Given the formula for converting Fahrenheit (F) to Celcius (C): C = 5/9 x (F - 32), where C is the unit of temperature in Celcius and F is the unit of temperature in Fahrenheit. Write a complete C++ program that reads in a list of data F from a text file, then calculates the values of C using the formula given. The program should use an array to store the values of F as example shown in Figure 4. 13.29 29.76 14.81 23.78 29.37 .. Figure 4: Example of data F in the input file The program then prints a summary output onto the screen and the detail output into a text file as shown in Figures 5 and 6. Grades ‘H’ mean high temperature; ‘M’ is medium temperature and ‘L’ is low temperature. Your program must define several functions at least as listed in Table 3. You are also required to apply the concept of parameter passing to these functions. Average of the temperature: 32.3 Number of high temperature: 2 Number of medium temperature: 20 Number of low temperature: 8 Figure 5: Example of output on the screen C(Celcius) F (Farenheit) Description ========== ========= ===== 54.94 130.89 H 19.86 67.75 L 93.70 200.67 H 13.77 56.78 L : : : : : : Figure 6: Example of content in the output file Function Description readFile This function reads in a list of numbers from a text file and stores them into a onedimensional array. It receives the following parameters: a. The name of the text file to be read from b. An array to store the list of numbers read c. A variable to store the number of data read computeC This function computes the values of C. It receives the following parameters: a. An array that contains data F b. An array to store the calculated values of C c. The number of data average This function computes the average of a list of numbers stored in an array. grade This function determines either temperature (C) is high or medium or low. This function will return ‘H’ if C ≥ 35 , ‘M’ if C < 35 and C ≥ 20, and ‘L’ if C < 20. writeFile This function prints the output file as in Figure 7. It receives the following parameters: a. An array that contains data F b. An array that contains data C c. The number of data For printing summary output onto the screen, you may define another function or you may just put the code into the main function.
Given the formula for converting Fahrenheit (F) to Celcius (C):
C = 5/9 x (F - 32),
where C is the unit of temperature in Celcius and F is the unit of temperature in Fahrenheit. Write a complete C++
13.29 29.76 14.81 23.78 29.37 .. |
Figure 4: Example of data F in the input file
The program then prints a summary output onto the screen and the detail output into a text
file as shown in Figures 5 and 6. Grades ‘H’ mean high temperature; ‘M’ is medium temperature and
‘L’ is low temperature.
Your program must define several functions at least as listed in Table 3. You are also required to apply
the concept of parameter passing to these functions.
Average of the temperature: 32.3 Number of high temperature: 2 Number of medium temperature: 20 Number of low temperature: 8 |
Figure 5: Example of output on the screen
C(Celcius) F (Farenheit) Description ========== ========= ===== 54.94 130.89 H 19.86 67.75 L 93.70 200.67 H 13.77 56.78 L : : : : : : |
Figure 6: Example of content in the output file
Function | Description |
readFile | This function reads in a list of numbers from a text file and stores them into a onedimensional array. It receives the following parameters: a. The name of the text file to be read from b. An array to store the list of numbers read c. A variable to store the number of data read |
computeC | This function computes the values of C. It receives the following parameters: a. An array that contains data F b. An array to store the calculated values of C c. The number of data |
average | This function computes the average of a list of numbers stored in an array. |
grade | This function determines either temperature (C) is high or medium or low. This function will return ‘H’ if C ≥ 35 , ‘M’ if C < 35 and C ≥ 20, and ‘L’ if C < 20. |
writeFile | This function prints the output file as in Figure 7. It receives the following parameters: a. An array that contains data F b. An array that contains data C c. The number of data |
For printing summary output onto the screen, you may define another function or you may just put the code into the main function.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

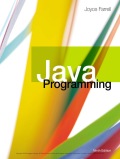
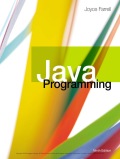