A LinkNode structure or class which will have two attributes - a data attribute and a pointer attribute to the next node. The data attribute of the LinkNode should be the Money class of Lab 1. A Singly Linked List class which will be composed of three attributes - a count attribute, a LinkedNode pointer/reference attribute pointing to the start of the list and a LinkedNode pointer/reference attribute pointing to the end of the list. Since this is a class, make sure all these attributes are private. The attribute names for the Node and Linked List are the words in bold in #1 and #2. For the Linked List, implement the most common linked-list behaviors as explained in class - getters/setters/constructors/destructors for the attributes of the class, (a) create new list, (b) add data, (c) delete data, (d) find data, (e) count of data items in the list, (f) is list empty, (g) empty/destroy the list and (h) print all data items in a list. Note: create new list can be the constructor and destroy list can be the destructor or garbage collector. Note 2: It will be helpful if you define specific add/delete/find methods for the first and last position of the list. Any other methods you think would be useful in your program. A Stack class derived from the Linked List but with no additional attributes and the usual stack methods - (a) create stack, (b) push, (c) pop, (d) peek, and (e) destroy/empty the stack. Similar to above, create can be the constructor etc. A Queue class derived from the Linked List but with no additional attributes and the usual queue methods - (a) create queue, (b) enqueue, (c) dequeue, (d) peekFront, (e) peekRear, and (f) destroy/empty the queue. Similar to above, create can be the constructor etc. Ensure that all your classes are mimimal and cohesive with adequate walls around them. Try to reuse and not duplicate any code. You are allowed to define interfaces as needed, so you can reuse them in your project. Then write a main driver program that will demonstrate all the capabilities of your ADTs as follows - Create seven (7) Node objects of Money class to be used in the program. Demonstrate the use of your LinkedList with the seven USD Node objects inserted/deleted/searched in a random order. Remember, you will need to print the contents of the List several times to demonstrate the functionality. Demonstrate the use of your Stack with the same seven USD Node objects pushed/popped/peeked in a random order different from the one in LinkedList. Demonstrate the use of your Queue with the same seven USD Node objects enqueued/dequeued/peeked in a random order different from both the LinkedList and Stack. Demonstrate use of all the methods above by providing sufficient information to screen for actions being taken. Restrict all your input / output to the main driver program only. For submission, code your LinkNode and LinkedList in one file and Stack and Queue in a separate file. Remember to include the code file(s) for your Currency and Money classes. Also, your 'main' which is the driver program should be in a separate file of its own. Additionally, you will include your output text file as well as screenshots of the console run. Remember to include pre/post documentation and comment blocks as necessary in your code files. The codes are stored in the following link, use them to complete the assignment: https://github.com/LunaMercer/Lab322C
A LinkNode structure or class which will have two attributes - a data attribute and a pointer attribute to the next node. The data attribute of the LinkNode should be the Money class of Lab 1. A Singly Linked List class which will be composed of three attributes - a count attribute, a LinkedNode pointer/reference attribute pointing to the start of the list and a LinkedNode pointer/reference attribute pointing to the end of the list. Since this is a class, make sure all these attributes are private. The attribute names for the Node and Linked List are the words in bold in #1 and #2. For the Linked List, implement the most common linked-list behaviors as explained in class - getters/setters/constructors/destructors for the attributes of the class, (a) create new list, (b) add data, (c) delete data, (d) find data, (e) count of data items in the list, (f) is list empty, (g) empty/destroy the list and (h) print all data items in a list. Note: create new list can be the constructor and destroy list can be the destructor or garbage collector. Note 2: It will be helpful if you define specific add/delete/find methods for the first and last position of the list. Any other methods you think would be useful in your program. A Stack class derived from the Linked List but with no additional attributes and the usual stack methods - (a) create stack, (b) push, (c) pop, (d) peek, and (e) destroy/empty the stack. Similar to above, create can be the constructor etc. A Queue class derived from the Linked List but with no additional attributes and the usual queue methods - (a) create queue, (b) enqueue, (c) dequeue, (d) peekFront, (e) peekRear, and (f) destroy/empty the queue. Similar to above, create can be the constructor etc. Ensure that all your classes are mimimal and cohesive with adequate walls around them. Try to reuse and not duplicate any code. You are allowed to define interfaces as needed, so you can reuse them in your project. Then write a main driver program that will demonstrate all the capabilities of your ADTs as follows - Create seven (7) Node objects of Money class to be used in the program. Demonstrate the use of your LinkedList with the seven USD Node objects inserted/deleted/searched in a random order. Remember, you will need to print the contents of the List several times to demonstrate the functionality. Demonstrate the use of your Stack with the same seven USD Node objects pushed/popped/peeked in a random order different from the one in LinkedList. Demonstrate the use of your Queue with the same seven USD Node objects enqueued/dequeued/peeked in a random order different from both the LinkedList and Stack. Demonstrate use of all the methods above by providing sufficient information to screen for actions being taken. Restrict all your input / output to the main driver program only. For submission, code your LinkNode and LinkedList in one file and Stack and Queue in a separate file. Remember to include the code file(s) for your Currency and Money classes. Also, your 'main' which is the driver program should be in a separate file of its own. Additionally, you will include your output text file as well as screenshots of the console run. Remember to include pre/post documentation and comment blocks as necessary in your code files. The codes are stored in the following link, use them to complete the assignment: https://github.com/LunaMercer/Lab322C
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
For this assignment, you need to implement link-based List and derivative ADTs in Java. To complete this, you will need the following:
- A LinkNode structure or class which will have two attributes - a data attribute and a pointer attribute to the next node. The data attribute of the LinkNode should be the Money class of Lab 1.
