Add missing code to turn this class a singleton in Java package com.gamingroom; import java.util.ArrayList; import java.util.List; /** * A singleton service for the game engine */ public class GameService { /** * A list of the active games */ private static List
Add missing code to turn this class a singleton in Java package com.gamingroom; import java.util.ArrayList; import java.util.List; /** * A singleton service for the game engine */ public class GameService { /** * A list of the active games */ private static List
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Add missing code to turn this class a singleton in Java
package com.gamingroom;
import java.util.ArrayList;
import java.util.List;
import java.util.List;
/**
* A singleton service for the game engine */
public class GameService {
* A singleton service for the game engine */
public class GameService {
/**
* A list of the active games
*/
private static List<Game> games = new ArrayList<Game>();
* A list of the active games
*/
private static List<Game> games = new ArrayList<Game>();
/*
* Holds the next game identifier
*/
private static long nextGameId = 1;
* Holds the next game identifier
*/
private static long nextGameId = 1;
// FIXME: Add missing pieces to turn this class a singleton
/**
* Construct a new game instance
*
* @param name the unique name of the game
* @return the game instance (new or existing)
*/
public Game addGame(String name) {
// a local game instance
Game game = null;
Game game = null;
// FIXME: Use iterator to look for existing game with same name
// if found, simply return the existing instance
// if found, simply return the existing instance
// if not found, make a new game instance and add to list of games
if (game == null) {
game = new Game(nextGameId++, name);
games.add(game);
}
if (game == null) {
game = new Game(nextGameId++, name);
games.add(game);
}
// return the new/existing game instance to the caller
return game;
}
return game;
}
/**
* Returns the game instance at the specified index.
* <p>
* Scope is package/local for testing purposes.
* </p>
* @param index index position in the list to return
* @return requested game instance
*/
Game getGame(int index) {
return games.get(index);
}
/**
* Returns the game instance with the specified id.
*
* @param id unique identifier of game to search for
* @return requested game instance
*/
public Game getGame(long id) {
* Returns the game instance at the specified index.
* <p>
* Scope is package/local for testing purposes.
* </p>
* @param index index position in the list to return
* @return requested game instance
*/
Game getGame(int index) {
return games.get(index);
}
/**
* Returns the game instance with the specified id.
*
* @param id unique identifier of game to search for
* @return requested game instance
*/
public Game getGame(long id) {
// a local game instance
Game game = null;
Game game = null;
// FIXME: Use iterator to look for existing game with same id
// if found, simply assign that instance to the local variable
// if found, simply assign that instance to the local variable
return game;
}
}
/**
* Returns the game instance with the specified name.
*
* @param name unique name of game to search for
* @return requested game instance
*/
public Game getGame(String name) {
* Returns the game instance with the specified name.
*
* @param name unique name of game to search for
* @return requested game instance
*/
public Game getGame(String name) {
// a local game instance
Game game = null;
Game game = null;
// FIXME: Use iterator to look for existing game with same name
// if found, simply assign that instance to the local variable
// if found, simply assign that instance to the local variable
return game;
}
}
/**
* Returns the number of games currently active
*
* @return the number of games currently active
*/
public int getGameCount() {
return games.size();
}
}
* Returns the number of games currently active
*
* @return the number of games currently active
*/
public int getGameCount() {
return games.size();
}
}
Game.java
package com.gamingroom;
/**
* A simple class to hold information about a game
*
* <p>
* Notice the overloaded constructor that requires
* an id and name to be passed when creating.
* Also note that no mutators (setters) defined so
* these values cannot be changed once a game is
* created.
* </p>
*
*
*
*/
public class Game {
long id;
String name;
/**
* Hide the default constructor to prevent creating empty instances.
*/
private Game() {
}
* A simple class to hold information about a game
*
* <p>
* Notice the overloaded constructor that requires
* an id and name to be passed when creating.
* Also note that no mutators (setters) defined so
* these values cannot be changed once a game is
* created.
* </p>
*
*
*
*/
public class Game {
long id;
String name;
/**
* Hide the default constructor to prevent creating empty instances.
*/
private Game() {
}
/**
* Constructor with an identifier and name
*/
public Game(long id, String name) {
this();
this.id = id;
this.name = name;
}
* Constructor with an identifier and name
*/
public Game(long id, String name) {
this();
this.id = id;
this.name = name;
}
/**
* @return the id
*/
public long getId() {
return id;
}
* @return the id
*/
public long getId() {
return id;
}
/**
* @return the name
*/
public String getName() {
return name;
}
* @return the name
*/
public String getName() {
return name;
}
@Override
public String toString() {
return "Game [id=" + id + ", name=" + name + "]";
}
public String toString() {
return "Game [id=" + id + ", name=" + name + "]";
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
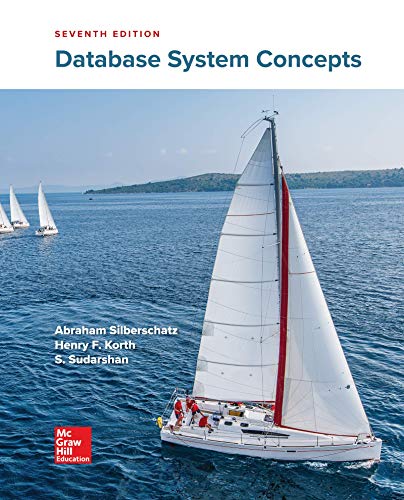
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
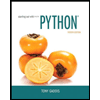
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
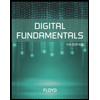
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
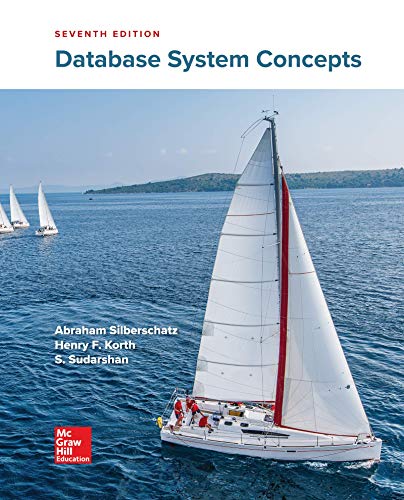
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
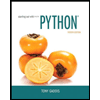
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
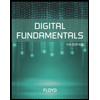
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
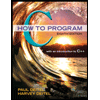
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
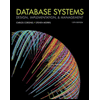
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
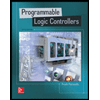
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education