Function Implementation with Data Abstraction in C Programming 1. Create a new file and SAVE as MATHV1.H Define and Implement the following functions: int sum(int x, int y); - returns the computed sum of two numbers x and y int diff(int x, int y); - returns the computed difference of two numbers x and y. Make sure that the larger number is deducted by the smaller number to avoid a negative answer long int prod(int x, int y); - returns the computed product of two number x and y float quot(int x, int y); - returns the computed quotient of two numbers x and y. Make sure that the larger number is always the numerator and divided by the smaller number to avoid division by zero error. int mod(int x, int y); - returns the computed remainder of two numbers x and y using the modulo operator % 2. Create another new file and save as MYTOOLS.H void center(int row, char str[]); /*centers text across the screen -calculate here for the center col using the formula col=(80-strlen(str))/2; -then use gotoxy(col,row) to print the value in string str*/ void outString(int col,int row,char str[]); /*outputs the string str at the specified col and row numbers.*/ void drawSBox(int x1,int y1, int x2, int y2); /* draws a single-line box */ void drawDBox(int x1,int y1, int x2, int y2); /* draws a double-line box */ note: you may place here the definition of your function void menu(); /* to display this menu layout: PROGRAMMING 2 FUNCTION IMPLEMENTATION w/ DATA ABSTARACTION ===================================== ~~~~~ BASIC MATH OPERATIONS ~~~~~ MODULO [%] DIVIDE [ / ] MULTIPLY [ * ] ADD [ +] SUBTRACT [ - ] Enter math operator[%/*+-]: ======================================= 3. Define the above functions declared in both MATHV1.H and MYTOOLS.H. Save them as MATHV1.C and MYTOOLS.C respectively. 4. Create another new file and save it as FUNCTIONS.C Include the files "MYTOOLS.H" and "MATHV1.H" Requirements/Specifications: -write a function main() to use all functions defined in MATHV1.H and MYTOOLS.H -invoke the menu layout above w/ double-line box -prompt the user to input and select a math operator -the inputted math operator that are valid [% or / or * or + or - only]. If it's invalid, prompt the user a message that it's invalid and ask him /her to input again -If the math operator entered by the user is valid, draw a small double-line box and prompt the user to input two numbers, like this: _____________________________ Enter 1st number: Enter 2nd number: Result is : _____________________________ Press 1 to continue or 0 to exit... - Repeat the entire process while user res
Function Implementation with Data Abstraction in C
1. Create a new file and SAVE as MATHV1.H
Define and Implement the following functions:
int sum(int x, int y);
- returns the computed sum of two numbers x and y
int diff(int x, int y);
- returns the computed difference of two numbers x and y.
Make sure that the larger number is deducted
by the smaller number to avoid a negative answer
long int prod(int x, int y);
- returns the computed product of two number x and y
float quot(int x, int y);
- returns the computed quotient of two numbers x and y.
Make sure that the larger number is always the numerator
and divided by the smaller number to avoid division
by zero error.
int mod(int x, int y);
- returns the computed remainder of two numbers x and y using
the modulo operator %
2. Create another new file and save as MYTOOLS.H
void center(int row, char str[]);
/*centers text across the screen
-calculate here for the center col using
the formula col=(80-strlen(str))/2;
-then use gotoxy(col,row) to print the value in string
str*/
void outString(int col,int row,char str[]);
/*outputs the string str at the specified col and row numbers.*/
void drawSBox(int x1,int y1, int x2, int y2);
/* draws a single-line box */
void drawDBox(int x1,int y1, int x2, int y2);
/* draws a double-line box */
note: you may place here the definition of your function
void menu();
/* to display this menu layout:
PROGRAMMING 2
FUNCTION IMPLEMENTATION
w/ DATA ABSTARACTION
=====================================
~~~~~ BASIC MATH OPERATIONS ~~~~~
MODULO [%]
DIVIDE [ / ]
MULTIPLY [ * ]
ADD [ +]
SUBTRACT [ - ]
Enter math operator[%/*+-]:
=======================================
3. Define the above functions declared in both MATHV1.H and MYTOOLS.H. Save them as
MATHV1.C and MYTOOLS.C respectively.
4. Create another new file and save it as FUNCTIONS.C
Include the files "MYTOOLS.H" and "MATHV1.H"
Requirements/Specifications:
-write a function main() to use all functions defined in MATHV1.H and MYTOOLS.H
-invoke the menu layout above w/ double-line box
-prompt the user to input and select a math operator
-the inputted math operator that are valid [% or / or * or + or - only]. If it's invalid, prompt
the user a message that it's invalid and ask him /her to input again
-If the math operator entered by the user is valid, draw a small double-line box and
prompt the user to input two numbers, like this:
_____________________________
Enter 1st number:
Enter 2nd number:
Result is :
_____________________________
Press 1 to continue or 0 to exit...
- Repeat the entire process while user response is
1 (for continue), program exits when user
replies 0 (means to exit)
![Description
1. Create a new file and SAVE as MATHV1.H
Define and Implement the following functions:
int sum(int x, int y);
- returns the computed sum of two numbers x and y
int diff(int x, int y);
- returns the computed difference of two numbers x and y.
Make sure that the larger number is deducted
by the smaller number to avoid a negative answer
long int prod(int x, int y);
- returns the computed product of two number x and y
float quot(int x, int y);
- returns the computed quotient of two numbers x and y.
Make sure that the larger number is always the numerator
and divided by the smaller number to avoid division
by zero error.
int mod(int x, int y);
- returns the computed remainder of two numbers x and y using
the modulo operator %
2. Create another new file and save as MYTOOLS.H
void center(int row, char str[]);
/*centers text across the screen
-calculate here for the center col using
the formula col=(80-strlen(str))/2;
-then use gotoxy(col, row) to print the value in string
str*/
void outString(int col,int row,char str[]);
/*outputs the string str at the specified col and row numbers.*/
void drawSBox(int x1, int y1, int x2, int y2);
/* draws a single-line box */
void drawDBox(int x1, int y1, int x2, int y2);
/* draws a double-line box */
note: you may place here the definition of your function
void menu();](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F10f77bcd-ed4c-456f-a8ab-f19df4ccdbf2%2Fd000fbbd-3650-4071-81e7-408fff792f9a%2F242zmwk_processed.jpeg&w=3840&q=75)
![/* to display this menu layout:
PROGRAMMING 2
FUNCTION IMPLEMENTATION
w/ DATA ABSTARACTION
BASIC MATH OPERATIONS ~~~~~
MODULO
DIVIDE
MULTIPLY
ADD
SUBTRACT
Enter math operator[%/*+-]:
[%]
[/ ]
[*]
[+]
[-]
3. Define the above functions declared in both MATHV1.H and MYTOOLS.H. Save them as
MATHV1.C and MYTOOLS.C respectively.
4. Create another new file and save it as FUNCTIONS.C
Include the files "MYTOOLS.H" and "MATHV1.H"
Requirements/Specifications:
-write a function main() to use all functions defined in MATHV1.H and MYTOOLS.H
-invoke the menu layout above w/ double-line box
-prompt the user to input and select a math operator
-the inputted math operator that are valid [% or / or* or + or - only]. If it's invalid, prompt
the user a message that it's invalid and ask him/her to input again
-If the math operator entered by the user is valid, draw a small double-line box and
prompt the user to input two numbers, like this:
Enter 1st number:
Enter 2nd number:
Result is:
Press 1 to continue or 0 to exit...
- Repeat the entire process while user response is
1 (for continue), program exits when user
replies 0 (means to exit)
Sample Output if Applicable
CNY Z:\CCS122-1L13FBC-1\EXER11.EXE
PROGRAMMING 2
FUNCTION IMPLEMENTATION
W/ DATA ABSTRACION
BASIC MATH OPERATIONS
Modulo [%]
Divide
[/]
Multiply [*]
ndd
[+]
Subtract [-]
Enter math operator: [ % / * * - ]:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F10f77bcd-ed4c-456f-a8ab-f19df4ccdbf2%2Fd000fbbd-3650-4071-81e7-408fff792f9a%2Fjkfpedk_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

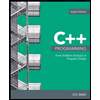
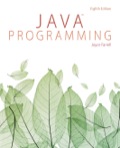
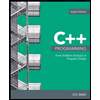
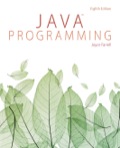