Given the main class Dog, subclass Poodle, and the beginning of the subclass Pug: Instructions for Pug.java Variables: int flabCount – the count of layers on the Pug An invalid value should default this field to 25. A value is invalid if it is nonpositive. boolean isPound – whether this Pug resides in the pound. Poodle bestFriend- a Poodle who is the best friend with the Pug Constructors: A constructor that takes in name, length, weight, stripeCount, isSaltwater, and bestFriend. The bestFriend argument should be deep copied. A constructor that takes in no parameters and sets name to “Pluto”, length to 30.0, weight to 320.0, stripeCount to 14, isPound to false, and bestFriend to null. A copy constructor that deep copies all necessary instance fields. Methods: migrate The pug moves to the opposite kind of living situation if and only if it does not have a best friend. For example, if this pug were now in a pound and it has a best friend, it would not migrate to a house but instead stay in the pound. toString Overrides the corresponding method in its super class, such that a String with the following format is returned without trailing or leading whitespace. “I’m a talking dog named . My length is ft in and my weight is lbs oz. I’m a {pound/house} pug with layers. I have {no best friend/a best friend} named }.” No getters or setters should be written. 1. Would I do the below to set the default for flabCount? if(int flabCount < 0) this.flabcount = 25? 2. Am I creating the migrate method correctly and if so, how would I go about creating the toString method and calling a name from Poodle for it? 3. Is that the correct notation to deep copy bestFriend and is there anything else that needs fixing to match the instructions?
Given the main class Dog, subclass Poodle, and the beginning of the subclass Pug: Instructions for Pug.java Variables: int flabCount – the count of layers on the Pug An invalid value should default this field to 25. A value is invalid if it is nonpositive. boolean isPound – whether this Pug resides in the pound. Poodle bestFriend- a Poodle who is the best friend with the Pug Constructors: A constructor that takes in name, length, weight, stripeCount, isSaltwater, and bestFriend. The bestFriend argument should be deep copied. A constructor that takes in no parameters and sets name to “Pluto”, length to 30.0, weight to 320.0, stripeCount to 14, isPound to false, and bestFriend to null. A copy constructor that deep copies all necessary instance fields. Methods: migrate The pug moves to the opposite kind of living situation if and only if it does not have a best friend. For example, if this pug were now in a pound and it has a best friend, it would not migrate to a house but instead stay in the pound. toString Overrides the corresponding method in its super class, such that a String with the following format is returned without trailing or leading whitespace. “I’m a talking dog named . My length is ft in and my weight is lbs oz. I’m a {pound/house} pug with layers. I have {no best friend/a best friend} named }.” No getters or setters should be written. 1. Would I do the below to set the default for flabCount? if(int flabCount < 0) this.flabcount = 25? 2. Am I creating the migrate method correctly and if so, how would I go about creating the toString method and calling a name from Poodle for it? 3. Is that the correct notation to deep copy bestFriend and is there anything else that needs fixing to match the instructions?
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 2PE
Related questions
Question
Given the main class Dog, subclass Poodle, and the beginning of the subclass Pug:
Instructions for Pug.java
Variables:
- int flabCount – the count of layers on the Pug
- An invalid value should default this field to 25.
- A value is invalid if it is nonpositive.
- boolean isPound – whether this Pug resides in the pound.
- Poodle bestFriend- a Poodle who is the best friend with the Pug
Constructors:
- A constructor that takes in name, length, weight, stripeCount, isSaltwater, and bestFriend.
- The bestFriend argument should be deep copied.
- A constructor that takes in no parameters and sets name to “Pluto”, length to 30.0, weight to 320.0, stripeCount to 14, isPound to false, and bestFriend to null.
- A copy constructor that deep copies all necessary instance fields.
Methods:
- migrate
- The pug moves to the opposite kind of living situation if and only if it does not have a best friend.
- For example, if this pug were now in a pound and it has a best friend, it would not migrate to a house but instead stay in the pound.
- toString
- Overrides the corresponding method in its super class, such that a String with the following format is returned without trailing or leading whitespace.
- “I’m a talking dog named <name>. My length is <feet> ft <inches> in and my weight is <pounds> lbs <ounces> oz. I’m a {pound/house} pug with <flabCount> layers. I have {no best friend/a best friend} named <bestFriendName>}.”
- No getters or setters should be written.
1. Would I do the below to set the default for flabCount?
if(int flabCount < 0)
this.flabcount = 25?
2. Am I creating the migrate method correctly and if so, how would I go about creating the toString method and calling a name from Poodle for it?
3. Is that the correct notation to deep copy bestFriend and is there anything else that needs fixing to match the instructions?
