For the code in java below it shows a deck of 52 cards and asks the name of the two players and makes both players draw five cards from the deck. What I want to be added into the code is that both Players are people that can select what card they pick and Player A starts. Player A picks a card in his/her hand. Player B gets to choose the two cards which add to the value of Player A’s card, if player B lies and the two cards they choose do not add up to A's card, player B loses a point, if player B does not have two cards whose value adds to the value of Player A’s card, then no one gets a point. Player’s A card (if selected) and the two cards from Player B are discarded both players draw back to 5 cards from the deck.
For the code in java below it shows a deck of 52 cards and asks the name of the two players and makes both players draw five cards from the deck. What I want to be added into the code is that both Players are people that can select what card they pick and Player A starts. Player A picks a card in his/her hand. Player B gets to choose the two cards which add to the value of Player A’s card, if player B lies and the two cards they choose do not add up to A's card, player B loses a point, if player B does not have two cards whose value adds to the value of Player A’s card, then no one gets a point. Player’s A card (if selected) and the two cards from Player B are discarded both players draw back to 5 cards from the deck.
Main class code:
![Main.java
import java.util.ArrayList;
import java.util.Scanner;
import java.util.List;
import java.util.Random;
2
3
4
6
7
class Main {
public static void main(String[] args) {
// card game, two players, take turns.
string[] suits = {"Hearts", "Clubs", "Spades", "Diamonds"};
string[] numbers = {"A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J",
"о", "к"};
8
9.
10
11
12
13
for(String oneSuit : suits){
for (String num : numbers){
14
15
16
17
System.out.println(oneSuit +
+ num);
18
19
}
}
//add a player to the list
List<Player> listofPlayers = new ArrayList<>();
Scanner sc = new Scanner (System.in);
System.out.println("Name of Player 1");
String PlayerName = sc.nextLine();
Player newPlayer = new Player(PlayerName);
listofplayers.add(newPlayer);
20
21
22
23
24
25
26
27
28
29
//adding another player to the list
system.out.println("Name of Player 2");
30
31
1.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5ce8c54e-2b59-4ae4-af38-4aa6183ed8ad%2Fc602aa1c-b910-41bf-8856-11054eb0280f%2Fncj57ay_processed.png&w=3840&q=75)
![Main.java
system.out.println("Name of Player 2");
PlayerName = sc.nextLine();
Player newPlayer2 = new Player (PlayerName);
listofplayers.add(newPlayer2);
//now choosing Random cards for both players and then store it in the array.
List<string> Player1Cards = new ArrayList<>();
List<string> Player2Cards = new ArrayList<>();|
for(int i=1;i<=5;i++){
int suitIndex1, suitIndex2;
31
32
33
34
35
36
37
38
39
int numbersIndex1,numbersIndex2;
Random random = new Random();
suitIndex1 = random.nextInt(3 - 0) + 0;
numbersIndex1= random.nextInt(12 - 0) + 0;
suitIndex2 = random.nextInt(3 - 0) + 0;
numbersIndex2= random.nextInt(12 - 0) + 0;
40
41
42
43
44
45
46
Player1Cards.add(suits[suitIndex1]+numbers[numbersIndex1]);
Player2Cards.add(suits[suitIndex2]+numbers[numbersIndex2]);
}
//displaying cards choosen
System.out.print("Player "+newPlayer.getName ()+" cards are: ");
for(String card:Player1Cards){
System.out.print(card+" ");
}
System.out.print("\nPlayer "+newPlayer2.getName()+" cards are: ");
for (String card:Player2Cards){
System.out.print(card+" ");
47
48
49
50
51
52
53
54
55
56
57
58
59
}
60](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5ce8c54e-2b59-4ae4-af38-4aa6183ed8ad%2Fc602aa1c-b910-41bf-8856-11054eb0280f%2Funtyx5d_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

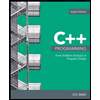
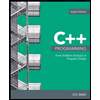