For this problem, you can use all classes in the java.util package. As a genius IT/SE student, you are asked by the Ministry of Health to help them analyze the Covid sequences. In particular, given 2 Covid variants, your task is to find the longest common subsequence between them. Each Covid variant has 2 properties: code (String) and sequence (String). Both properties contain only upper case letters 'A' to 'Z'. The length of the sequence is up to 100 characters. Subsequence definition: given a string S consists of the letters s1, s2, remaining string S2 is called a subsequence of S. sn in that order. If you delete zero, one, or more letters from S without changing the letters' order, the For example: given the string "HELLO", the following strings are some of subsequences: "HELLO", "HLO", "O", "HO", "' (empty string). The following strings are not its subsequences: "OL", "HELLOO", "LEH". Create a type CovidVariant that contains 2 properties: code (String), and sequence (String). Implement the following 3 public methods (in addition to the constructor, of course) on the CovidVariant type: • boolean evenSequence(): Test whether the current object's sequence has an even length or not. Return true if the sequence's length is an even number, return false otherwise. • boolean isSubsequence(String s): Test whether the given String parameter is a subsequence of the current object's sequence property. Return true if the given parameter is a subsequence, return false otherwise. • int longestCommonSubsequence(CovidVariant other): Return the length of the longest common subsequence between the current object's sequence and the parameter object's sequence.
For this problem, you can use all classes in the java.util package. As a genius IT/SE student, you are asked by the Ministry of Health to help them analyze the Covid sequences. In particular, given 2 Covid variants, your task is to find the longest common subsequence between them. Each Covid variant has 2 properties: code (String) and sequence (String). Both properties contain only upper case letters 'A' to 'Z'. The length of the sequence is up to 100 characters. Subsequence definition: given a string S consists of the letters s1, s2, remaining string S2 is called a subsequence of S. sn in that order. If you delete zero, one, or more letters from S without changing the letters' order, the For example: given the string "HELLO", the following strings are some of subsequences: "HELLO", "HLO", "O", "HO", "' (empty string). The following strings are not its subsequences: "OL", "HELLOO", "LEH". Create a type CovidVariant that contains 2 properties: code (String), and sequence (String). Implement the following 3 public methods (in addition to the constructor, of course) on the CovidVariant type: • boolean evenSequence(): Test whether the current object's sequence has an even length or not. Return true if the sequence's length is an even number, return false otherwise. • boolean isSubsequence(String s): Test whether the given String parameter is a subsequence of the current object's sequence property. Return true if the given parameter is a subsequence, return false otherwise. • int longestCommonSubsequence(CovidVariant other): Return the length of the longest common subsequence between the current object's sequence and the parameter object's sequence.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
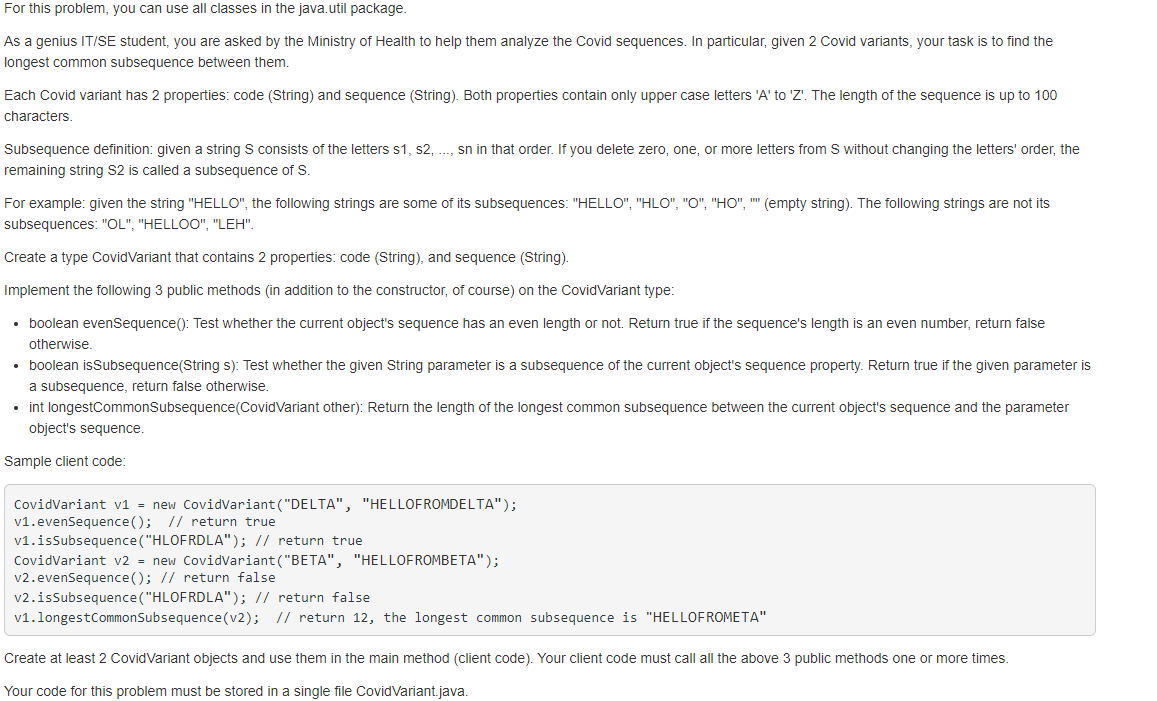
Transcribed Image Text:For this problem, you can use all classes in the java.util package.
As a genius IT/SE student, you are asked by the Ministry of Health to help them analyze the Covid sequences. In particular, given 2 Covid variants, your task is to find the
longest common subsequence between them.
Each Covid variant has 2 properties: code (String) and sequence (String). Both properties contain only upper case letters 'A' to 'Z'. The length of the sequence is up to 100
characters.
Subsequence definition: given a string S consists of the letters s1, s2,
sn in that order. If you delete zero, one, or more letters from S without changing the letters' order, the
remaining string S2 is called a subsequence of S.
For example: given the string "HELLO", the following strings are some of its subsequences: "HELLO", "HLO", "O", "HO", "'" (empty string). The following strings are not its
subsequences: "OL", "HELLOO", "LEH".
Create a type CovidVariant that contains 2 properties: code (String), and sequence (String).
Implement the following 3 public methods (in addition to the constructor, of course) on the CovidVariant type:
• boolean evenSequence(): Test whether the current object's sequence has an even length or not. Return true if the sequence's length is
even number, return false
otherwise.
• boolean isSubsequence(String s): Test whether the given String parameter is a subsequence of the current object's sequence property. Return true if the given parameter is
a subsequence, return false otherwise.
• int longestCommonSubsequence(CovidVariant other): Return the length of the longest common subsequence between the current object's sequence and the parameter
object's sequence.
Sample client code:
CovidVariant v1 = new CovidVariant ("DELTA", "HELLOFROMDELTA");
v1.evenSequence(); // return true
v1.isSubsequence ("HLOFRDLA"); // return true
CovidVariant v2 = new CovidVariant ("BETA", "HELLOFROMBETA");
v2.evenSequence(); // return false
v2.issubsequence ("HLOFRDLA"); // return false
v1.longestCommonSubsequence(v2); // return 12, the longest common subsequence is "HELLOFROMETA"
Create at least 2 CovidVariant objects and use them in the main method (client code). Your client code must call all the above 3 public methods one or more times.
Your code for this problem must be stored in a single file CovidVariant.java.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
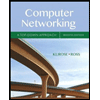
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
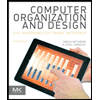
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
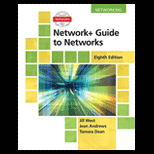
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
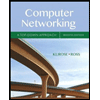
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
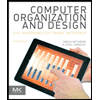
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
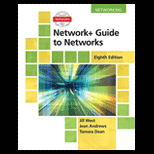
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
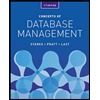
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
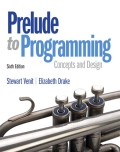
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
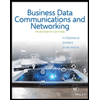
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY