrentTime(), padSingleDigit(), and DOMContentLoaded event handler functions from the Clock application. In addition, note the global variables and starting code for the tickStopwatch(), startStopwatch(), stopStopwatch(), and resetStopwatch() functions.
3. In the tickStopwatch() function, add code that adds 10 milliseconds to the elapsedMilliseconds variable and then adjusts the elapsedMinutes and elapsedSeconds variables accordingly. Then, add code that displays the result in the appropriate span tags in the page.
4. In the startStopwatch() function, add code that starts the stopwatch. Be sure to cancel the default action of the link too.
5. In the stopStopwatch() and resetStopwatch() functions, add code that stops the stopwatch. Also, in the resetStopwatch() function, reset the elapsed time and the page display. Be sure to cancel the default action of the links too.
6. In the DOMContentLoaded event handler, attach the stopwatch event handlers to the appropriate links.
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Clock</title>
<link rel="stylesheet" href="clock.css">
</head>
<body>
<main>
<h1>Digital clock with stopwatch</h1>
<fieldset>
<legend>Clock</legend>
<span id="hours"></span>:
<span id="minutes"></span>:
<span id="seconds"></span>
<span id="ampm"></span>
</fieldset>
<fieldset>
<legend>Stop Watch</legend>
<a href="#" id="start">Start</a>
<a href="#" id="stop">Stop</a>
<a href="#" id="reset">Reset</a>
<span id="s_minutes">00</span>:
<span id="s_seconds">00</span>:
<span id="s_ms">000</span>
</fieldset>
</main>
<script src="clock.js"></script>
</body>
</html>
clock.js
"use strict";
const $ = selector => document.querySelector(selector);
const padSingleDigit = num => num.toString().padStart(2, "0");
const displayCurrentTime = () => {
const now = new Date();
const hours = now.getHours();
let ampm = "AM"; // set default value
// correct hours and AM/PM value for display
if (hours > 12) { // convert from military time
hours = hours - 12;
ampm = "PM";
} else { // adjust 12 noon and 12 midnight
switch (hours) {
case 12: // noon
ampm = "PM";
break;
case 0: // midnight
hours = 12;
ampm = "AM";
}
}
$("#hours").textContent = hours;
$("#minutes").textContent = padSingleDigit(now.getMinutes());
$("#seconds").textContent = padSingleDigit(now.getSeconds());
$("#ampm").textContent = ampm;
};
//global stop watch timer variable and elapsed time object
let stopwatchTimer = null;
let elapsedMinutes = 0;
let elapsedSeconds = 0;
let elapsedMilliseconds = 0;
const tickStopwatch = () => {
// increment milliseconds by 10 milliseconds
// if milliseconds total 1000, increment seconds by one and reset milliseconds to zero
// if seconds total 60, increment minutes by one and reset seconds to zero
//display new stopwatch time
};
// event handler functions
const startStopwatch = evt => {
// prevent default action of link
// do first tick of stop watch and then set interval timer to tick
// stop watch every 10 milliseconds. Store timer object in stopwatchTimer
// variable so next two functions can stop timer.
};
const stopStopwatch = evt => {
// prevent default action of link
// stop timer
};
const resetStopwatch = evt => {
// prevent default action of link
// stop timer
// reset elapsed variables and clear stopwatch display
};
document.addEventListener("DOMContentLoaded", () => {
// set initial clock display and then set interval timer to display
// new time every second. Don't store timer object because it
// won't be needed - clock will just run.
displayCurrentTime();
setInterval(displayCurrentTime, 1000);
// set up stopwatch event handlers
});
clock.css
body {
font-family: Arial, Helvetica, sans-serif;
background-color: white;
margin: 0 auto;
width: 450px;
border: 3px solid blue;
padding: 0 2em 1em;
}
h1 {
color: blue;
}
div {
margin-bottom: 1em;
}
label {
display: inline-block;
width: 11em;
text-align: right;
}
input {
margin-left: 1em;
margin-bottom: .5em;
}
fieldset {
margin-bottom: 1em;
}
fieldset a, #seconds {
margin-right: 0.5em;
}
library_event.js
"use strict";
var evt = {
attach: function(node, eventName, func) {
},
detach: function(node, eventName, func) {
},
preventDefault: function(e) {
}
};

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

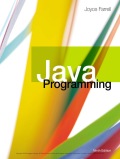
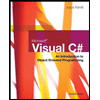
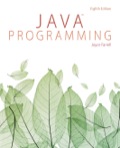
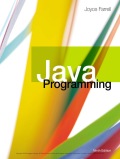
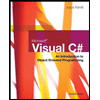
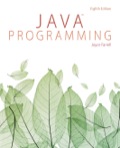
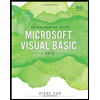