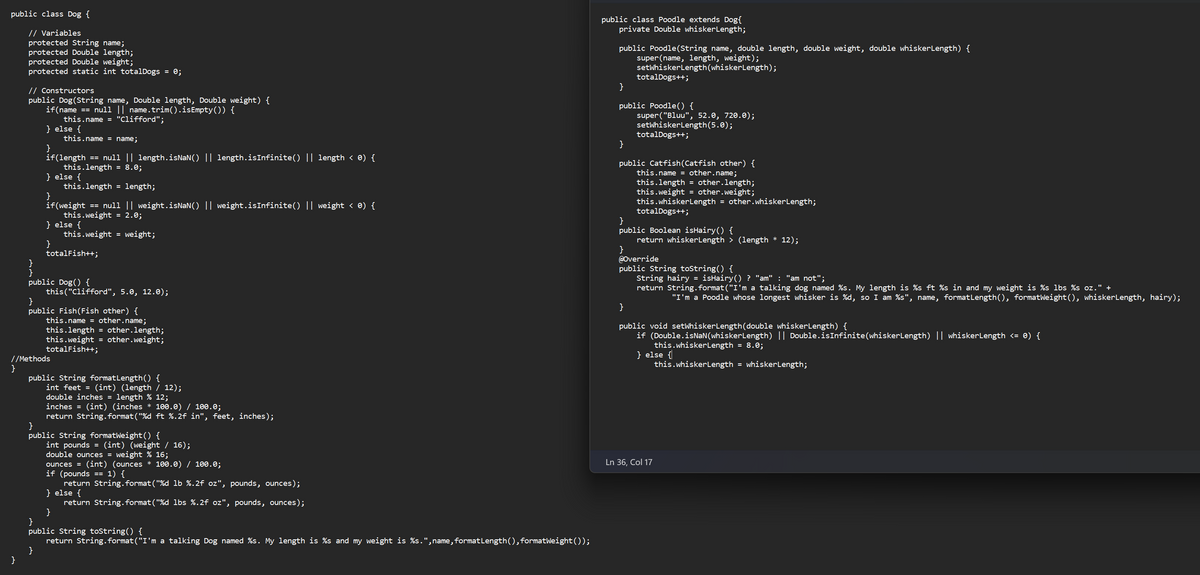
Transcribed Image Text:public class Dog {
// Variables
protected String name;
protected Double length;
protected Double weight;
protected static int totalDogs = 0;
}
// Constructors
public Dog (String name, Double length, Double weight) {
if(name == null || name.trim().isEmpty()) {
this.name = "Clifford";
} else {
this.name = name;
}
if(length == null || length.isNaN() || length.isInfinite() || length < 0) {
this.length = 8.0;
} else {
this.length = length;
if(weight == null || weight.isNaN() || weight.isInfinite() || weight < 0) {
this.weight = 2.0;
} else {
this.weight = weight;
totalFish++;
public Dog() {
//Methods
this("Clifford", 5.0, 12.0);
}
public Fish (Fish other) {
this.name = other.name;
this.length = other.length;
this.weight = other.weight;
totalFish++;
public String format Length() {
int feet = (int) (length / 12);
double inches = length % 12;
inches = (int) (inches * 100.0) / 100.0;
return String.format("%d ft %.2f in", feet, inches);
}
public String formatWeight() {
int pounds = (int) (weight / 16);
double ounces = weight % 16;
ounces = (int) (ounces * 100.0) / 100.0;
if (pounds == 1) {
return String.format("%d lb %.2f oz", pounds, ounces);
} else {
return String.format("%d lbs %.2f oz", pounds, ounces);
}
}
public String toString() {
return String.format("I'm a talking Dog named %s. My length is %s and my weight is %s.",name, format Length(), formatWeight());
public class Poodle extends Dog{
private Double whiskerLength;
public Poodle(String name, double length, double weight, double whiskerLength) {
super (name, length, weight);
setWhiskerLength (whiskerLength);
totalDogs++;
}
public Poodle() {
}
public Catfish (Catfish other) {
this.name = other.name;
this.length = other.length;
this.weight = other.weight;
this.whiskerLength = other.whiskerLength;
totalDogs++;
super("Bluu", 52.0, 720.0);
setWhiskerLength (5.0);
totalDogs++;
}
public Boolean isHairy() {
}
}
return whiskerLength > (length * 12);
@Override
public String toString() {
String hairy = isHairy() ? "am" : "am not";
return String.format("I'm a talking dog named %s. My length is %s ft %s in and my weight is %s lbs %s oz." +
"I'm a Poodle whose longest whisker is %d, so I am %s", name, formatLength(), formatWeight(), whiskerLength, hairy);
public void setWhiskerLength(double whiskerLength) {
if (Double.isNaN(whiskerLength) || Double.isInfinite(whiskerLength) || whiskerLength <= 0) {
} else {
Ln 36, Col 17
this.whiskerLength = 8.0;
this.whiskerLength = whiskerLength;
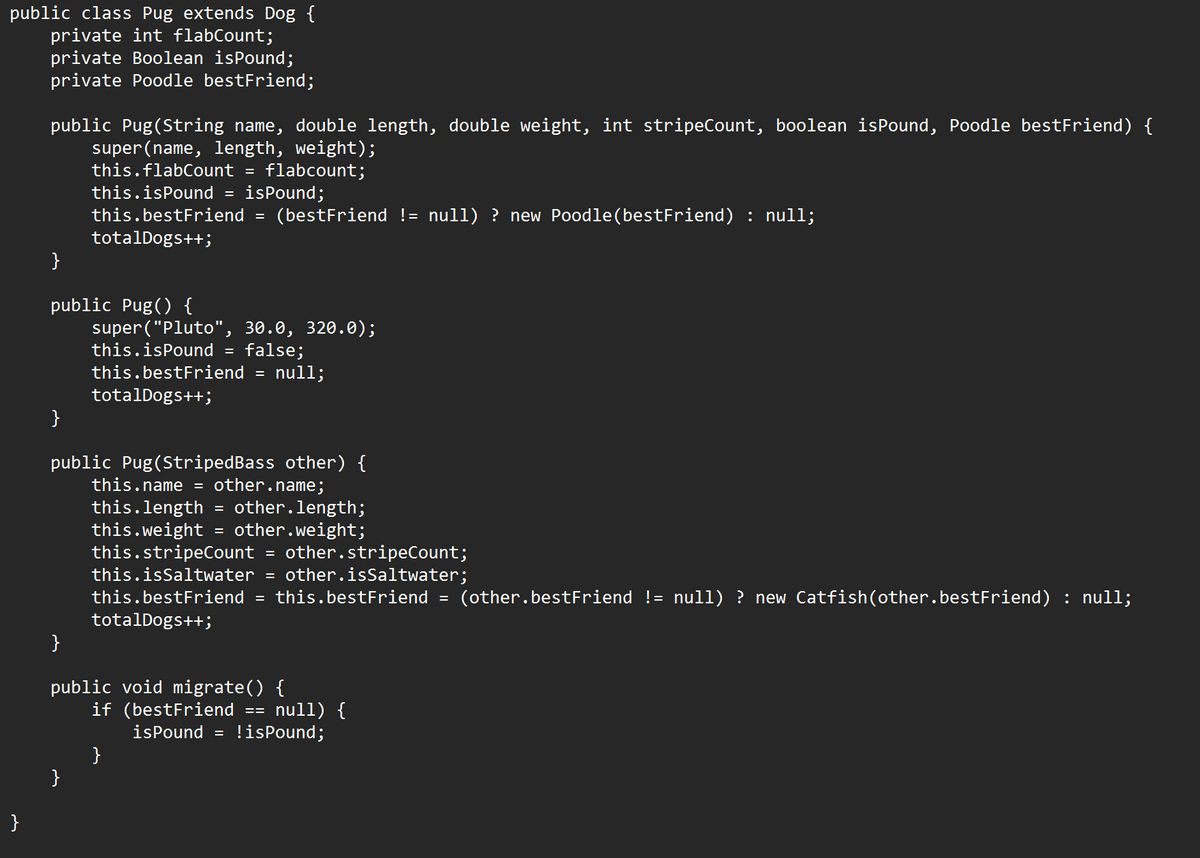
Transcribed Image Text:public class Pug extends Dog {
private int flabCount;
private Boolean isPound;
private Poodle bestFriend;
}
public Pug (String name, double length, double weight, int stripeCount, boolean isPound, Poodle bestFriend) {
super (name, length, weight);
this.flabCount =
flabcount;
this.isPound = isPound;
this.bestFriend (bestFriend != null) ? new Poodle (bestFriend) : null;
totalDogs++;
}
public Pug() {
}
}
super("Pluto", 30.0, 320.0);
this.isPound = false;
this.bestFriend
totalDogs++;
public Pug (StripedBass other) {
this.name = other.name;
this.length = other.length;
this.weight other.weight;
this.stripeCount = other.stripeCount;
this.isSaltwater
= other.isSaltwater;
this.bestFriend = this.bestFriend = (other.bestFriend != null) ? new Catfish(other.bestFriend) : null;
totalDogs++;
}
=
=
null;
public void migrate() {
==
if (bestFriend null) {
isPound = !isPound;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
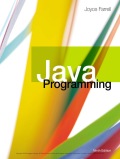
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
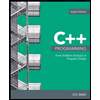
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
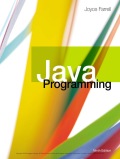
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
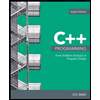
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
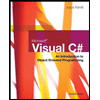
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,