// For an input string of words, find the most frequently occuring word. In case of ties, report any one of them. // Your algorithm should be O(n) time where n is the number of words in the string #include #include #include #include using namespace std; string findWord(vector& tokens); int main() { string line = "I felt happy because I saw the others were happy and because I knew I should feel happy but I wasn’t really happy"; // Convert string to a vector of words char delimiter = ' '; string token; istringstream tokenStream(line); vector tokens; while (getline(tokenStream, token, delimiter)) { tokens.push_back(token); } cout << "The most frequently occuring word is: " << findWord(tokens) << endl; } string findWord(vector& tokens) { // Your code here }
// For an input string of words, find the most frequently occuring word. In case of ties, report any one of them.
// Your
#include <iostream>
#include <
#include <sstream>
#include <unordered_map>
using namespace std;
string findWord(vector<string>& tokens);
int main() {
string line = "I felt happy because I saw the others were happy and because I knew I should feel happy but I wasn’t really happy";
// Convert string to a vector of words
char delimiter = ' ';
string token;
istringstream tokenStream(line);
vector<string> tokens;
while (getline(tokenStream, token, delimiter)) {
tokens.push_back(token);
}
cout << "The most frequently occuring word is: " << findWord(tokens) << endl;
}
string findWord(vector<string>& tokens) {
// Your code here
}

Step by step
Solved in 3 steps with 3 images

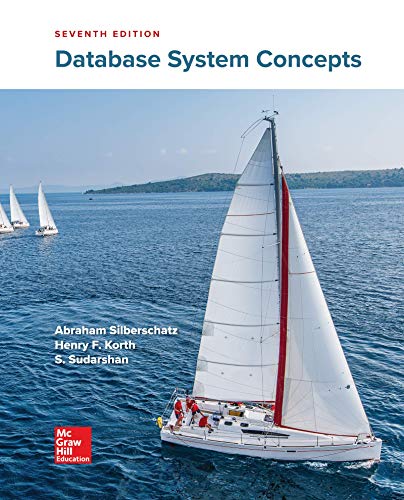
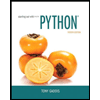
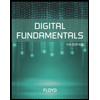
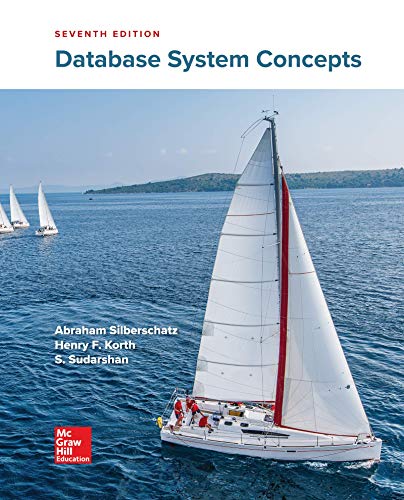
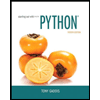
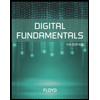
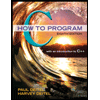
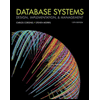
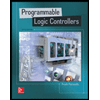