• For this assignment, you will write a program to simulate enrolling a user-specified number of email addresses to a newsletter subscription • The user should enter the exact number of emails to be subscribed • Since this information will be stored as an int, the program should check for input mismatch exception Next, using the number input by the user, you should declare an array of Strings of the given length • Using a for loop, read in the specified number of emails as Strings • However, if the email address is not valid for one of the following reasons, the user should be prompted to enter the email again, until it is correct. You should check for the below errors in the address, in this exact order 1. The email does not contain an @ 2. The email has an invalid extension 3. The email contains a space, in the middle of the String • Additionally, if the email contains any leading or trailing whitespace, it should be removed - preferably before checking if there is a whitespace in the middle of the String You should check for errors in the email address, and remove leading and trailing whitespace, by calling 4 methods, defined in the starter code below
• For this assignment, you will write a program to simulate enrolling a user-specified number of email addresses to a newsletter subscription • The user should enter the exact number of emails to be subscribed • Since this information will be stored as an int, the program should check for input mismatch exception Next, using the number input by the user, you should declare an array of Strings of the given length • Using a for loop, read in the specified number of emails as Strings • However, if the email address is not valid for one of the following reasons, the user should be prompted to enter the email again, until it is correct. You should check for the below errors in the address, in this exact order 1. The email does not contain an @ 2. The email has an invalid extension 3. The email contains a space, in the middle of the String • Additionally, if the email contains any leading or trailing whitespace, it should be removed - preferably before checking if there is a whitespace in the middle of the String You should check for errors in the email address, and remove leading and trailing whitespace, by calling 4 methods, defined in the starter code below
Chapter12: Exception Handling
Section: Chapter Questions
Problem 9PE
Related questions
Question
(Intro to Java)
Avoid using Breaks!
/**
* @author
* @author
* CIS 36A, Assignment 17.1
*/
import java.util.Scanner;
public class Newsletter {
/**
* Removes all blank spaces in front of
* and at the end of a String
* Hint: use two while loops - one for
* the front of the String, and one
* for the end of the String.
* Use substring to remove the blank space
* @author
* @author
* CIS 36A, Assignment 17.1
*/
import java.util.Scanner;
public class Newsletter {
/**
* Removes all blank spaces in front of
* and at the end of a String
* Hint: use two while loops - one for
* the front of the String, and one
* for the end of the String.
* Use substring to remove the blank space
* from the String
* @param email the email address
* @return an email with no blank spaces
* at the front or end of the String
*/
public static String cutSpace(String email) {
* @param email the email address
* @return an email with no blank spaces
* at the front or end of the String
*/
public static String cutSpace(String email) {
//write one while loop here
//write the second while loop here
return email;
}
/**
* Searches a String for an @ character
* @param email the String to search
* @return whether the String contains an '@'
*/
public static boolean hasAt(String email) {
}
/**
* Searches a String for an @ character
* @param email the String to search
* @return whether the String contains an '@'
*/
public static boolean hasAt(String email) {
//write a for loop here to iterate through the String email
return false;
}
/**
* Uses a for loop to iterate through an array of
* correct email extensions, comparing the last 4
* characters in a String parameter to each correct
* extension. Hint: If the extension matches the
* last 4 chars in the parameter return true
* and return false only after checking all extensions
* @param email the String, whose last 4 chars is
* compared to each extension
* @return whether or not the last 4 chars match one
* of the extensions
*/
public static boolean correctExtension(String email) {
//source: https://en.wikipedia.org/wiki/List_of_Internet_top-level_domains
String[] extensions = {".com", ".net", ".org", ".edu", ".gov", ".int"};
}
/**
* Uses a for loop to iterate through an array of
* correct email extensions, comparing the last 4
* characters in a String parameter to each correct
* extension. Hint: If the extension matches the
* last 4 chars in the parameter return true
* and return false only after checking all extensions
* @param email the String, whose last 4 chars is
* compared to each extension
* @return whether or not the last 4 chars match one
* of the extensions
*/
public static boolean correctExtension(String email) {
//source: https://en.wikipedia.org/wiki/List_of_Internet_top-level_domains
String[] extensions = {".com", ".net", ".org", ".edu", ".gov", ".int"};
//write a for loop here to iterate through the array extensions
return false;
}
/**
* Determines whether a String contains a space
* @param email the String to search for a space
* @return whether the String contains a space
*/
public static boolean containsASpace(String email) {
}
/**
* Determines whether a String contains a space
* @param email the String to search for a space
* @return whether the String contains a space
*/
public static boolean containsASpace(String email) {
//Write a for loop here to iterate through the String email
return false;
}
public static void main(String[] args){
Scanner input = new Scanner(System.in);
int numEmails;
}
public static void main(String[] args){
Scanner input = new Scanner(System.in);
int numEmails;
String email;
System.out.println("Welcome to our newsletter subscription service!\n");
System.out.print("Enter the number of emails to subscribe: ");
System.out.print("Enter the number of emails to subscribe: ");
//check for input mismatch exception with a while loop
numEmails = input.nextInt();
//declare your array of Strings here
//write a for loop here with if-else if-else if- else
//In each if and else if statement, call one of your methods
System.out.println("\nYou entered the following emails:\n");
//print the array to the console with a for loop
input.close();
}
}
}
}
- Begin by writing each method given the detailed instructions in the comments for each method
- Then, write the main method by following the hints in the comments
- Run your code to verify that you get the same output given the same input, following my sample output below
- Once your program is working properly, submit it to Canvas
Sample Output #1:
Welcome to our newsletter subscription service!
Enter the number of emails to subscribe: six
Error! Please enter a number not text!
Enter the number of emails to subscribe: 6d
Error! Please enter a number not text!
Enter the number of emails to subscribe: 6
Enter email #0: marianne2gmail.com
Error! The email address must contain an @ symbol
Enter email #0: marianne@gmail.com
Enter email #1: mustafa@hotmail.co
Error! The email address must have a valid extension
Enter email #1: mustafa@hotmailcom
Error! The email address must have a valid extension
Enter email #1: mustafa@hotmail.com
Enter email #2: xi@comcast .net
Error! The email address cannot contain spaces
Enter email #2: xi@comcast.net
Enter email #3: kim sbcglobal .net
Error! The email address must contain an @ symbol
Enter email #3: kim@ sbcglobal .ne
Error! The email address must have a valid extension
Enter email #3: kim@ sbcglobal .net
Error! The email address cannot contain spaces
Enter email #3: kim@sbcglobal
Error! The email address must have a valid extension
Enter email #3: kim@sbcglobal.net
Enter email #4: leila@gmail.com
Enter email #5: lee@deanza.edu
You entered the following emails:
0. marianne@gmail.com
1. mustafa@hotmail.com
2. xi@comcast.net
3. kim@sbcglobal.net
4. leila@gmail.com
5. lee@deanza.edu
Enter the number of emails to subscribe: six
Error! Please enter a number not text!
Enter the number of emails to subscribe: 6d
Error! Please enter a number not text!
Enter the number of emails to subscribe: 6
Enter email #0: marianne2gmail.com
Error! The email address must contain an @ symbol
Enter email #0: marianne@gmail.com
Enter email #1: mustafa@hotmail.co
Error! The email address must have a valid extension
Enter email #1: mustafa@hotmailcom
Error! The email address must have a valid extension
Enter email #1: mustafa@hotmail.com
Enter email #2: xi@comcast .net
Error! The email address cannot contain spaces
Enter email #2: xi@comcast.net
Enter email #3: kim sbcglobal .net
Error! The email address must contain an @ symbol
Enter email #3: kim@ sbcglobal .ne
Error! The email address must have a valid extension
Enter email #3: kim@ sbcglobal .net
Error! The email address cannot contain spaces
Enter email #3: kim@sbcglobal
Error! The email address must have a valid extension
Enter email #3: kim@sbcglobal.net
Enter email #4: leila@gmail.com
Enter email #5: lee@deanza.edu
You entered the following emails:
0. marianne@gmail.com
1. mustafa@hotmail.com
2. xi@comcast.net
3. kim@sbcglobal.net
4. leila@gmail.com
5. lee@deanza.edu

Transcribed Image Text:• For this assignment, you will write a program to simulate enrolling a user-specified number of email addresses to a newsletter subscription
• The user should enter the exact number of emails to be subscribed
• Since this information will be stored as an int, the program should check for input mismatch exception
• Next, using the number input by the user, you should declare an array of Strings of the given length
Using a for loop, read in the specified number of emails as Strings
o However, if the email address is not valid for one of the following reasons, the user should be prompted to enter the email again, until
it is correct. You should check for the below errors in the address, in this exact order
1. The email does not contain an @
2. The email has an invalid extension
3. The email contains a space, in the middle of the String
• Additionally, if the email contains any leading or trailing whitespace, it should be removed - preferably before checking if there is a
whitespace in the middle of the String
• You should check for errors in the email address, and remove leading and trailing whitespace, by calling 4 methods, defined in the starter
code below
• Once the email address is correct, save it inside the array of Strings
• After all email addresses have been saved in the String array, print out this array of Strings and exit the program.
• Important Note: the only String methods you are allowed to use in this program are .equals(), .length(), .substring() and .charAt(). If you use
any other String methods, you will receive a 0 for this assignment.
Begin by copying and pasting the below starter code into a Java file named NewsLetter:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
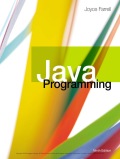
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
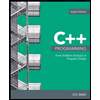
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
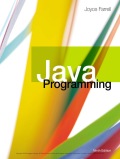
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
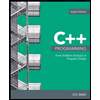
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning