TOPIC: Inheritance In Python, modify the given code based on the given specifications below. Create another class, “Food.” For the Food class, the specifications are: The object will have the following attributes on initialization: weight (weight of the food in kg) energy_add (amount of energy it will add once consumed) happiness_add (amount of happiness it will add once consumed) Modify the Child and the Friends classes such that: Instead of accepting a string (as food) in the eat method, the method should accept a Foodobject Once a Child/Friendsobject eats a Food object: The added weight should be the Food object’s weight The added energy should be the Food object’s energy_add The added happiness should be the Food object’s happiness_add After modifying the Child and Friends behavior, simulate a small group of Friends. In the main code, do the following: Accept a positive integer from the user that determines the number of friends and create a number of Friends specified in the input. For each friend: The child_number should be from 1 to children_count (inclusive) and each Friend should have a unique child_number name should be “ChildX” where X is the child number weight should be 50 + 0.5 * (child number - 1) in kg The code should look like (can be found on the first picture) The given code (can also be found on the picture):
TOPIC: Inheritance In Python, modify the given code based on the given specifications below. Create another class, “Food.” For the Food class, the specifications are: The object will have the following attributes on initialization: weight (weight of the food in kg) energy_add (amount of energy it will add once consumed) happiness_add (amount of happiness it will add once consumed) Modify the Child and the Friends classes such that: Instead of accepting a string (as food) in the eat method, the method should accept a Foodobject Once a Child/Friendsobject eats a Food object: The added weight should be the Food object’s weight The added energy should be the Food object’s energy_add The added happiness should be the Food object’s happiness_add After modifying the Child and Friends behavior, simulate a small group of Friends. In the main code, do the following: Accept a positive integer from the user that determines the number of friends and create a number of Friends specified in the input. For each friend: The child_number should be from 1 to children_count (inclusive) and each Friend should have a unique child_number name should be “ChildX” where X is the child number weight should be 50 + 0.5 * (child number - 1) in kg The code should look like (can be found on the first picture) The given code (can also be found on the picture):
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
TOPIC: Inheritance
In Python, modify the given code based on the given specifications below. Create another class, “Food.”
For the Food class, the specifications are:
- The object will have the following attributes on initialization:
- weight (weight of the food in kg)
- energy_add (amount of energy it will add once consumed)
- happiness_add (amount of happiness it will add once consumed)
Modify the Child and the Friends classes such that:
- Instead of accepting a string (as food) in the eat method, the method should accept a Foodobject
- Once a Child/Friendsobject eats a Food object:
- The added weight should be the Food object’s weight
- The added energy should be the Food object’s energy_add
- The added happiness should be the Food object’s happiness_add
After modifying the Child and Friends behavior, simulate a small group of Friends. In the main code, do the following:
- Accept a positive integer from the user that determines the number of friends and create a number of Friends specified in the input.
- For each friend:
- The child_number should be from 1 to children_count (inclusive) and each Friend should have a unique child_number
- name should be “ChildX” where X is the child number
- weight should be 50 + 0.5 * (child number - 1) in kg
- The code should look like (can be found on the first picture)
The given code (can also be found on the picture):
class Child:
def __init__(self, child_number: int, name: str, weight: float):
self.child_number = child_number
self.name = name
self.weight = weight
def eat(self, food: str):
self.weight = self.weight + 0.50
def exercise(self, exercise: str):
self.weight = self.weight - 0.50
def weight_in_grams(self):
return self.weight * 1000
class Friends(Child):
energy = 50
happiness = 50
def eat(self, food: str):
self.energy = self.energy + 10
self.happiness = self.happiness + 10
return Child.eat(self, food) and self.energy and self.happiness
def exercise(self, food: str):
self.energy = self.energy - 10
self.happiness = self.happiness + 10
return Child.exercise(self, food) and self.energy and self.happiness
![In Python, modify the given code based on the given specifications below. Create another class, "Food."
A. For the Food class, the specifications are:
The object will have the following attributes on initialization:
weight (weight of the food in kg)
energy_add (amount of energy it will add once consumed)
happiness_add (amount of happiness it will add once consumed)
B. Modify the Child and the Friends classes such that:
Instead of accepting a string (as food) in the eat method, the method should accept a Food object
Once a Child/Friends object eats a Food object:
The added weight should be the Food object's weight
The added energy should be the Food object's energy_add
The added happiness should be the Food object's happiness_add
C. After modifying the Child and Friends behavior, simulate a small group of Friends. In the main code, do
the following:
Accept a positive integer from the user that determines the number of friends and create a.
number of Friends specified in the input.
For each friend:
The child_number should be from 1 to children_count (inclusive) and each Friend should have
a unique child_number
name should be “ChildX" where X is the child number
weight should be 50 + 0.5 * (child number -1) in kg
The code should look like:
children_count = int(input("Input number of friends: ")
children = []
for i in range(children_count):
## create new_friend here ##
children.append(new_friend)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa3cf655d-3bdc-4013-8c1f-5d6d56ea7228%2F163e2337-40db-4991-80b4-47798ea9605e%2Fa3hcc8r_processed.png&w=3840&q=75)
Transcribed Image Text:In Python, modify the given code based on the given specifications below. Create another class, "Food."
A. For the Food class, the specifications are:
The object will have the following attributes on initialization:
weight (weight of the food in kg)
energy_add (amount of energy it will add once consumed)
happiness_add (amount of happiness it will add once consumed)
B. Modify the Child and the Friends classes such that:
Instead of accepting a string (as food) in the eat method, the method should accept a Food object
Once a Child/Friends object eats a Food object:
The added weight should be the Food object's weight
The added energy should be the Food object's energy_add
The added happiness should be the Food object's happiness_add
C. After modifying the Child and Friends behavior, simulate a small group of Friends. In the main code, do
the following:
Accept a positive integer from the user that determines the number of friends and create a.
number of Friends specified in the input.
For each friend:
The child_number should be from 1 to children_count (inclusive) and each Friend should have
a unique child_number
name should be “ChildX" where X is the child number
weight should be 50 + 0.5 * (child number -1) in kg
The code should look like:
children_count = int(input("Input number of friends: ")
children = []
for i in range(children_count):
## create new_friend here ##
children.append(new_friend)
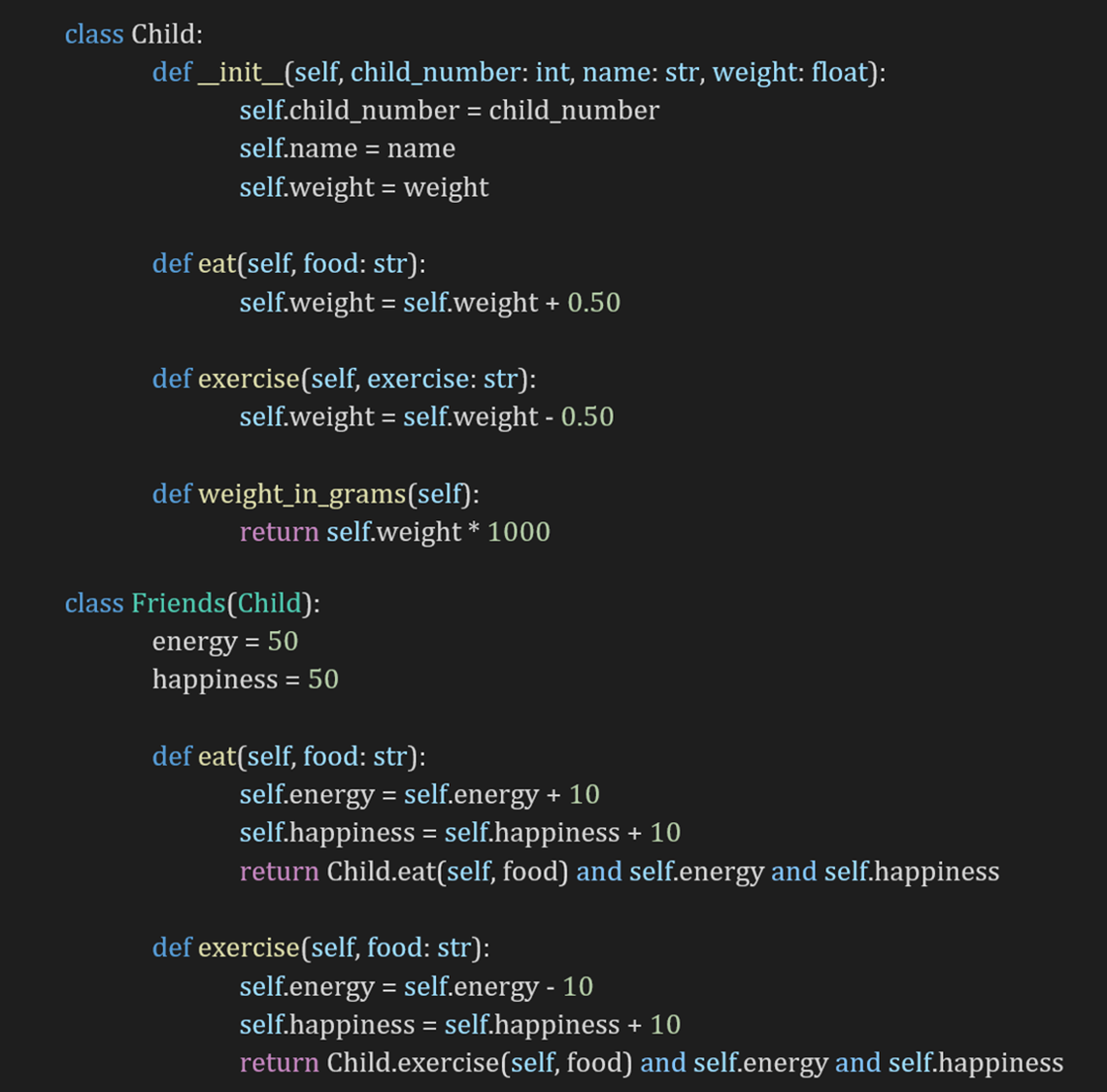
Transcribed Image Text:class Child:
def _init_(self, child_number: int, name: str, weight: float):
self.child_number = child_number
%3D
self.name = name
self.weight = weight
def eat(self, food: str):
self.weight = self.weight + 0.50
def exercise(self, exercise: str):
self.weight = self.weight - 0.50
def weight_in_grams(self):
return self.weight * 1000
class Friends(Child):
energy = 50
happiness = 50
%3D
def eat(self, food: str):
self.energy = self.energy + 10
self.happiness = self.happiness + 10
return Child.eat(self, food) and self.energy and self.happiness
%3D
def exercise(self, food: str):
self.energy = self.energy - 10
self.happiness = self.happiness + 10
return Child.exercise(self, food) and self.energy and self.happiness
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
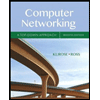
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
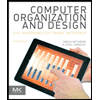
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
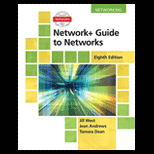
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
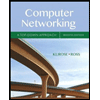
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
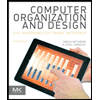
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
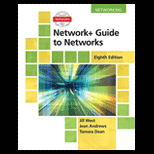
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
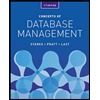
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
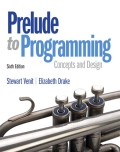
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
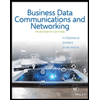
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY