For this assignment, we will correctly handle Fractions that are negative, improper (larger numerator than denominator), or whole numbers (denominator of 1). You should write the class in two phases. I've set it up this way because there is one function that is very difficult and I want to provide a way for you to skip that function and move on if you need to. If you submit the assignment with only phase 1 completed you can still earn almost all of the points. ⚫ Phase 1: Write everything except the extraction operator (>>). To do this, you'll want to comment out that part of the client program that tests the extraction operator. Phase 2 [5 points]: The extraction operator. Your class should support the following operations on Fraction objects: Construction of a Fraction from two, one, or zero integer arguments. If two arguments, they are assumed to be the numerator and denominator, just one is assumed to be a whole number, and zero arguments creates a zero Fraction. Use default parameters so that you only need a single function to implement all three of these constructors. You should check to make sure that the denominator is not set to 0. The easiest way to do this is to use an assert statement: assert(inDenominator != 0); You can put this statement at the top of your constructor. Note that the variable in the assert() is the incoming parameter, not the data member. In order to use assert(), you must #include Printing a Fraction to a stream with an overloaded insertion (<<) operator. The Fraction should be printed in reduced form (not 3/6 but 1/2). Whole numbers should print without a denominator (e.g. not 3/1 but just 3). Improper Fractions should be printed as a mixed number with a + sign between the two parts (2+1/2). Negative Fractions should be printed with a leading minus sign. Reading a Fraction from a stream using an overloaded extraction (>>) operator. You should be able to read any of the formats described above (mixed number, negative, whole numbers). You may assume that there are no spaces or formatting errors in the Fractions that you read. This means, for example, that in a mixed number only the whole number (not the numerator or denominator) may be negative, and that in a fraction with no whole number part only the numerator (not the denominator) may be negative. Note: You may need to exceed 15 lines for this function. My solution is about 20 lines long. • All six of the relational operators (<, <=, >, >=, ==, !=) should be supported. They should be able to compare Fractions to other Fractions as well as Fractions to integers. Either Fractions or integers can appear on either side of the binary comparison operator. You should only use one function for each operator. • • The four basic arithmetic operations (+, -, *, /) should be supported. Again, they should allow Fractions to be combined with other Fractions, as well as with integers. Either Fractions or integers can appear on either side of the binary operator. Only use one function for each operator. Note that no special handling is needed to handle the case of dividing by a Fraction that is equal to 0. If the client attempts to do this, they will get a runtime error, which is the same behavior they would expect if they tried to divide by an int or double that was equal to 0. The shorthand arithmetic assignment operators (+=, -=, *=, /=) should also be implemented. Fractions can appear on the left-hand side, and Fractions or integers on the right-hand side. Only use one function for each operator. The increment and decrement (++, --) operators should be supported in both prefix and postfix form for Fractions. To increment or decrement a Fraction means to add or subtract (respectively) one (1). Add a private "simplify()" function to your class and call it from the appropriate member functions. The best way to do this is to make the function a void function with no parameters that reduces the calling object. Recall that "simplifying" or "reducing" a Fraction is a separate task from converting it from an improper Fraction to a mixed number. Make sure you keep those two tasks separate in your mind. Make sure that your class will reduce ANY Fraction, not just the Fractions that are tested in the provided client program. Fractions should not be simply reduced upon output, they should be stored in reduced form at all times. In other words, you should ensure that all Fraction objects are reduced before the end of any member function. To put it yet another way: each member function (other than simplify() itself) must be able to assume that all Fraction objects are in simple form when it begins execution. You must create your own algorithm for reducing Fractions. Don't look up an already existing algorithm for reducing Fractions or finding GCF. The point here is to have you practice solving the problem on your own. In particular, don't use Euclid's algorithm. Don't worry about being efficient. It's fine to have your function check every possible factor, even if it would be more efficient to just check prime numbers. Just create something of your own that works correctly on ANY Fraction. Your simplify() function should also ensure that the denominator is never negative. If the denominator is negative, fix this by multiplying numerator and denominator by -1. Also, if the numerator is 0, the denominator should be set to 1. Additional Requirements and Hints: • • The name of your class must be "Fraction". No variations will work. Your class must be split into two files, a header file and an implementation file. You must place your header file and implementation file in a namespace. Normally one would call a namespace something more likely to be unique, but for purposes of convenience we will all call our namespace "cs_fraction". • Use exactly two data members. • • • • You should not compare two Fractions by dividing the numerator by the denominator. This is not guaranteed to give you the correct result every time, because of the way that double values are stored internally by the computer. I would cross multiply and compare the products. Don't go to a lot of trouble to find the common denominator (when adding or subtracting). Simply multiply the denominators together. The last two bullets bring up an interesting issue: if your denominators are really big, multiplying them together (or cross multiplying) may give you a number that is too big to store in an int variable. This is called overflow. The rule for this assignment is: don't worry about overflow in these two situations. Your class must guarantee that Fractions are stored in reduced form at all times, not just when they are output. In other words, each member function must be able to assume that all Fraction objects are in simple form when they begin execution. The '+' in mixed numbers does not mean add. It is simply a separator (to separate the integer part from the Fraction part of the number). So the Fraction "negative two and one-sixth" would be written as -2+1/6, even though -2 plus 1/6 is not what we mean. Extraction Operator Hints: ° Since your extraction operator should not consume anything after the end of the Fraction being read, you will probably want to use the .peek() function to look ahead in the input stream and see what the next character is after the first number is read. If it's not either a '/" or a '+', then you are done reading and should read no further. I have something like this: int temp; in >> temp; if (in.peek() == '+') { doSomething... } else if (in. peek() doSomething Else... } else { '/'){ } doThird Option ° You don't need to detect or read the minus operator as a separate character. When you use the extraction operator to read an int, it will interpret a leading minus sign correctly. So, for example, you shouldn't have "if (in.peek() == '-')" ° The peek() function does not consume the character from the input stream, so after you use it to detect what the next character is, the first thing you need to do is get past that character. One good way to do that would be to use in.ignore(), which ignores one single character in the input stream. ° Here is one example of how processing will work: if the fraction in the input stream is a mixed number, you will need to have at least three input operations so that you can read all three integers in the mixed number. ° You can model the syntax of your operator>>() function header after the operator<<() function syntax you have seen in examples. Just use istream in the place of ostream (since we are doing input instead of output). Also, keep in mind that the right operand will NOT be const since the extraction operator's purpose is to modify the right operand. About comments: Don't forget to review Style Convention 1D, where commenting guidelines for classes are described in detail. In particular, every public member function (and friend function), however simple, must have a precondition (if there is one) and a postcondition listed in the header file. Only the most complex of your function definitions will need additional comment (and that would appear in the implementation file). My solution had 22 functions (including friend functions). All but three of them (<<, >>, and simplify()) were less than 4 lines long. I'm not saying yours has to be like this, but it shouldn't be way off. My simplify() and << have about 10 - 14 lines. >> has 21 lines. This is including declarations and lines that have nothing but a close curly brace. Do not use as a resource a supplementary text or website if it includes a Fraction class (or rational or ratio or whatever).
For this assignment, we will correctly handle Fractions that are negative, improper (larger numerator than denominator), or whole numbers (denominator of 1). You should write the class in two phases. I've set it up this way because there is one function that is very difficult and I want to provide a way for you to skip that function and move on if you need to. If you submit the assignment with only phase 1 completed you can still earn almost all of the points. ⚫ Phase 1: Write everything except the extraction operator (>>). To do this, you'll want to comment out that part of the client program that tests the extraction operator. Phase 2 [5 points]: The extraction operator. Your class should support the following operations on Fraction objects: Construction of a Fraction from two, one, or zero integer arguments. If two arguments, they are assumed to be the numerator and denominator, just one is assumed to be a whole number, and zero arguments creates a zero Fraction. Use default parameters so that you only need a single function to implement all three of these constructors. You should check to make sure that the denominator is not set to 0. The easiest way to do this is to use an assert statement: assert(inDenominator != 0); You can put this statement at the top of your constructor. Note that the variable in the assert() is the incoming parameter, not the data member. In order to use assert(), you must #include Printing a Fraction to a stream with an overloaded insertion (<<) operator. The Fraction should be printed in reduced form (not 3/6 but 1/2). Whole numbers should print without a denominator (e.g. not 3/1 but just 3). Improper Fractions should be printed as a mixed number with a + sign between the two parts (2+1/2). Negative Fractions should be printed with a leading minus sign. Reading a Fraction from a stream using an overloaded extraction (>>) operator. You should be able to read any of the formats described above (mixed number, negative, whole numbers). You may assume that there are no spaces or formatting errors in the Fractions that you read. This means, for example, that in a mixed number only the whole number (not the numerator or denominator) may be negative, and that in a fraction with no whole number part only the numerator (not the denominator) may be negative. Note: You may need to exceed 15 lines for this function. My solution is about 20 lines long. • All six of the relational operators (<, <=, >, >=, ==, !=) should be supported. They should be able to compare Fractions to other Fractions as well as Fractions to integers. Either Fractions or integers can appear on either side of the binary comparison operator. You should only use one function for each operator. • • The four basic arithmetic operations (+, -, *, /) should be supported. Again, they should allow Fractions to be combined with other Fractions, as well as with integers. Either Fractions or integers can appear on either side of the binary operator. Only use one function for each operator. Note that no special handling is needed to handle the case of dividing by a Fraction that is equal to 0. If the client attempts to do this, they will get a runtime error, which is the same behavior they would expect if they tried to divide by an int or double that was equal to 0. The shorthand arithmetic assignment operators (+=, -=, *=, /=) should also be implemented. Fractions can appear on the left-hand side, and Fractions or integers on the right-hand side. Only use one function for each operator. The increment and decrement (++, --) operators should be supported in both prefix and postfix form for Fractions. To increment or decrement a Fraction means to add or subtract (respectively) one (1). Add a private "simplify()" function to your class and call it from the appropriate member functions. The best way to do this is to make the function a void function with no parameters that reduces the calling object. Recall that "simplifying" or "reducing" a Fraction is a separate task from converting it from an improper Fraction to a mixed number. Make sure you keep those two tasks separate in your mind. Make sure that your class will reduce ANY Fraction, not just the Fractions that are tested in the provided client program. Fractions should not be simply reduced upon output, they should be stored in reduced form at all times. In other words, you should ensure that all Fraction objects are reduced before the end of any member function. To put it yet another way: each member function (other than simplify() itself) must be able to assume that all Fraction objects are in simple form when it begins execution. You must create your own algorithm for reducing Fractions. Don't look up an already existing algorithm for reducing Fractions or finding GCF. The point here is to have you practice solving the problem on your own. In particular, don't use Euclid's algorithm. Don't worry about being efficient. It's fine to have your function check every possible factor, even if it would be more efficient to just check prime numbers. Just create something of your own that works correctly on ANY Fraction. Your simplify() function should also ensure that the denominator is never negative. If the denominator is negative, fix this by multiplying numerator and denominator by -1. Also, if the numerator is 0, the denominator should be set to 1. Additional Requirements and Hints: • • The name of your class must be "Fraction". No variations will work. Your class must be split into two files, a header file and an implementation file. You must place your header file and implementation file in a namespace. Normally one would call a namespace something more likely to be unique, but for purposes of convenience we will all call our namespace "cs_fraction". • Use exactly two data members. • • • • You should not compare two Fractions by dividing the numerator by the denominator. This is not guaranteed to give you the correct result every time, because of the way that double values are stored internally by the computer. I would cross multiply and compare the products. Don't go to a lot of trouble to find the common denominator (when adding or subtracting). Simply multiply the denominators together. The last two bullets bring up an interesting issue: if your denominators are really big, multiplying them together (or cross multiplying) may give you a number that is too big to store in an int variable. This is called overflow. The rule for this assignment is: don't worry about overflow in these two situations. Your class must guarantee that Fractions are stored in reduced form at all times, not just when they are output. In other words, each member function must be able to assume that all Fraction objects are in simple form when they begin execution. The '+' in mixed numbers does not mean add. It is simply a separator (to separate the integer part from the Fraction part of the number). So the Fraction "negative two and one-sixth" would be written as -2+1/6, even though -2 plus 1/6 is not what we mean. Extraction Operator Hints: ° Since your extraction operator should not consume anything after the end of the Fraction being read, you will probably want to use the .peek() function to look ahead in the input stream and see what the next character is after the first number is read. If it's not either a '/" or a '+', then you are done reading and should read no further. I have something like this: int temp; in >> temp; if (in.peek() == '+') { doSomething... } else if (in. peek() doSomething Else... } else { '/'){ } doThird Option ° You don't need to detect or read the minus operator as a separate character. When you use the extraction operator to read an int, it will interpret a leading minus sign correctly. So, for example, you shouldn't have "if (in.peek() == '-')" ° The peek() function does not consume the character from the input stream, so after you use it to detect what the next character is, the first thing you need to do is get past that character. One good way to do that would be to use in.ignore(), which ignores one single character in the input stream. ° Here is one example of how processing will work: if the fraction in the input stream is a mixed number, you will need to have at least three input operations so that you can read all three integers in the mixed number. ° You can model the syntax of your operator>>() function header after the operator<<() function syntax you have seen in examples. Just use istream in the place of ostream (since we are doing input instead of output). Also, keep in mind that the right operand will NOT be const since the extraction operator's purpose is to modify the right operand. About comments: Don't forget to review Style Convention 1D, where commenting guidelines for classes are described in detail. In particular, every public member function (and friend function), however simple, must have a precondition (if there is one) and a postcondition listed in the header file. Only the most complex of your function definitions will need additional comment (and that would appear in the implementation file). My solution had 22 functions (including friend functions). All but three of them (<<, >>, and simplify()) were less than 4 lines long. I'm not saying yours has to be like this, but it shouldn't be way off. My simplify() and << have about 10 - 14 lines. >> has 21 lines. This is including declarations and lines that have nothing but a close curly brace. Do not use as a resource a supplementary text or website if it includes a Fraction class (or rational or ratio or whatever).
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 12PE
Related questions
Question
Provide full C++ main.cpp, fraction.cpp, fraction.h
![For this assignment, we will correctly handle Fractions that are negative, improper (larger numerator than denominator), or whole numbers (denominator of 1).
You should write the class in two phases. I've set it up this way because there is one function that is very difficult and I want to provide a way for you to skip that function and move on if you need to. If you submit the assignment with only phase 1 completed you can still earn almost all of the points.
⚫ Phase 1: Write everything except the extraction operator (>>). To do this, you'll want to comment out that part of the client program that tests the extraction operator.
Phase 2 [5 points]: The extraction operator.
Your class should support the following operations on Fraction objects:
Construction of a Fraction from two, one, or zero integer arguments. If two arguments, they are assumed to be the numerator and denominator, just one is assumed to be a whole number, and zero arguments creates a zero Fraction. Use default parameters so that you only need a single function to implement all three of these constructors.
You should check to make sure that the denominator is not set to 0. The easiest way to do this is to use an assert statement: assert(inDenominator != 0); You can put this statement at the top of your constructor. Note that the variable in the assert() is the incoming parameter, not the data member. In order to use assert(), you must #include
<cassert>
Printing a Fraction to a stream with an overloaded insertion (<<) operator. The Fraction should be printed in reduced form (not 3/6 but 1/2). Whole numbers should print without a denominator (e.g. not 3/1 but just 3). Improper Fractions should be printed as a mixed number with a + sign between the two parts (2+1/2). Negative Fractions
should be printed with a leading minus sign.
Reading a Fraction from a stream using an overloaded extraction (>>) operator. You should be able to read any of the formats described above (mixed number, negative, whole numbers). You may assume that there are no spaces or formatting errors in the Fractions that you read. This means, for example, that in a mixed number only the whole
number (not the numerator or denominator) may be negative, and that in a fraction with no whole number part only the numerator (not the denominator) may be negative. Note: You may need to exceed 15 lines for this function. My solution is about 20 lines long.
• All six of the relational operators (<, <=, >, >=, ==, !=) should be supported. They should be able to compare Fractions to other Fractions as well as Fractions to integers. Either Fractions or integers can appear on either side of the binary comparison operator. You should only use one function for each operator.
•
•
The four basic arithmetic operations (+, -, *, /) should be supported. Again, they should allow Fractions to be combined with other Fractions, as well as with integers. Either Fractions or integers can appear on either side of the binary operator. Only use one function for each operator.
Note that no special handling is needed to handle the case of dividing by a Fraction that is equal to 0. If the client attempts to do this, they will get a runtime error, which is the same behavior they would expect if they tried to divide by an int or double that was equal to 0.
The shorthand arithmetic assignment operators (+=, -=, *=, /=) should also be implemented. Fractions can appear on the left-hand side, and Fractions or integers on the right-hand side. Only use one function for each operator.
The increment and decrement (++, --) operators should be supported in both prefix and postfix form for Fractions. To increment or decrement a Fraction means to add or subtract (respectively) one (1).
Add a private "simplify()" function to your class and call it from the appropriate member functions. The best way to do this is to make the function a void function with no parameters that reduces the calling object.
Recall that "simplifying" or "reducing" a Fraction is a separate task from converting it from an improper Fraction to a mixed number. Make sure you keep those two tasks separate in your mind.
Make sure that your class will reduce ANY Fraction, not just the Fractions that are tested in the provided client program. Fractions should not be simply reduced upon output, they should be stored in reduced form at all times. In other words, you should ensure that all Fraction objects are reduced before the end of any member function. To put it
yet another way: each member function (other than simplify() itself) must be able to assume that all Fraction objects are in simple form when it begins execution.
You must create your own algorithm for reducing Fractions. Don't look up an already existing algorithm for reducing Fractions or finding GCF. The point here is to have you practice solving the problem on your own. In particular, don't use Euclid's algorithm. Don't worry about being efficient. It's fine to have your function check every possible factor,
even if it would be more efficient to just check prime numbers. Just create something of your own that works correctly on ANY Fraction.
Your simplify() function should also ensure that the denominator is never negative. If the denominator is negative, fix this by multiplying numerator and denominator by -1. Also, if the numerator is 0, the denominator should be set to 1.
Additional Requirements and Hints:
•
•
The name of your class must be "Fraction". No variations will work.
Your class must be split into two files, a header file and an implementation file.
You must place your header file and implementation file in a namespace. Normally one would call a namespace something more likely to be unique, but for purposes of convenience we will all call our namespace "cs_fraction".
• Use exactly two data members.
•
•
•
•
You should not compare two Fractions by dividing the numerator by the denominator. This is not guaranteed to give you the correct result every time, because of the way that double values are stored internally by the computer. I would cross multiply and compare the products.
Don't go to a lot of trouble to find the common denominator (when adding or subtracting). Simply multiply the denominators together.
The last two bullets bring up an interesting issue: if your denominators are really big, multiplying them together (or cross multiplying) may give you a number that is too big to store in an int variable. This is called overflow. The rule for this assignment is: don't worry about overflow in these two situations.
Your class must guarantee that Fractions are stored in reduced form at all times, not just when they are output. In other words, each member function must be able to assume that all Fraction objects are in simple form when they begin execution.
The '+' in mixed numbers does not mean add. It is simply a separator (to separate the integer part from the Fraction part of the number). So the Fraction "negative two and one-sixth" would be written as -2+1/6, even though -2 plus 1/6 is not what we mean.
Extraction Operator Hints:
° Since your extraction operator should not consume anything after the end of the Fraction being read, you will probably want to use the .peek() function to look ahead in the input stream and see what the next character is after the first number is read. If it's not either a '/" or a '+', then you are done reading and should read no further. I
have something like this:
int temp;
in >> temp;
if (in.peek() == '+') {
doSomething...
} else if (in. peek()
doSomething Else...
} else {
'/'){
}
doThird Option
° You don't need to detect or read the minus operator as a separate character. When you use the extraction operator to read an int, it will interpret a leading minus sign correctly. So, for example, you shouldn't have "if (in.peek() == '-')"
° The peek() function does not consume the character from the input stream, so after you use it to detect what the next character is, the first thing you need to do is get past that character. One good way to do that would be to use in.ignore(), which ignores one single character in the input stream.
° Here is one example of how processing will work: if the fraction in the input stream is a mixed number, you will need to have at least three input operations so that you can read all three integers in the mixed number.
°
You can model the syntax of your operator>>() function header after the operator<<() function syntax you have seen in examples. Just use istream in the place of ostream (since we are doing input instead of output). Also, keep in mind that the right operand will NOT be const since the extraction operator's purpose is to modify the right
operand.
About comments: Don't forget to review Style Convention 1D, where commenting guidelines for classes are described in detail. In particular, every public member function (and friend function), however simple, must have a precondition (if there is one) and a postcondition listed in the header file. Only the most complex of your function definitions
will need additional comment (and that would appear in the implementation file).
My solution had 22 functions (including friend functions). All but three of them (<<, >>, and simplify()) were less than 4 lines long. I'm not saying yours has to be like this, but it shouldn't be way off. My simplify() and << have about 10 - 14 lines. >> has 21 lines. This is including declarations and lines that have nothing but a close curly brace.
Do not use as a resource a supplementary text or website if it includes a Fraction class (or rational or ratio or whatever).](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1bfda259-7429-4717-b69c-7067c249bba0%2Feee7bc4e-eadf-4a12-97a9-005636d358af%2F1gkg52r_processed.png&w=3840&q=75)
Transcribed Image Text:For this assignment, we will correctly handle Fractions that are negative, improper (larger numerator than denominator), or whole numbers (denominator of 1).
You should write the class in two phases. I've set it up this way because there is one function that is very difficult and I want to provide a way for you to skip that function and move on if you need to. If you submit the assignment with only phase 1 completed you can still earn almost all of the points.
⚫ Phase 1: Write everything except the extraction operator (>>). To do this, you'll want to comment out that part of the client program that tests the extraction operator.
Phase 2 [5 points]: The extraction operator.
Your class should support the following operations on Fraction objects:
Construction of a Fraction from two, one, or zero integer arguments. If two arguments, they are assumed to be the numerator and denominator, just one is assumed to be a whole number, and zero arguments creates a zero Fraction. Use default parameters so that you only need a single function to implement all three of these constructors.
You should check to make sure that the denominator is not set to 0. The easiest way to do this is to use an assert statement: assert(inDenominator != 0); You can put this statement at the top of your constructor. Note that the variable in the assert() is the incoming parameter, not the data member. In order to use assert(), you must #include
<cassert>
Printing a Fraction to a stream with an overloaded insertion (<<) operator. The Fraction should be printed in reduced form (not 3/6 but 1/2). Whole numbers should print without a denominator (e.g. not 3/1 but just 3). Improper Fractions should be printed as a mixed number with a + sign between the two parts (2+1/2). Negative Fractions
should be printed with a leading minus sign.
Reading a Fraction from a stream using an overloaded extraction (>>) operator. You should be able to read any of the formats described above (mixed number, negative, whole numbers). You may assume that there are no spaces or formatting errors in the Fractions that you read. This means, for example, that in a mixed number only the whole
number (not the numerator or denominator) may be negative, and that in a fraction with no whole number part only the numerator (not the denominator) may be negative. Note: You may need to exceed 15 lines for this function. My solution is about 20 lines long.
• All six of the relational operators (<, <=, >, >=, ==, !=) should be supported. They should be able to compare Fractions to other Fractions as well as Fractions to integers. Either Fractions or integers can appear on either side of the binary comparison operator. You should only use one function for each operator.
•
•
The four basic arithmetic operations (+, -, *, /) should be supported. Again, they should allow Fractions to be combined with other Fractions, as well as with integers. Either Fractions or integers can appear on either side of the binary operator. Only use one function for each operator.
Note that no special handling is needed to handle the case of dividing by a Fraction that is equal to 0. If the client attempts to do this, they will get a runtime error, which is the same behavior they would expect if they tried to divide by an int or double that was equal to 0.
The shorthand arithmetic assignment operators (+=, -=, *=, /=) should also be implemented. Fractions can appear on the left-hand side, and Fractions or integers on the right-hand side. Only use one function for each operator.
The increment and decrement (++, --) operators should be supported in both prefix and postfix form for Fractions. To increment or decrement a Fraction means to add or subtract (respectively) one (1).
Add a private "simplify()" function to your class and call it from the appropriate member functions. The best way to do this is to make the function a void function with no parameters that reduces the calling object.
Recall that "simplifying" or "reducing" a Fraction is a separate task from converting it from an improper Fraction to a mixed number. Make sure you keep those two tasks separate in your mind.
