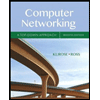
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
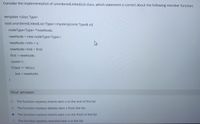
Transcribed Image Text:Consider the implementation of unorderedLinkedList class, which statement is correct about the following member function:
template <class Type>
void unorderedLinkedList<Type>::mystery(const Type& x){
nodeType<Type> *newNode;
newNode = new nodeType<Type>3;
newNode->info = x;
newNode->link = first;
first = newNode;
count++;
if (last == NULL)
last = newNode;
Your answer:
O The function mystery inserts item x to the end of the list
The function mystery deletes item x from the list.
The function mystery inserts item x to the front of the list
The function mystery searches item x in the list
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- template <class T> class List; template <class T> class Node{ friend class List<T>; private: T data; Node* link; }; template <class T> class List{ public: List(){first = 0;} void InsertBack(const T& e); void Concatenate(List<T>& b); void Reverse(); class Iterator{ …. }; Iterator Begin(); Iterator End(); private: Node* first; }; I need algorithm , I think it may use iterator to complile. The question shows on below photo.arrow_forward1. Follow the class instruction and complete the Cat class. Create class Cat a. Add properties: i. What characteristics do they have? Name, age, color, type (domestic / feral), etc. (secure the data) ii. What color(s) might they have? White, cream, fawn, Cinnamon, Chocolate, Red, Lilac, Blue, Black, Lavender, etc. b. Add methods: eat()), play(), etc. c. Constructors? i. A class provides a special type of methods, known as constructors, which are invoked to construct objects from the class ii. Create 2 different constructor with different initialization parameters d. How to get the number of cats? Add a static variable and a static method to print out the number of cats. e. Test Cat class by creating 3 Cat instances: i. Each cat should have different properties. ii. Test all the methods. iii. Print out the number of cats. 2. Define a BankAccount class that has: accNum (int) Balance (double) ● a constructor four methods: o deposit() withdraw() O o getAccNum() o getBalance() Implement a…arrow_forwardCould you help me the following question? The Node structure is defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; public Node previous; } The Double Ended Doubly Linked List (DEDLL) class definition is as follows: public class DEDLL { public int currentSize; public Node head; public Node tail; } This design is a modification of the standard linked list. In the standard that we discussed in class, there is only a head node that represents the first node in the list and each node only contains a reference to the next node in the list until the chain of nodes reach null. In this DEDLL, each node also has a reference to the previous node. The DEDLL also contains a reference to the tail of the list that represents the last node in the list. For example, a DEDLL with 4 elements may have nodes with values 5, 2, 6, and 8 from head to tail. This…arrow_forward
- 3. Define a Pair class using Java Generics framework a. Properties: first, second b. Constructors: Create 2 different constructor with different initialization parameters c. Methods: setFirst(), setSecond(), getFirst(), getSecond(); d. Test Pair class print out the value of Pair instance: i. Define a Pair instance and assign (10,10.1) to the pair; ii. Define a Pair instance and assign (8.2, "ABC") to the pair; iii. Define an array holding[] of Pair class. 1. holding has 100 elements; 2. Assign (int, double) to array by using loop -> (0, 100.0), (1, 99.0), (2, 98.0), ..., (99, 1.0).arrow_forwardCreate a ToArray function for the LinkedList class that returns an array from a linked list instance.arrow_forwardIn this chapter, the class to implement the nodes of a linked list is defined as a struct. The following rewrites the definition of the struct nodeType so that it is declared as a class and the member variables are private. template <class Type>class nodeType{public:const nodeType<Type>& operator=(const nodeType<Type>&);//Overload the assignment operator.void setInfo(const Type& elem);//Function to set the info of the node.//Postcondition: info = elem;Type getInfo() const;//Function to return the info of the node.//Postcondition: The value of info is returned.void setLink(nodeType<Type> *ptr);//Function to set the link of the node.//Postcondition: link = ptr;nodeType<Type>* getLink() const;//Function to return the link of the node.//Postcondition: The value of link is returned.nodeType();//Default constructor//Postcondition: link = NULL;nodeType(const Type& elem, nodeType<Type> *ptr);//Constructor with parameters//Sets info point to…arrow_forward
- Assume that, you have a Linked List class "LL" with a function named "fun1". You can assume that "value" is the value of the nodes and "Next" is the next pointer of the node. You created an instance of the LL class, named "L1", where "head" is the head node. And you inserted the following numbers: 31 -> 32 -> 43 -> 17 -> 34 -> 57 ->91. What will be the output of the linked list L1 after the "fun1" operation to it?* 31 -> 32 -> 43 -> 17 -> 34 -> 57 ->91 31 -> 32 -> 17 -> 34 -> 57 ->91 31 -> 32 -> 43 -> 17 -> 57 ->91 31 -> 32 -> 43 -> 17 -> 34 -> 57 31 -> 32 -> 43 -> 57 ->91 31 -> 32 -> 43 -> 34 -> 57 ->91arrow_forward1. Write code to define LinkedQueue<T> and CircularArrayQueue<T> classes which willimplement QueueADT<T> interface. Note that LinkedQueue<T> and CircularArrayQueue<T>will use linked structure and circular array structure, respectively, to define the followingmethods.(i) public void enqueue(T element); //Add an element at the rear of a queue(ii) public T dequeue(); //Remove and return the front element of the queue(iii) public T first(); //return the front element of the queue without removing it(iv) public boolean isEmpty(); //Return True if the queue is empty(v) public int size(); //Return the number of elements in the queue(vi) public String toString();//Print all the elements of the queuea. Create an object of LinkedQueue<String> class and then add some names of your friendsin the queue and perform some dequeue operations. At the end, check the queue sizeand print the entire queue.b. Create an object from CircularArrayQueue<T> class and…arrow_forwardCreate a ( operator overlod = ) for a doubly linked list, in C++. for this class #include<iostream>using namespace std; class Node{ public: int data; Node *next, *prev; Node(int val){ data = val; next = NULL; prev = NULL; }}; class DLL{ private: Node *root; public: //default constructor DLL(){ root = NULL; } //copy constructor DLL(const DLL& list){ Node *newNode, *tail; Node *current = list.root; while(current != NULL){ newNode = new Node(current->data); if(root == NULL) { root = newNode; tail = root; } else{ tail->next = newNode; newNode->prev = tail; tail = newNode; } current =…arrow_forward
- Given the tollowiıng class template detinition: template class linkedListType { public: const linked ListType& operator=(const linked ListType&); I/Overload the assignment operator. void initializeList(); /Initialize the list to an empty state. //Postcondition: first = nullptr, last = nullptr, count = 0; linked ListType(); Ildefault constructor //Initializes the list to an empty state. /Postcondition: first = nullptr, last = nullptr, count = 0; -linkedListīype(); Ildestructor /Deletes all the nodes from the list. //Postcondition: The list object is destroyed. protected: int count; //variable to store the number of elements in the list nodeType *first; //pointer to the first node of the list nodeType *last; //pointer to the last node of the list 1) Write initializeList() 2) Write linkedListType() 3) Write operator=0 4) Add the following function along with its definition: void rotate(); I/ Remove the first node of a linked list and put it at the end of the linked listarrow_forwardTwo stacks of the same type are the same if they have the same number of elements and their elements at the corresponding positions are the same. Overload the relational operator == for the class stackType that returns true if two stacks of the same type are the same; it returns false otherwise. Also, write the definition of the function template to overload this operator.arrow_forwardDesign an implementation of an Abstract Data Type consisting of a set with the following operations: insert(S, x) Insert x into the set S. delete(S, x) Delete x fromthe set S. member(S, x) Return true if x ∈ S, false otherwise. position(S, x) Return the number of elements of S less than x. concatenate(S, T) Set S to the union of S and T, assuming every element in S is smaller than every element of T. All operations involving n-element sets are to take time O(log n).arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
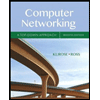
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
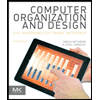
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
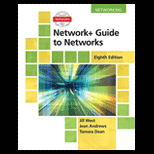
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
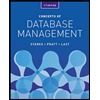
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
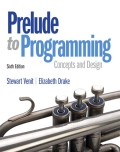
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
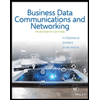
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY