I need to write a program which allows me to input "3 Joe,123-5432 Linda,983-4123 Frank,867-5309 Frank" and output "867-5309". This is the code I have so far..." import java.util.Hashtable; import java.util.Scanner; public class Main { public static void main(String[] args) { // initialize Scanner class Scanner sc = new Scanner(System.in); // initialize Hashtable // tells java that both key and value will be of type String Hashtable my_dict = new Hashtable<>(); // get the count of records to be entered // 1st part of the input int n = sc.nextInt(); // execute for loop n times // 2nd part of the input for (int i = 0; i < n; i++) { // get a record from the user. example: Linda,983-4123 String s = sc.next(); // split the string on comma to get the name and code in an array String[] details = s.split(","); // get the name String name = details[0]; // get the code String code = details[1]; // put the record in the dictionary // here name is key and code is value corresponding to it my_dict.put(name, code); } // get the name // 3rd part of the input String name = sc.next(); // get the code corresponding to name in the dictionary String code = my_dict.get(name); // print the code System.out.println(code); } }" I try to run it, but I keep getting " LabProgram.java:4: error: class Main is public, should be declared in a file named Main.java public class Main { ^ 1 error"
I need to write a program which allows me to input "3 Joe,123-5432 Linda,983-4123 Frank,867-5309 Frank" and output "867-5309". This is the code I have so far..."
import java.util.Hashtable;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// initialize Scanner class
Scanner sc = new Scanner(System.in);
// initialize Hashtable
// <String, String> tells java that both key and value will be of type String
Hashtable<String, String> my_dict = new Hashtable<>();
// get the count of records to be entered
// 1st part of the input
int n = sc.nextInt();
// execute for loop n times
// 2nd part of the input
for (int i = 0; i < n; i++) {
// get a record from the user. example: Linda,983-4123
String s = sc.next();
// split the string on comma to get the name and code in an array
String[] details = s.split(",");
// get the name
String name = details[0];
// get the code
String code = details[1];
// put the record in the dictionary
// here name is key and code is value corresponding to it
my_dict.put(name, code);
}
// get the name
// 3rd part of the input
String name = sc.next();
// get the code corresponding to name in the dictionary
String code = my_dict.get(name);
// print the code
System.out.println(code);
}
}"
I try to run it, but I keep getting "
![ESC myFSC X
Bb LEARNI X
Вы Мodule х
Ь ESC MY X
* holodec x
zy Section X
G Chegg
G Chegg
C 11.18 L X
G Chegg x
G Chegg X
C 11.18 L X
+
i learn.zybooks.com/zybook/FARMINGDALECSC111MoorningFall2021/chapter/6/section/39?content_resource_id=49390879
6.39 LAB: Contact list
A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address,
birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs
consist of a name and a phone number (both strings), separated by a comma. That list is followed by a name, and your program should
output the phone number associated with that name. Assume that the list will always contain less than 20 word pairs.
Ex: If the input is:
3 Joe,123-5432 Linda, 983-4123 Frank,867-5309 Frank
the output is:
867-5309
Your program must define and call the following method. The return value of getPhoneNumber() is the phone number associated with the
specific contact name.
public static String getPhoneNumber(String[] nameArr, String[] phoneNumberArr, String contactName, int
arraySize)
Hint: Use two arrays: One for the string names, and the other for the string phone numbers.
338758.2149958.qx3zqy7
LAB
6.39.1: LAB: Contact list
0/10
АCTIVITY
2:58 PM
O Type here to search
(1
10/22/2021
..](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7daa69d8-205a-4b72-abf5-96966d755823%2F9676393a-8a3b-4e9b-a1dc-56c7fcd9a534%2F5u5o1v_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

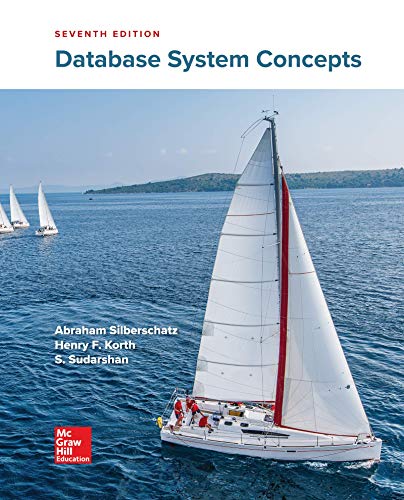
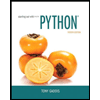
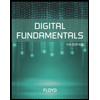
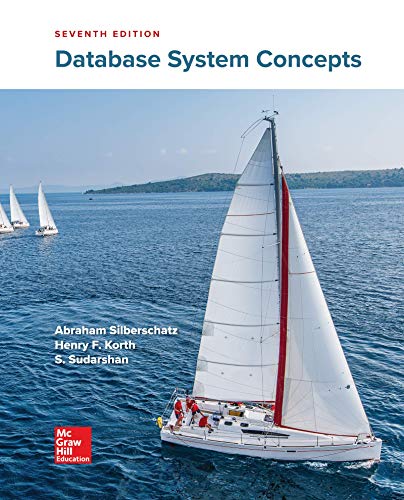
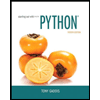
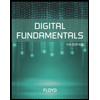
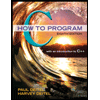
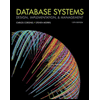
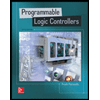