Make sure that your class will reduce ANY Fraction, not just the Fractions that are tested in the provided client program. Fractions should not be simply reduced upon output, they should be stored in reduced form at all times. In other words, you should ensure that all Fraction objects are reduced before the end of any member function. To put it
yet another way: each member function (other than simplify() itself) must be able to assume that all Fraction objects are in simple form when it begins execution.
You must create your own algorithm for reducing Fractions. Don't look up an already existing algorithm for reducing Fractions or finding GCF. The point here is to have you practice solving the problem on your own. In particular, don't use Euclid's algorithm. Don't worry about being efficient. It's fine to have your function check every possible factor,
even if it would be more efficient to just check prime numbers. Just create something of your own that works correctly on ANY Fraction.
Your simplify() function should also ensure that the denominator is never negative. If the denominator is negative, fix this by multiplying numerator and denominator by -1. Also, if the numerator is 0, the denominator should be set to 1.
Additional Requirements and Hints:
•
•
The name of your class must be "Fraction". No variations will work.
Your class must be split into two files, a header file and an implementation file.
You must place your header file and implementation file in a namespace. Normally one would call a namespace something more likely to be unique, but for purposes of convenience we will all call our namespace "cs_fraction".
• Use exactly two data members.
•
•
•
•
You should not compare two Fractions by dividing the numerator by the denominator. This is not guaranteed to give you the correct result every time, because of the way that double values are stored internally by the computer. I would cross multiply and compare the products.
Don't go to a lot of trouble to find the common denominator (when adding or subtracting). Simply multiply the denominators together.
The last two bullets bring up an interesting issue: if your denominators are really big, multiplying them together (or cross multiplying) may give you a number that is too big to store in an int variable. This is called overflow. The rule for this assignment is: don't worry about overflow in these two situations.
Your class must guarantee that Fractions are stored in reduced form at all times, not just when they are output. In other words, each member function must be able to assume that all Fraction objects are in simple form when they begin execution.
The '+' in mixed numbers does not mean add. It is simply a separator (to separate the integer part from the Fraction part of the number). So the Fraction "negative two and one-sixth" would be written as -2+1/6, even though -2 plus 1/6 is not what we mean.
Extraction Operator Hints:
° Since your extraction operator should not consume anything after the end of the Fraction being read, you will probably want to use the .peek() function to look ahead in the input stream and see what the next character is after the first number is read. If it's not either a '/" or a '+', then you are done reading and should read no further. I
have something like this:
int temp;
in >> temp;
if (in.peek() == '+') {
doSomething...
} else if (in. peek()
doSomething Else...
} else {
'/'){
}
doThird Option
° You don't need to detect or read the minus operator as a separate character. When you use the extraction operator to read an int, it will interpret a leading minus sign correctly. So, for example, you shouldn't have "if (in.peek() == '-')"
° The peek() function does not consume the character from the input stream, so after you use it to detect what the next character is, the first thing you need to do is get past that character. One good way to do that would be to use in.ignore(), which ignores one single character in the input stream.
° Here is one example of how processing will work: if the fraction in the input stream is a mixed number, you will need to have at least three input operations so that you can read all three integers in the mixed number.
°
You can model the syntax of your operator>>() function header after the operator<<() function syntax you have seen in examples. Just use istream in the place of ostream (since we are doing input instead of output). Also, keep in mind that the right operand will NOT be const since the extraction operator's purpose is to modify the right
operand.
About comments: Don't forget to review Style Convention 1D, where commenting guidelines for classes are described in detail. In particular, every public member function (and friend function), however simple, must have a precondition (if there is one) and a postcondition listed in the header file. Only the most complex of your function definitions
will need additional comment (and that would appear in the implementation file).
My solution had 22 functions (including friend functions). All but three of them (<<, >>, and simplify()) were less than 4 lines long. I'm not saying yours has to be like this, but it shouldn't be way off. My simplify() and << have about 10 - 14 lines. >> has 21 lines. This is including declarations and lines that have nothing but a close curly brace.
Do not use as a resource a supplementary text or website if it includes a Fraction class (or rational or ratio or whatever).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
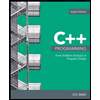
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
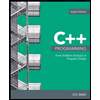
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning