Write a program that opens a file for read, reads the data in from the file and calculates the sum of the row values. The data is all integers with 7 values per line, and 10 lines of data in the file. The program must use two functions, one to open the file, and another to output and calculate the sum of the row values. All data must be read into a two dimensional array first, then the data is output and the sum calculated. The data should be printed out by row, along with the sum of the row values. Prototypes void rowSumCalculator( int myArray[][10], int rowCount, int colCount ); Function rowSumCalculator( ) writes out the data in the array and the sum of the values in the row. void openFile( ifstream& infile, string prompt ); Function openFile should print the prompt out to the user, read in the filename and open the file. If the file does not open, an error is written to the display, and the user prompted again. The function does not return until a file have been opened. Example Output Enter file to process: wksht19_data.txt 1 2 3 4 5 6 7 8 9 10 = 55 10 11 12 13 14 15 16 17 18 19 = 145 10 10 10 10 10 10 10 10 10 10 = 100 0 0 0 0 0 0 0 0 0 0 = 0 23 14 25 22 23 11 33 33 33 12 = 229 2 14 2 22 3 11 3 33 3 12 = 105 3 4 5 22 3 1 3 3 3 12 = 59 ./a.out Enter file to process: data.txt Error-"data.txt" did not open Enter file to process:
Write a program that opens a file for read, reads the data in from the file and calculates the sum of the row values. The data is all integers with 7 values per line, and 10 lines of data in the file. The program must use two functions, one to open the file, and another to output and calculate the sum of the row values. All data must be read into a two dimensional array first, then the data is output and the sum calculated. The data should be printed out by row, along with the sum of the row values. Prototypes
void rowSumCalculator( int myArray[][10], int rowCount, int colCount );
Function rowSumCalculator( ) writes out the data in the array and the sum of the values in the row.
void openFile( ifstream& infile, string prompt );
Function openFile should print the prompt out to the user, read in the filename and open the file. If the file does not open, an error is written to the display, and the user prompted again. The function does not return until a file have been opened.
Example Output
Enter file to process: wksht19_data.txt
1 2 3 4 5 6 7 8 9 10 = 55
10 11 12 13 14 15 16 17 18 19 = 145
10 10 10 10 10 10 10 10 10 10 = 100
0 0 0 0 0 0 0 0 0 0 = 0
23 14 25 22 23 11 33 33 33 12 = 229
2 14 2 22 3 11 3 33 3 12 = 105
3 4 5 22 3 1 3 3 3 12 = 59
./a.out
Enter file to process: data.txt
Error-"data.txt" did not open
Enter file to process:

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

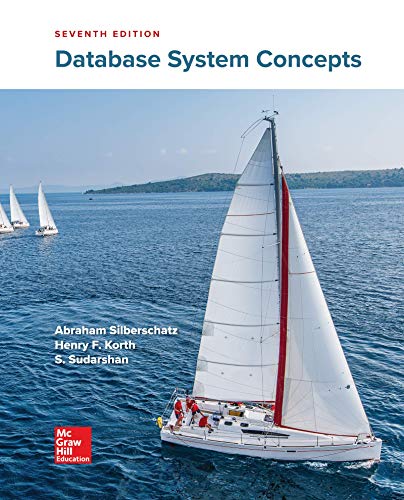
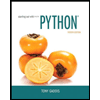
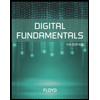
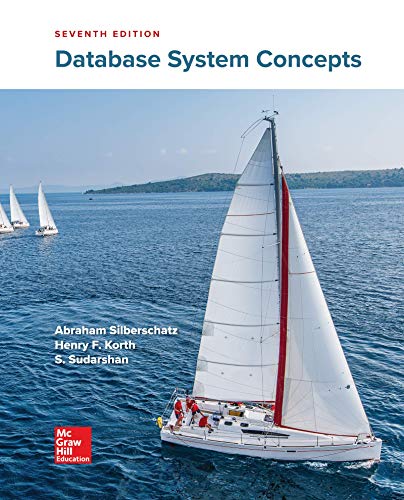
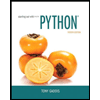
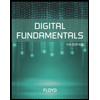
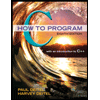
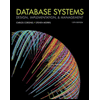
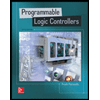