run of the do while loop it gives me the error message at the first instance of inStack.peek() method the comments explain what the code should do.please explain to me what the problem is in words not in code. queue is hidden due to character constraints. this is in java code. here is the code: MAIN FUNCTION import java.util.Scanner; import java.util.Random; public class test { public static void main(String[] Args) { Scanner input = new Scanner(System.in); Random rand = new Random(); item maximum = new item(0, 0); stackX inStack; stackX outStack;
in my code I am experiencing a problem with the inStack.peek() method. it is giving me an out of bounds exception "java.lang.ArrayIndexOutOfBoundsException: Index -1 out of bounds for length 10" I have no idea why this is happening but i believe the problem is in the first for loop in the do while in the code. When the code goes through its second run of the do while loop it gives me the error message at the first instance of inStack.peek() method the comments explain what the code should do.please explain to me what the problem is in words not in code. queue is hidden due to character constraints. this is in java code.
here is the code:
MAIN FUNCTION
import java.util.Scanner;
import java.util.Random;
public class test
{
public static void main(String[] Args)
{
Scanner input = new Scanner(System.in);
Random rand = new Random();
item maximum = new item(0, 0);
stackX inStack;
stackX outStack;
queue discardQueue;
item itemObj;
System.out.println("please enter the size of the array");
int maxSize = input.nextInt();
while(maxSize < 1 || maxSize > 20)
{
System.out.println("number must be a positive number between 1 and 20");
maxSize = input.nextInt();
}
inStack = new stackX(maxSize);
outStack = new stackX(maxSize);
discardQueue = new queue(maxSize);
//initialize the inStack
for(int i = 0; i < maxSize; i++)
{
itemObj = new item(1 + i, 1 + rand.nextInt(10));
inStack.push(itemObj);
}
//display the stack for debug purposes. look for discrepancies in timestamp
inStack.displayStack();
//to compare items with
item compareItem;
// do this loop untill the outstack is full
do
{
// this for loop finds the maximum inside the stack and then inserts it into a queue
for(int i = 0; i < maxSize; i++)
{
//compare item is now references the inStack.peek() item
compareItem = inStack.peek();
//if the compareItem's key is greater than the maximum object's key then make maximum equal to compare item
if(compareItem.GetKey() > maximum.GetKey())
{
maximum = compareItem;
}
//if queue is not full insert the top value of the instack
if(!(discardQueue.isFull()))
discardQueue.insert(inStack.peek());
//remove an element from instack
inStack.pop();
}
//display maximum for debug
System.out.println("maximum is " + maximum.GetKey());
//display the size of the queue for debug purposes
System.out.println("size of queue" + discardQueue.size());
//display the queue in its entirety for debug purposes. check for anomolies in items
discardQueue.displayQueue();
// this for loop uses the maximum value as a searchkey and as the queue is discarding its
//contents it checks if the maximum is equal to the front of the discard queue.
// if the maximum is not equal it discards the queue's element and pushes it back into
// the inStack. if a maximum value is found it discards it and pushes it into the outStack.
for(int i = 0; i < maxSize; i++)
{
//make it possible to compare the front of discardQueue by initializing compareItem
compareItem = discardQueue.peekFront();
//if the key of the front of the queue is equal to the maximum's key then
if( compareItem.GetKey() == maximum.GetKey())
{
//push into outStack
if(!outStack.isFull() && !discardQueue.isEmpty())
{
outStack.push(discardQueue.remove());
}
}
//if the key of the front is not equal to the maximum then
if(compareItem.GetKey() != maximum.GetKey())
{
//push into instack
if(!inStack.isFull() && !discardQueue.isEmpty())
{
inStack.push(discardQueue.remove());
}
}
}
System.out.println("\n inStack after queue.");
inStack.displayStack();
maximum = new item(0,0);
} while(!outStack.isFull());
System.out.println("\n displaying inStack");
System.out.println("\n displaying outStack");
outStack.displayStack();
}
}
ITEM CLASS
public class item
{
private int key;
private int timeStamp;
public item(int newtimeStamp, int rand)
{
key = rand; //instantiates the key with parameter
timeStamp = newtimeStamp; //instantiates the timestamp with paramater
}
//this method returns the key of the object
public int GetKey()
{
return key;
}
//this method returns the timestamp for debug purposes
public int GetTimeStamp()
{
return timeStamp;
}
//this method displays the object's contents
public void ToString()
{
System.out.println(key + " : " + timeStamp);
}
}
STACKX CLASS
public class stackX
{
// instance variables - replace the example below with your own
private int top;
private item[] stackArray;
private int maxSize;
private int size;
public stackX(int s)
{
maxSize = s;
stackArray = new item[maxSize];
top = -1; // top is empty by default
}
// this method adds an item to the stack
public void push(item j)
{
stackArray[++top] = j;
size++;
}
// this method removes an item from the stack
public item pop()
{
return stackArray[top--];
}
// this method returns the current top of the stack
public item peek()
{
return stackArray[top];
}
public boolean isEmpty()
{
return (top == -1);
}
public boolean isFull()
{
return (top == maxSize - 1);
}
// displays the entire stack for debug purposes.
public void displayStack()
{
for(int i = 0; i < size; i++)
{
stackArray[i].ToString();
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

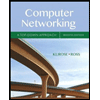
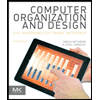
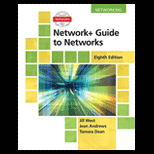
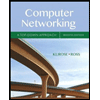
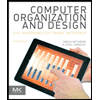
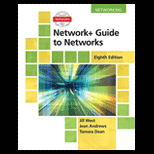
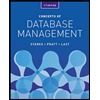
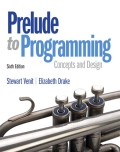
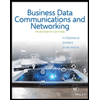