- A Singly Linked List class which will be composed of three attributes - a count attribute, a LinkedNode pointer/reference attribute pointing to the start of the list and a LinkedNode pointer/reference attribute pointing to the end of the list. Since this is a class, make sure all these attributes are private.
- The attribute names for the Node and Linked List are the words in bold in #1 and #2.
- For the Linked List, implement the most common linked-list behaviors as explained in class -
- getters/setters/constructors/destructors for the attributes of the class,
- (a) create new list, (b) add data, (c) delete data, (d) find data, (e) count of data items in the list, (f) is list empty, (g) empty/destroy the list and (h) print all data items in a list. Note: create new list can be the constructor and destroy list can be the destructor or garbage collector. Note 2: It will be helpful if you define specific add/delete/find methods for the first and last position of the list.
- Any other methods you think would be useful in your program.
- A Stack class derived from the Linked List but with no additional attributes and the usual stack methods - (a) create stack, (b) push, (c) pop, (d) peek, and (e) destroy/empty the stack. Similar to above, create can be the constructor etc.
- A Queue class derived from the Linked List but with no additional attributes and the usual queue methods - (a) create queue, (b) enqueue, (c) dequeue, (d) peekFront, (e) peekRear, and (f) destroy/empty the queue. Similar to above, create can be the constructor etc.
- Ensure that all your classes are mimimal and cohesive with adequate walls around them. Try to reuse and not duplicate any code. You are allowed to define interfaces as needed, so you can reuse them in your project.
- Then write a main driver program that will demonstrate all the capabilities of your ADTs as follows -
- Create seven (7) Node objects of Money class to be used in the program.
- Demonstrate the use of your LinkedList with the seven USD Node objects inserted/deleted/searched in a random order. Remember, you will need to print the contents of the List several times to demonstrate the functionality.
- Demonstrate the use of your Stack with the same seven USD Node objects pushed/popped/peeked in a random order different from the one in LinkedList.
- Demonstrate the use of your Queue with the same seven USD Node objects enqueued/dequeued/peeked in a random order different from both the LinkedList and Stack.
- Demonstrate use of all the methods above by providing sufficient information to screen for actions being taken.
- Restrict all your input / output to the main driver program only.
- For submission, code your LinkNode and LinkedList in one file and Stack and Queue in a separate file. Remember to include the code file(s) for your Currency and Money classes. Also, your 'main' which is the driver program should be in a separate file of its own. Additionally, you will include your output text file as well as screenshots of the console run.
- Remember to include pre/post documentation and comment blocks as necessary in your code files.
The codes are stored in the following link, use them to complete the assignment: https://github.com/LunaMercer/Lab322C
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 1 images

Recommended textbooks for you
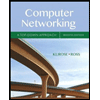
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
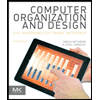
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
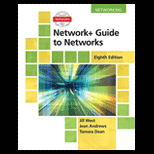
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
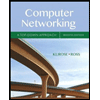
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
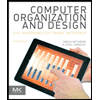
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
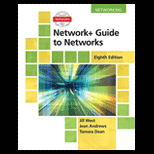
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
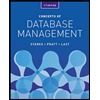
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
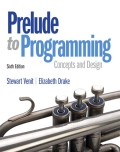
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
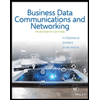
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY