In this question, you are going to write a program that computes matrix operations using NumPy. The program reads values of matrices A, B, and C stored in a file called inputs.txt. This file should be placed under current directory where you have the program. The first line before each matrix contains the number of rows and the number of columns as shown below. 44 55 55 55 56 66 66 66 67 77 77 77 78 88 88 88 89 44 1234 2222 3333 4444 44 11 12 13 14 22 24 24 25 33 34 35 36 44 45 46 47 As a first step, the program reads data for matrices A, B and C from inputs.txt file and write them into console. A fourth matrix D is generated randomly. The program will calculate S = (A+B) * Transpose(C) + D) - A and find the maximum element in s. Complete the code given above so that it will produce an output as follows: Reading data from inputs.txt file in current directory **** Matrix A **** 55 55 55 56 66 20 12 67 77 15 25 78 88 12 13 89
In this question, you are going to write a program that computes matrix operations using NumPy. The program reads values of matrices A, B, and C stored in a file called inputs.txt. This file should be placed under current directory where you have the program. The first line before each matrix contains the number of rows and the number of columns as shown below. 44 55 55 55 56 66 66 66 67 77 77 77 78 88 88 88 89 44 1234 2222 3333 4444 44 11 12 13 14 22 24 24 25 33 34 35 36 44 45 46 47 As a first step, the program reads data for matrices A, B and C from inputs.txt file and write them into console. A fourth matrix D is generated randomly. The program will calculate S = (A+B) * Transpose(C) + D) - A and find the maximum element in s. Complete the code given above so that it will produce an output as follows: Reading data from inputs.txt file in current directory **** Matrix A **** 55 55 55 56 66 20 12 67 77 15 25 78 88 12 13 89
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
BY USING PYTHON
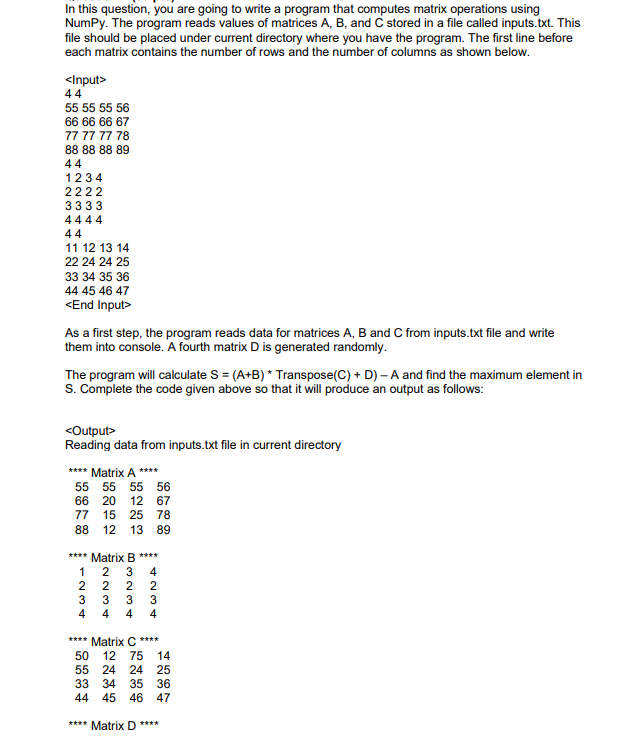
Transcribed Image Text:In this question, you are going to write a program that computes matrix operations using
NumPy. The program reads values of matrices A, B, and C stored in a file called inputs.txt. This
file should be placed under current directory where you have the program. The first line before
each matrix contains the number of rows and the number of columns as shown below.
<Input>
44
55 55 55 56
66 66 66 67
77 77 77 78
88 88 88 89
44
1234
2222
3333
4444
44
11 12 13 14
22 24 24 25
33 34 35 36
44 45 46 47
<End Input>
As a first step, the program reads data for matrices A, B and C from inputs.txt file and write
them into console. A fourth matrix D is generated randomly.
The program will calculate S = (A+B) * Transpose(C) + D) -A and find the maximum element in
s. Complete the code given above so that it will produce an output as follows:
<Output>
Reading data from inputs.txt file in current directory
**** Matrix A ****
55 55 55
56
66 20 12
67
77 15 25
78
88 12 13 89
**** Matrix B ****
2
3
4
2
2
3
3
3
3
4
4
4
**** Matrix C
****
50
55 24
12 75
14
24
25
33
34
35
36
44 45 46 47
**** Matrix D ****
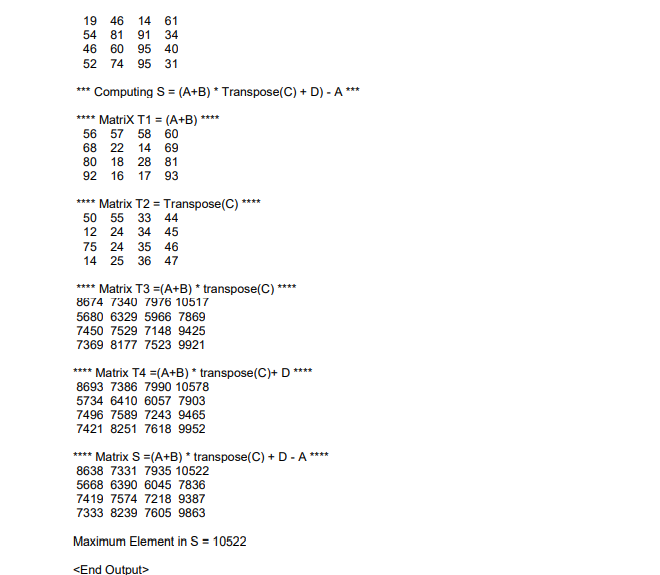
Transcribed Image Text:19 46
54 81
61
91 34
14
46 60
52 74 95
95
40
31
*** Computing S = (A+B) * Transpose(C) + D) - A ***
**** MatriX T1 = (A+B) ****
56 57 58 60
68 22 14
69
80 18 28
81
17 93
92 16
**** Matrix T2 = Transpose(C)
****
50 55 33 44
12
24
34 45
75 24
35 46
14 25 36 47
**** Matrix T3 =(A+B) * transpose(C) ****
8674 7340 7976 10517
5680 6329 5966 7869
7450 7529 7148 9425
7369 8177 7523 9921
**** Matrix T4 =(A+B) * transpose(C)+ D ***
8693 7386 7990 10578
5734 6410 6057 7903
7496 7589 7243 9465
7421 8251 7618 9952
**** Matrix S =(A+B) * transpose(C) + D -A**
***
8638 7331 7935 10522
5668 6390 6045 7836
7419 7574 7218 9387
7333 8239 7605 9863
Maximum Element in S= 10522
<End Output>
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

Recommended textbooks for you
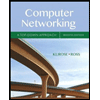
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
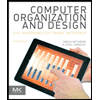
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
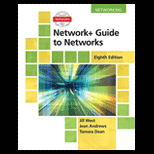
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
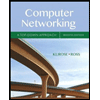
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
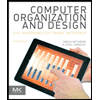
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
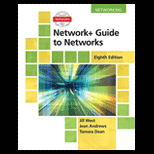
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
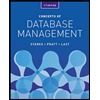
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
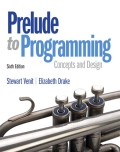
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
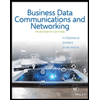
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY