For this assignment, you will be programming an airport simulator. The airport has one runway, and a plane can either be taking off or landing on it at any given unit of time. There will be a queue of planes waiting to take off and a priority queue of planes waiting to land. Your simulator will share some similarities to the movie theater simulator from the lecture notes (and the code is posted on Canvas). To make this project much simpler, I recommend studying that code and basing your code off of it. When the user starts the simulation, let them decide the % chance that a plane will show up to land and the % chance that a plane will show up to take off each clock tick. Let them also decide how many clock ticks to run the simulation for. Recall that the simulation should run inside a for loop. Each loop iteration represents one unit of time (or clock tick). In each clock tick, you need to: Randomly decide if a plane should join the take-off queue, based on the percentage provided by the user. Randomly decide if a plane should join the landing priority queue, based on the percentage provided by the user. For this assignment, you will create a Plane class. Any Plane object joining the landing priority queue should have a random amount of remaining fuel assigned to it. This should range from about 5 to 15 time-units of fuel. Planes with less fuel upon arrival have a higher priority than planes with more fuel. Note: Your Plane class needs to implement Comparable. When a Plane leaves the priority queue and begins to land, determine how long it had been in the queue. If it was in the queue for longer than the fuel it had upon arrival, it crashes. A crashed plane doesn't count as a landed plane. Each plane has a "transaction time" (i.e. how long it takes for a plane to land or take off). When a plane object is created, give it a random transaction time between 1 and 3. While a plane is landing or taking off, the runway is considered in use, and no other plane is allowed on the runway. When the runway is done being used, the landing priority queue is checked. If it contains any planes, the next one is selected to land. If there are no planes in the landing queue, the next plane in the take off queue may do so. Record interesting statistics from your simulation and print them to the console. For example: What is the average time spent waiting for take off? What is the longest time spent waiting for take off? What is the average time spent waiting to land? What is the longest time spent waiting to land? How many planes crashed? How many planes total took off and landed during the simulation? If you do not like the results you are getting (i.e. some planes planes crashed, no planes were able to take off, etc) feel free to tweak your numbers until the results are more realistic. For the landing priority queue, use the priority queue you created in part 1. For the take off queue, you may use the ArrayQueue code developed in class.
For this assignment, you will be programming an airport simulator. The airport has one runway, and a plane can either be taking off or landing on it at any given unit of time. There will be a queue of planes waiting to take off and a priority queue of planes waiting to land.
Your simulator will share some similarities to the movie theater simulator from the lecture notes (and the code is posted on Canvas). To make this project much simpler, I recommend studying that code and basing your code off of it.
When the user starts the simulation, let them decide the % chance that a plane will show up to land and the % chance that a plane will show up to take off each clock tick. Let them also decide how many clock ticks to run the simulation for. Recall that the simulation should run inside a for loop. Each loop iteration represents one unit of time (or clock tick). In each clock tick, you need to:
- Randomly decide if a plane should join the take-off queue, based on the percentage provided by the user.
- Randomly decide if a plane should join the landing priority queue, based on the percentage provided by the user.
For this assignment, you will create a Plane class. Any Plane object joining the landing priority queue should have a random amount of remaining fuel assigned to it. This should range from about 5 to 15 time-units of fuel. Planes with less fuel upon arrival have a higher priority than planes with more fuel. Note: Your Plane class needs to implement Comparable. When a Plane leaves the priority queue and begins to land, determine how long it had been in the queue. If it was in the queue for longer than the fuel it had upon arrival, it crashes. A crashed plane doesn't count as a landed plane.
Each plane has a "transaction time" (i.e. how long it takes for a plane to land or take off). When a plane object is created, give it a random transaction time between 1 and 3. While a plane is landing or taking off, the runway is considered in use, and no other plane is allowed on the runway. When the runway is done being used, the landing priority queue is checked. If it contains any planes, the next one is selected to land. If there are no planes in the landing queue, the next plane in the take off queue may do so.
Record interesting statistics from your simulation and print them to the console. For example:
- What is the average time spent waiting for take off?
- What is the longest time spent waiting for take off?
- What is the average time spent waiting to land?
- What is the longest time spent waiting to land?
- How many planes crashed?
- How many planes total took off and landed during the simulation?
If you do not like the results you are getting (i.e. some planes planes crashed, no planes were able to take off, etc) feel free to tweak your numbers until the results are more realistic.
For the landing priority queue, use the priority queue you created in part 1.
For the take off queue, you may use the ArrayQueue code developed in class.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

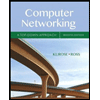
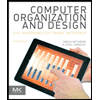
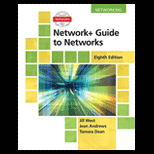
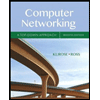
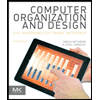
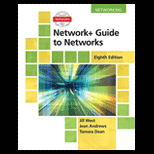
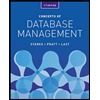
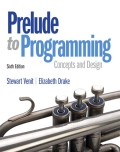
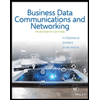